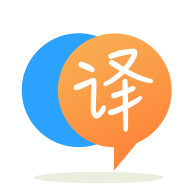
[英]Why “resolve” method always calls the class constructor that is already registered in Unity?
[英]Proper method calls from a common class in Unity
我正在尝试编写漂亮且“正确”的代码,重点是我有一个 Timer 类,它有两个方法“开始游戏的时间”和“结束游戏的时间”,这两个方法都是公开的。 我还有一个场景控制器类,我在其中考虑计时器值并将它们输出到 UI。 我有一个难题,我在哪里调用计时器方法? 在更新控制器或更新计时器中? 这听起来当然很傻,但我想了解如何做得更好。 Timer脚本是一个通用脚本,即不应该绑定到任何场景或UI界面上。
定时器:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Timer : MonoBehaviour
{
public float waitForStartGame = 5;
public float waitForEndGame = 300;
private void Update()
{
WaitForEndGame();
WaitForStartGame();
}
public void WaitForEndGame()
{
if (waitForEndGame >= 0)
{
waitForEndGame -= Time.deltaTime;
}
}
public void WaitForStartGame()
{
if (waitForStartGame >= 0)
{
waitForStartGame -= Time.deltaTime;
}
}
}
控制器:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SumoController : MonoBehaviour
{
public bool isWin;
public bool isGameOver;
public bool isGameStart;
public SumoTankController player;
public SumoTankAI bot;
public SumoUIManager sumoUIManager;
public Timer timer;
private void Start()
{
player.GetComponent<SumoTankController>();
player.sumoController = this;
player.GetComponentInChildren<SumoTankGun>().sumoController = this;
bot.GetComponent<SumoTankAI>();
bot.sumoController = this;
bot.GetComponentInChildren<SumoTankAIGun>().sumoController = this;
sumoUIManager.matchResultText.gameObject.SetActive(false);
sumoUIManager.timeToStartGame.gameObject.SetActive(true);
}
private void Update()
{
WaitForStartGame();
WaitForEndGame();
}
public void CheckMatchResult()
{
if (!isGameOver)
{
if (isWin)
{
sumoUIManager.matchResultText.text = "Вы победили!";
sumoUIManager.matchResultText.gameObject.SetActive(true);
}
else
{
sumoUIManager.matchResultText.text = "Вы проиграли!";
sumoUIManager.matchResultText.gameObject.SetActive(true);
}
}
}
public void WaitForStartGame()
{
if (timer.waitForStartGame >= 0)
{
sumoUIManager.timeToStartGame.text = $"{timer.waitForStartGame:f1}";
}
else
{
sumoUIManager.timeToStartGame.gameObject.SetActive(false);
isGameStart = true;
}
}
public void WaitForEndGame(){
if (timer.waitForEndGame >= 0)
{
float minutes = Mathf.FloorToInt(timer.waitForEndGame / 60);
float seconds = Mathf.FloorToInt(timer.waitForEndGame % 60);
sumoUIManager.timeToEndGame.text = $"{minutes:00}:{seconds:00}";
}
else
{
sumoUIManager.backBtn.onClick.Invoke();
}
}
}
您已经在计时器下的更新中调用了更新中的方法,因此它们已经在计数但是如果您需要一个计时器,而不是让一个单独的类只包含计时器您可以通过将时间保存在变量中来计算时差然后通过Time.time
或使用协程减去它
在设计课程时,您需要了解课程将/应该做什么。 在本例中,您创建了一个类 Timer。 此类将自行更新并应处理所有时间操作。
考虑一下:当两个人在用时钟进行国际象棋比赛时,为什么击中王子来停止计时器是有意义的?
您可以在 Timer 类中创建一个带有委托的回调,该回调会在满足条件时调用。
最后一个将使您的 SumoController.Update 中的调用变得不必要。
这适用于每个类和方法。 责任,这个类或方法的目的是什么。 那是在每个应用程序中。 在游戏开发中有一个叫做性能的东西,优化代码以减少执行时间非常重要。 这也是一个需要牢记的考虑因素。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.