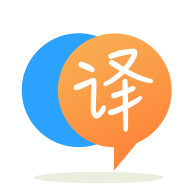
[英]How do I create a segmented cumulative sum graph in Palantir Foundry Workshop?
[英]How do I create a cumulative sum graph in Palantir Foundry Workshop?
您可以使用 Foundry Function 来执行此操作。 创建一个 TypeScript 函数存储库并使用以下代码(有关说明,请参阅内联注释):
import { Function, Double, ThreeDimensionalAggregation, IRange, IRangeable, Timestamp, BucketKey, BucketValue } from "@foundry/functions-api";
// Replace MaintenanceJob with your object
// To make your object available, add it in the Settings > Ontology tab
import { ObjectSet, MaintenanceJob } from "@foundry/ontology-api";
export class MyFunctions {
// You will find this function in Workshop after it's published
// Replace MaintenanceJob with your object
@Function()
public async cumulativeJobsByMonth(jobs: ObjectSet<MaintenanceJob>): Promise<TwoDimensionalAggregation<IRange<Timestamp>, Double>> {
const bucketedJobs = await jobs
.groupBy(j => j.dueOn.byMonth())
.count();
const sortedBucketedJobs = sortBuckets(bucketedJobs);
const cumulativeSortedBucketedJobs = cumulativeSum2D(sortedBucketedJobs);
return cumulativeSortedBucketedJobs
}
}
/**
* Sort buckets of a 2D or 3D aggregation by the first axis in ascending order
*
* Example input 1:
* { buckets: [
* { key: { min: "2022-01-01", max: "2022-12-31" }, value: 456 },
* { key: { min: "2021-01-01", max: "2021-12-31" }, value: 123 },
* { key: { min: "2023-01-01", max: "2023-12-31" }, value: 789 },
* ]}
*
* Example output 1:
* { buckets: [
* { key: { min: "2021-01-01", max: "2021-12-31" }, value: 123 },
* { key: { min: "2022-01-01", max: "2022-12-31" }, value: 456 },
* { key: { min: "2023-01-01", max: "2023-12-31" }, value: 789 },
* ]}
*
* Example input 2:
* { buckets: [
* { key: 22, value: 456 },
* { key: 21, value: 123 },
* { key: 23, value: 789 },
* ]}
*
* Example output 2:
* { buckets: [
* { key: 21, value: 123 },
* { key: 22, value: 456 },
* { key: 23, value: 789 },
* ]}
*
* Example input 3:
* { buckets: [
* { key: { min: "2022-01-01", max: "2022-12-31" }, value: [{ key: "open", value: 789 }, { key: "closed", value: 910 }] },
* { key: { min: "2021-01-01", max: "2021-12-31" }, value: [{ key: "open", value: 123 }, { key: "closed", value: 456 }] },
* { key: { min: "2023-01-01", max: "2023-12-31" }, value: [{ key: "open", value: 314 }, { key: "closed", value: 42 }] },
* ]}
*
* Example output 3:
* { buckets: [
* { key: { min: "2021-01-01", max: "2021-12-31" }, value: [{ key: "open", value: 123 }, { key: "closed", value: 456 }] },
* { key: { min: "2022-01-01", max: "2022-12-31" }, value: [{ key: "open", value: 789 }, { key: "closed", value: 910 }] },
* { key: { min: "2023-01-01", max: "2023-12-31" }, value: [{ key: "open", value: 314 }, { key: "closed", value: 42 }] },
* ]}
*/
function sortBuckets<K1 extends BucketKey, K2 extends BucketKey, V extends BucketValue>(buckets: ThreeDimensionalAggregation<K1, K2, V>): ThreeDimensionalAggregation<K1, K2, V>;
function sortBuckets<K extends BucketKey, V extends BucketValue>(buckets: TwoDimensionalAggregation<K, V>): TwoDimensionalAggregation<K, V>;
function sortBuckets<K extends BucketKey, V>(buckets: { buckets: { key: K, value: V}[] }): { buckets: { key: K, value: V}[] } {
return {
// See https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort
buckets: buckets.buckets.sort(({ key: k1 }, { key: k2 }) => {
if (typeof k1 !== typeof k2) throw new Error("Inconsistent bucket key types")
// If not objects, these must be either numbers or booleans which can be compared like this
if (typeof k1 !== "object" || typeof k2 !== "object") return Number(k1) - Number(k2);
// If a bucket doesn't have a minimum, it suggests that it is the global unbounded minimum bucket, so must be lower
if (!(k1 as IRange<IRangeable>).min) return -1;
if (!(k2 as IRange<IRangeable>).min) return 1;
// Otherwise, compare both buckets' minimums
return (k1 as IRange<IRangeable>).min!.valueOf() - (k2 as IRange<IRangeable>).min!.valueOf();
}),
};
}
/**
* Calculates a cumulative sum for a TwoDimensionalAggregation over numbers, along the first axis
* The order of the buckets into the function matters for how the values are aggregated
*
* Example input 1:
* { buckets: [
* { key: { min: "2021-01-01", max: "2021-12-31" }, value: 123 },
* { key: { min: "2022-01-01", max: "2022-12-31" }, value: 789 },
* { key: { min: "2023-01-01", max: "2023-12-31" }, value: 314 },
* ]}
*
* Example output 1:
* { buckets: [
* { key: { min: "2021-01-01", max: "2021-12-31" }, value: 123 },
* { key: { min: "2022-01-01", max: "2022-12-31" }, value: 912 },
* { key: { min: "2023-01-01", max: "2023-12-31" }, value: 1226 },
* ]}
*
* Example input 2:
* { buckets: [
* { key: 23, value: 314 },
* { key: 22, value: 789 },
* { key: 21, value: 123 },
* ]}
*
* Example output 2:
* { buckets: [
* { key: 23, value: 314 },
* { key: 22, value: 1103 },
* { key: 21, value: 1226 },
* ]}
*/
const cumulativeSum2D = <K extends BucketKey>(buckets: TwoDimensionalAggregation<K, number>): TwoDimensionalAggregation<K, number> => {
// This holds the running total
let cumulativeValue = 0;
return {
buckets: buckets.buckets.map(b => ({
key: b.key,
// This line adds the current value to the running total, and uses the result of that
value: cumulativeValue += b.value,
}))
}
}
提交您的更改并发布函数版本。 Palantir Foundry 文档中有关于如何创建存储库和发布函数的分步指南。
在您的创意工坊中,您可以创建一个“图表:XY”小部件。 作为数据源,选择您创建的函数并传入相关的对象集。 还有关于在 Workshop 中使用派生聚合的 Palantir Foundry 文档。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.