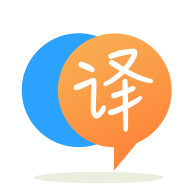
[英]How to change certain elements in a 2D numpy array at specified col positions?
[英]How to change the font color of the specified elements in Numpy
我想知道如何更改指定元素的字体颜色以在 numpy 数组中标记它们。 我引用了这个问题的答案: Coloring entries in an Matrix/2D-numpy array? 我编写了以下两个函数来做到这一点。 例如,在下面的例子中,我想改变a[0,1], a[1,2], a[2,0]
的颜色。 在trans(a, j, *lst)
函数中, a
是输入矩阵, j
是颜色编号, *lst
是要修改的元素的索引。
import numpy as np
a = np.array([[0,0,0],
[0,0,0],
[23.7135132,0,0],
[32.22954112,0,0],
[71.01941585,31.27384681,0]])
print(a,'\n')
def trans(a,j,*lst):
num_row = a.shape[0]
num_column = a.shape[1]
add = ''
row = []
column = []
# record the row index and column index of each input (in *lst)
for k in range(len(lst)):
row.append(lst[k][0])
column.append(lst[k][1])
# Generate the same content as 'a' and change the color
for m in range(num_row):
for n in range(num_column):
for k in range(len(lst)):
# the element whose color needs to be modified
if m == row[k] and n == column[k]:
add += "\033[3{}m{}\033[0m ".format(j, a[row[k],column[k]])
break
# the element whose color does not need to be modified
if k == len(lst)-1:
add += "\033[3{}m{}\033[0m ".format(0, a[m,n])
# There is a space at the end of "\033[3{}m{}\033[0m "
add = add.strip(' ')
add = add.split(' ')
add = np.array(add)
add = add.reshape(num_row,num_column)
return add
def show(a, row_sep=" "):
n, m = a.shape
fmt_str = "\n".join([row_sep.join(["{}"]*m)]*n)
return fmt_str.format(*a.ravel())
print(show(trans(a,1,[0,1],[1,2],[2,0])))
但结果如下。
可以看到,元素是无法对齐的,所以我想知道如何自动对齐这些元素,就像numpy数组的显示一样。 或者有没有其他方法可以直接修改numpy数组的字体颜色? 我只是想让一些特殊的元素更加显眼。
这是一个非常通用的版本,它包含np.array2string()
。 它适用于任何 dtype 和/或自定义格式化程序,并且输出看起来应该与np.array2string()
相同。 它没有尝试解析格式化的字符串,而是使用自己的自定义格式化程序在目标元素周围添加转义序列。 它还使用str
子类,因此换行的长度计算忽略了转义序列。
import re
import numpy as np
class EscapeString(str):
"""A string that excludes SGR escape sequences from its length."""
def __len__(self):
return len(re.sub(r"\033\[[\d:;]*m", "", self))
def __add__(self, other):
return EscapeString(super().__add__(other))
def __radd__(self, other):
return EscapeString(str.__add__(other, self))
def color_array(arr, color, *lst, **kwargs):
"""Takes the same keyword arguments as np.array2string()."""
# adjust some kwarg name differences from _make_options_dict()
names = {"max_line_width": "linewidth", "suppress_small": "suppress"}
options_kwargs = {names.get(k, k): v for k, v in kwargs.items() if v is not None}
# this comes from arrayprint.array2string()
overrides = np.core.arrayprint._make_options_dict(**options_kwargs)
options = np.get_printoptions()
options.update(overrides)
# from arrayprint._array2string()
format_function = np.core.arrayprint._get_format_function(arr, **options)
# convert input index lists to tuples
target_indices = set(map(tuple, lst))
def color_formatter(i):
# convert flat index to coordinates
idx = np.unravel_index(i, arr.shape)
s = format_function(arr[idx])
if idx in target_indices:
return EscapeString(f"\033[{30+color}m{s}\033[0m")
return s
# array of indices into the flat array
indices = np.arange(arr.size).reshape(arr.shape)
kwargs["formatter"] = {"int": color_formatter}
return np.array2string(indices, **kwargs)
例子:
np.set_printoptions(threshold=99, linewidth=50)
a = np.arange(100).reshape(5, 20)
print(a)
print(np.array2string(a, threshold=a.size))
print()
print(color_array(a, 1, [0, 1], [1, 3], [0, 12], [4, 17]))
print(color_array(a, 1, [0, 1], [1, 3], [0, 12], [4, 17], threshold=a.size))
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.