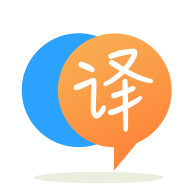
[英]What is the simplest way to create an empty iterable using yield in Python?
[英]What is the simplest way to SSH using Python?
如何从本地 Python (3.0) 脚本简单地通过 SSH 连接到远程服务器、提供登录名/密码、执行命令并将输出打印到 Python 控制台?
我宁愿不使用任何大型外部库或在远程服务器上安装任何东西。
如上所述,您可以使用 Paramiko 自己编写代码。 或者,您可以查看 Fabric,这是一个 python 应用程序,用于执行您询问的所有事情:
Fabric 是一个 Python 库和命令行工具,旨在通过 SSH 协议简化部署应用程序或执行系统管理任务。 它提供了用于运行任意 shell 命令(作为普通登录用户,或通过 sudo)、上传和下载文件等的工具。
我认为这符合您的需求。 它也不是一个大型库,不需要安装服务器,尽管它确实依赖于 paramiko 和 pycrypt,需要在客户端上安装。
* The official, canonical repository is git.fabfile.org
* The official Github mirror is GitHub/bitprophet/fabric
有几篇关于它的好文章,但你应该小心,因为它在过去六个月中发生了变化:
现代 Python 黑客的工具:Virtualenv、Fabric 和 Pip
后来:Fabric 不再需要 paramiko 安装:
$ pip install fabric
Downloading/unpacking fabric
Downloading Fabric-1.4.2.tar.gz (182Kb): 182Kb downloaded
Running setup.py egg_info for package fabric
warning: no previously-included files matching '*' found under directory 'docs/_build'
warning: no files found matching 'fabfile.py'
Downloading/unpacking ssh>=1.7.14 (from fabric)
Downloading ssh-1.7.14.tar.gz (794Kb): 794Kb downloaded
Running setup.py egg_info for package ssh
Downloading/unpacking pycrypto>=2.1,!=2.4 (from ssh>=1.7.14->fabric)
Downloading pycrypto-2.6.tar.gz (443Kb): 443Kb downloaded
Running setup.py egg_info for package pycrypto
Installing collected packages: fabric, ssh, pycrypto
Running setup.py install for fabric
warning: no previously-included files matching '*' found under directory 'docs/_build'
warning: no files found matching 'fabfile.py'
Installing fab script to /home/hbrown/.virtualenvs/fabric-test/bin
Running setup.py install for ssh
Running setup.py install for pycrypto
...
Successfully installed fabric ssh pycrypto
Cleaning up...
然而,这主要是装饰性的:ssh 是 paramiko 的一个分支,两个库的维护者是相同的(Jeff Forcier,也是 Fabric 的作者),并且维护者计划以 paramiko 的名称重新组合 paramiko 和 ssh 。 (通过pbanka进行此更正。)
我还没有尝试过,但是这个pysftp模块可能会有所帮助,它反过来使用 paramiko。 我相信一切都是客户端。
有趣的命令可能是.execute()
,它在远程机器上执行任意命令。 (该模块还具有.get()
和.put
方法,这些方法更多地暗示了它的 FTP 字符)。
更新:
在我最初链接到的博客文章不再可用后,我重新编写了答案。 一些引用此答案旧版本的评论现在看起来很奇怪。
如果你想避免任何额外的模块,你可以使用 subprocess 模块来运行
ssh [host] [command]
并捕获输出。
尝试类似:
process = subprocess.Popen("ssh example.com ls", shell=True,
stdout=subprocess.PIPE, stderr=subprocess.STDOUT)
output,stderr = process.communicate()
status = process.poll()
print output
处理用户名和密码,可以使用 subprocess 与 ssh 进程交互,也可以在服务器上安装一个公钥来避免密码提示。
我已经 为 libssh2 编写了 Python 绑定。 Libssh2 是一个实现 SSH2 协议的客户端库。
import socket
import libssh2
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(('exmaple.com', 22))
session = libssh2.Session()
session.startup(sock)
session.userauth_password('john', '******')
channel = session.channel()
channel.execute('ls -l')
print channel.read(1024)
喜欢hughdbrown,我喜欢Fabric。 请注意,虽然它实现了自己的声明性脚本(用于进行部署等),但它也可以作为 Python 模块导入并在您的程序中使用,而无需编写 Fabric 脚本。
Fabric 有一个新的维护者,正在重写; 这意味着您(当前)在网络上找到的大多数教程都不适用于当前版本。 此外,Google 仍将旧的 Fabric 页面显示为第一个结果。
有关最新文档,您可以查看: http ://docs.fabfile.org
我发现 paramiko 有点太低级了,Fabric 也不是特别适合用作库,所以我将我自己的库放在一起,称为spark ,它使用 paramiko 来实现更好的接口:
import spur
shell = spur.SshShell(hostname="localhost", username="bob", password="password1")
result = shell.run(["echo", "-n", "hello"])
print result.output # prints hello
您还可以选择在程序运行时打印程序的输出,如果您想在退出之前查看长时间运行的命令的输出,这很有用:
result = shell.run(["echo", "-n", "hello"], stdout=sys.stdout)
为了那些到达这里谷歌搜索python ssh示例的人的利益。 原来的问题和答案现在几乎是旧的解码了。 似乎 paramiko 获得了一些功能(好的。我承认 - 在这里纯粹猜测 - 我是 Python 新手),您可以直接使用 paramiko 创建 ssh 客户端。
import base64
import paramiko
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect('192.168.1.1', username='user', password='password')
stdin, stdout, stderr = client.exec_command('cat /proc/meminfo')
for line in stdout:
print('... ' + line.strip('\n'))
client.close()
此代码改编自https://github.com/paramiko/paramiko的演示它适用于我。
这对我有用
import subprocess
import sys
HOST="IP"
COMMAND="ifconfig"
def passwordless_ssh(HOST):
ssh = subprocess.Popen(["ssh", "%s" % HOST, COMMAND],
shell=False,
stdout=subprocess.PIPE,
stderr=subprocess.PIPE)
result = ssh.stdout.readlines()
if result == []:
error = ssh.stderr.readlines()
print >>sys.stderr, "ERROR: %s" % error
return "error"
else:
return result
please refer to paramiko.org, its very useful while doing ssh using python.
import paramiko
import time
ssh = paramiko.SSHClient() #SSHClient() is the paramiko object</n>
#Below lines adds the server key automatically to know_hosts file.use anyone one of the below
ssh.load_system_host_keys()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
#Here we are actually connecting to the server.
ssh.connect('10.106.104.24', port=22, username='admin', password='')
time.sleep(5)
#I have mentioned time because some servers or endpoint prints there own information after
#loggin in e.g. the version, model and uptime information, so its better to give some time
#before executing the command.
#Here we execute the command, stdin for input, stdout for output, stderr for error
stdin, stdout, stderr = ssh.exec_command('xstatus Time')
#Here we are reading the lines from output.
output = stdout.readlines()
print(output)
#Below all are the Exception handled by paramiko while ssh. Refer to paramiko.org for more information about exception.
except (BadHostKeyException, AuthenticationException,
SSHException, socket.error) as e:
print(e)
host = "test.rebex.net"
port = 22
username = "demo"
password = "password"
command = "ls"
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(host, port, username, password)
stdin, stdout, stderr = ssh.exec_command(command)
lines = stdout.readlines()
print(lines)
`
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.