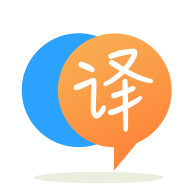
[英]python: how to check if string has the same characters / the probability of repeating them is the same
[英]How to check if the characters in the corresponding positions in the second string are repeating in same position like in first string? Educative EXAM
它来自 Educative.io 第一次考试“从头开始学习 Python 3”:
检测字符串模式
在这个编码练习中,你被要求编写一个名为detect_pattern 的function 的主体,它根据两个字符串是否具有相同的字符模式返回真或假。 更准确地说,如果两个字符串具有相同的长度并且如果第一个字符串中的两个字符相等,当且仅当第二个字符串中相应位置的字符也相等时,它们具有相同的模式。
以下是一些相同模式和不同模式的示例:
第一个字符串 | 第二串 | 相同的模式 |
---|---|---|
“” | “” | 真的 |
“一个” | “一个” | 真的 |
“X” | “是” | 真的 |
“ab” | “xy” | 真的 |
“阿巴” | “xyz” | 错误的 |
“————” | “xyz” | 错误的 |
“————” | “啊” | 真的 |
“xyzxyz” | “脚趾” | 真的 |
“xyzxyz” | “脚趾甲” | 错误的 |
“aaabbbcccd” | “eeeffgggz” | 真的 |
“cbacbacba” | “xyzxyzxyz” | 真的 |
“abcdefghijk” | “lmnopqrstuv” | 真的 |
“阿萨萨萨斯” | “xxxxxyyyyy” | 错误的 |
“ascneencsa” | “艾欧艾欧欧” | 错误的 |
“aaasssiiii” | “ggdddfffh” | 错误的 |
例如,如果名为 s1 和 s2 的两个字符串包含以下字母:
s1 = "阿巴"
s2 = "xyz"
那么调用 detect_pattern(s1, s2) 应该返回 False。
注意:function detect_pattern 有两个参数:要比较的两个字符串。 你可以创建新的字符串,但是你不能构造额外的数据结构来解决这个问题(没有列表、集合、字典等)。 请记住,无论传递两个字符串的顺序如何,该方法都应该返回相同的值。
祝你好运!
我的代码:
import unittest
import re # only needed if we use hint from 6th line
''' HINT:
if we add this to the end of 13th line before ending ":":
and pattern.match(s1.replace(" ", "")) == pattern.match(s2.replace(" ", "")) and len(set(s1.replace(" ", ""))) == len(set(s2.replace(" ", "")))
it will solve more case's but we can't use set() method.
'''
pattern = re.compile("^([a-z][0-9]+)+$") # only needed if we use hint from 6th line
def detect_pattern(s1, s2):
if len(s1.replace(" ", "")) == len(s2.replace(" ", "")):
return True
else:
return False
class TestDetectPattern(unittest.TestCase):
def test_basics(self):
self.assertEqual(detect_pattern("", ""), True)
self.assertEqual(detect_pattern("a", "a"), True)
self.assertEqual(detect_pattern("x", "y"), True)
self.assertEqual(detect_pattern("ab", "xy"), True)
# self.assertEqual(detect_pattern("aba", "xyz"), False) # can be solved with hint from 6th line but in this task we can't use set() method
# self.assertEqual(detect_pattern("- - -", "xyz"), False) # can be solved with hint from 6th line but in this task we can't use set() method
self.assertEqual(detect_pattern("- - -", "aaa"), True)
self.assertEqual(detect_pattern("xyzxyz", "toetoe"), True)
# self.assertEqual(detect_pattern("xyzxyz", "toetoa"), False) # can be solved with hint from 6th line but in this task we can't use set() method
self.assertEqual(detect_pattern("aaabbbcccd", "eeefffgggz"), True)
self.assertEqual(detect_pattern("cbacbacba", "xyzxyzxyz"), True)
self.assertEqual(detect_pattern("abcdefghijk", "lmnopqrstuv"), True)
# self.assertEqual(detect_pattern("asasasasas", "xxxxxyyyyy"), False)
# self.assertEqual(detect_pattern("ascneencsa", "aeiouaeiou"), False)
# self.assertEqual(detect_pattern("aaasssiiii", "gggdddfffh"), False) # can be solved with hint from 6th line but in this task we can't use set() method
if __name__ == '__main__':
unittest.main()
有谁知道如何检查第二个字符串中相应位置的字符是否像第一个字符串一样在相同的 position 中重复? - 我认为这可以在不使用 set() 方法的情况下解决整个问题。
编辑:在 if 语句的条件下存在一些问题,但现在应该解决这个问题。
这种方法应该有效。 我对循环中的变量命名不是很满意,所以请随意更改它们。 我用你提供的所有例子都试过了,所以在语义上它应该是正确的。
def detect_pattern(s1: str, s2: str) -> bool:
if len(s1) != len(s2):
return False
for idx, (let_1, let_2) in enumerate(zip(s1, s2)):
for let_1_inner, let_2_inner in zip(s1[idx:], s2[idx:]):
if (let_1_inner == let_1) != (let_2_inner == let_2):
return False
return True
目前我正在 education.io 上这门课程。 起初,当我阅读这个问题时,我不明白如何准确地执行这个程序。
在@benjamin 的上述解决方案中,他使用了 zip() 和 enumerate() 等函数。 这两个函数和数据结构在本课程中还没有讲授。 因此,上述解决方案不建议使用 go。
我写了一个没有这两个功能的程序,它成功了。
def detect_pattern(s1, s2):
if len(s1) != len(s2):
return False
for i in range (len (s1)):
let_1 = s1 [i]
let_2 = s2 [i]
for j in range ( i, len (s1)):
let_1_inner = s1 [j]
let_2_inner = s2 [j]
if (let_1_inner == let_1) != (let_2_inner == let_2):
return False
return True
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.