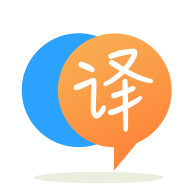
[英]Superimposing image over webcam feed using OpenCV 2.4.7.0 in Python 2.7
[英]Displaying a webcam feed using OpenCV and Python
我一直在尝试使用 Python 创建一个简单的程序,它使用OpenCV从我的网络摄像头获取视频源并将其显示在屏幕上。
我知道我在那里的部分原因是创建了 window 并且我的网络摄像头上的灯闪烁,但它似乎在 window 中没有显示任何内容。 希望有人可以解释我做错了什么。
import cv
cv.NamedWindow("w1", cv.CV_WINDOW_AUTOSIZE)
capture = cv.CaptureFromCAM(0)
def repeat():
frame = cv.QueryFrame(capture)
cv.ShowImage("w1", frame)
while True:
repeat()
在不相关的说明中,我注意到我的网络摄像头有时会更改其在cv.CaptureFromCAM
中的索引号,有时我需要输入 0、1 或 2,即使我只连接了一个摄像头并且我没有拔下它(我知道,因为除非我更改索引,否则灯不会亮起)。 有没有办法让 Python 确定正确的索引?
更新以显示如何在最新版本的OpenCV中执行此操作:
import cv2
cv2.namedWindow("preview")
vc = cv2.VideoCapture(0)
if vc.isOpened(): # try to get the first frame
rval, frame = vc.read()
else:
rval = False
while rval:
cv2.imshow("preview", frame)
rval, frame = vc.read()
key = cv2.waitKey(20)
if key == 27: # exit on ESC
break
cv2.destroyWindow("preview")
vc.release()
它适用于OpenCV-2.4.2。
尝试在repeat()
方法的底部添加c = cv.WaitKey(10)
行。
等待10毫秒,用户输入密钥。 即使你根本没有使用密钥,也要把它放进去。我认为只需要一些延迟,所以time.sleep(10)
也可以工作。
关于相机索引,你可以这样做:
for i in range(3):
capture = cv.CaptureFromCAM(i)
if capture: break
这将找到第一个“工作”捕获设备的索引,至少对于0-2的索引。 您的计算机中可能有多个设备被识别为正确的捕获设备。 我知道确认你有正确的唯一方法是手动查看你的灯。 也许得到一个图像并检查其属性?
要向进程添加用户提示,可以将键绑定到重复循环中的切换摄像头:
import cv
cv.NamedWindow("w1", cv.CV_WINDOW_AUTOSIZE)
camera_index = 0
capture = cv.CaptureFromCAM(camera_index)
def repeat():
global capture #declare as globals since we are assigning to them now
global camera_index
frame = cv.QueryFrame(capture)
cv.ShowImage("w1", frame)
c = cv.WaitKey(10)
if(c=="n"): #in "n" key is pressed while the popup window is in focus
camera_index += 1 #try the next camera index
capture = cv.CaptureFromCAM(camera_index)
if not capture: #if the next camera index didn't work, reset to 0.
camera_index = 0
capture = cv.CaptureFromCAM(camera_index)
while True:
repeat()
免责声明:我没有对此进行过测试,因此它可能存在错误或只是不起作用,但可能至少会让您了解一种解决方法。
如果您只有一台摄像机,或者您不关心哪台摄像机是正确的摄像机,则使用“-1”作为索引。 即你的例子capture = cv.CaptureFromCAM(-1)
。
将import cv
更改import cv2.cv as cv
请参见此处的帖子。
请尝试以下方法。 这很简单,但我还没有想出一个优雅的退出方式。
import cv2.cv as cv
import time
cv.NamedWindow("camera", 0)
capture = cv.CaptureFromCAM(0)
while True:
img = cv.QueryFrame(capture)
cv.ShowImage("camera", img)
if cv.WaitKey(10) == 27:
break
cv.DestroyAllWindows()
与在opencv-doc中一样,您可以通过以下代码从连接到计算机的摄像头获取视频。
import numpy as np
import cv2
cap = cv2.VideoCapture(0)
while(True):
# Capture frame-by-frame
ret, frame = cap.read()
# Our operations on the frame come here
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# Display the resulting frame
cv2.imshow('frame',gray)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# When everything done, release the capture
cap.release()
cv2.destroyAllWindows()
您可以将cap = cv2.VideoCapture(0)
索引从0
更改为1
以访问第二个摄像头。
在opencv-3.2.0
测试过
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.