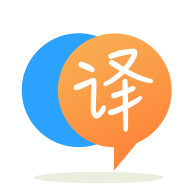
[英]How can I return values in a recursive function without stopping the recursion?
[英]How can I return values from a recursive function?
我的代码可以对值列表进行快速排序:
def Quick_sort_random(random_list):
r = random_list.copy()
def inner(r):
if len(r) < 2:
return r
p = random.randint(0, len(r) - 1)
pivot = r[p]
left = list(filter(lambda x: x <= pivot, r[:p] + r[p + 1:]))
right = list(filter(lambda x: x > pivot, r[:p] + r[p + 1:]))
return Quick_sort_random(left) + [pivot] + Quick_sort_random(right)
a = inner(r)
return random_list,a
这会引发错误:
TypeError: can only concatenate tuple (not "list") to tuple
我有 inner() function 因为我想返回原始列表和排序列表。 我怎样才能解决这个问题?
您的Quick_sort_random
function 返回一个元组,但您只需要其中的第二项进行排序。 将inner
中的最后一行更改为:
return Quick_sort_random(left)[1] + [pivot] + Quick_sort_random(right)[1]
只需返回inner
function 中的排序列表:
import random
def Quick_sort_random(random_list):
r = random_list.copy()
def inner(r):
if len(r) < 2:
return r
p = random.randint(0, len(r) - 1)
pivot = r[p]
left = list(filter(lambda x: x <= pivot, r[:p] + r[p + 1:]))
right = list(filter(lambda x: x > pivot, r[:p] + r[p + 1:]))
unsorted_left, sorted_left = Quick_sort_random(left)
unsorted_right, sorted_right = Quick_sort_random(right)
return sorted_left + [pivot] + sorted_right
a = inner(r)
return random_list,a
print(Quick_sort_random([4,5,1,5,2,3]))
Output:
([4, 5, 1, 5, 2, 3], [1, 2, 3, 4, 5, 5])
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.