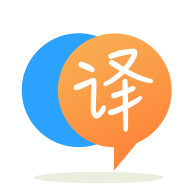
[英]How to print the most recent last call on linux without exiting the program
[英]How to catch and print the full exception traceback without halting/exiting the program?
我想在不退出的情况下捕获并记录异常,例如,
try:
do_stuff()
except Exception as err:
print(Exception, err)
# I want to print the entire traceback here,
# not just the exception name and details
我想打印在没有 try/except 拦截异常的情况下引发异常时打印的完全相同的输出,并且我不希望它退出我的程序。
如果您想要的话, traceback.format_exc()
或sys.exc_info()
将产生更多信息。
import traceback
import sys
try:
do_stuff()
except Exception:
print(traceback.format_exc())
# or
print(sys.exc_info()[2])
其他一些答案已经指出了回溯模块。
请注意,使用print_exc
,在某些极端情况下,您将无法获得预期的结果。 在 Python 2.x 中:
import traceback
try:
raise TypeError("Oups!")
except Exception, err:
try:
raise TypeError("Again !?!")
except:
pass
traceback.print_exc()
...将显示最后一个异常的回溯:
Traceback (most recent call last):
File "e.py", line 7, in <module>
raise TypeError("Again !?!")
TypeError: Again !?!
如果您确实需要访问原始回溯,一种解决方案是将exc_info
返回的异常信息缓存在局部变量中,并使用print_exception
显示它:
import traceback
import sys
try:
raise TypeError("Oups!")
except Exception, err:
try:
exc_info = sys.exc_info()
# do you usefull stuff here
# (potentially raising an exception)
try:
raise TypeError("Again !?!")
except:
pass
# end of useful stuff
finally:
# Display the *original* exception
traceback.print_exception(*exc_info)
del exc_info
生产:
Traceback (most recent call last):
File "t.py", line 6, in <module>
raise TypeError("Oups!")
TypeError: Oups!
不过,这有几个陷阱:
来自sys_info
的文档:
将回溯返回值分配给处理异常的函数中的局部变量将导致循环引用。 这将防止同一函数中的局部变量或回溯引用的任何内容被垃圾收集。 [...]如果您确实需要回溯,请确保在使用后将其删除(最好使用 try ... finally 语句完成)
但是,来自同一个文档:
从 Python 2.2 开始,当启用垃圾收集并且它们变得无法访问时,此类循环会自动回收,但避免创建循环仍然更有效。
另一方面,通过允许您访问与异常关联的回溯,Python 3 产生了一个不那么令人惊讶的结果:
import traceback
try:
raise TypeError("Oups!")
except Exception as err:
try:
raise TypeError("Again !?!")
except:
pass
traceback.print_tb(err.__traceback__)
...将显示:
File "e3.py", line 4, in <module>
raise TypeError("Oups!")
如何在不停止程序的情况下打印完整的回溯?
当您不想因错误而停止程序时,您需要使用 try/except 来处理该错误:
try:
do_something_that_might_error()
except Exception as error:
handle_the_error(error)
要提取完整的回溯,我们将使用标准库中的traceback
模块:
import traceback
并创建一个相当复杂的堆栈跟踪来证明我们获得了完整的堆栈跟踪:
def raise_error():
raise RuntimeError('something bad happened!')
def do_something_that_might_error():
raise_error()
要打印完整的回溯,请使用traceback.print_exc
方法:
try:
do_something_that_might_error()
except Exception as error:
traceback.print_exc()
哪个打印:
Traceback (most recent call last):
File "<stdin>", line 2, in <module>
File "<stdin>", line 2, in do_something_that_might_error
File "<stdin>", line 2, in raise_error
RuntimeError: something bad happened!
但是,最佳实践是为您的模块设置一个记录器。 它将知道模块的名称并能够更改级别(以及其他属性,例如处理程序)
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
在这种情况下,您将需要logger.exception
函数:
try:
do_something_that_might_error()
except Exception as error:
logger.exception(error)
哪些日志:
ERROR:__main__:something bad happened!
Traceback (most recent call last):
File "<stdin>", line 2, in <module>
File "<stdin>", line 2, in do_something_that_might_error
File "<stdin>", line 2, in raise_error
RuntimeError: something bad happened!
或者您可能只需要字符串,在这种情况下,您需要使用traceback.format_exc
函数:
try:
do_something_that_might_error()
except Exception as error:
logger.debug(traceback.format_exc())
哪些日志:
DEBUG:__main__:Traceback (most recent call last):
File "<stdin>", line 2, in <module>
File "<stdin>", line 2, in do_something_that_might_error
File "<stdin>", line 2, in raise_error
RuntimeError: something bad happened!
对于所有三个选项,我们看到我们得到与出现错误时相同的输出:
>>> do_something_that_might_error()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 2, in do_something_that_might_error
File "<stdin>", line 2, in raise_error
RuntimeError: something bad happened!
性能问题在这里并不重要,因为 IO 通常占主导地位。 我更喜欢,因为它以向前兼容的方式精确地完成了请求:
logger.exception(error)
可以调整记录级别和输出,无需触摸代码即可轻松关闭。 通常做直接需要的事情是最有效的方法。
首先,不要使用print
进行日志记录,有一个稳定、经过验证且经过深思熟虑的stdlib
模块可以做到这一点: logging
。 你绝对应该使用它。
其次,当有原生且简单的方法时,不要试图将不相关的工具弄得一团糟。 这里是:
log = logging.getLogger(__name__)
try:
call_code_that_fails()
except MyError:
log.exception('Any extra info you want to see in your logs')
而已。 你现在完成了。
log.exception
实际上所做的只是调用log.error
(即记录级别为ERROR
的事件)然后打印回溯。
好吧,这里有一些注意事项:
traceback
或使用exc_info=True
调用 logger 或使用sys.exc_info
弄脏他们的手? 好吧,只是因为! 它们都存在于不同的目的。 例如, traceback.print_exc
的输出与解释器本身产生的回溯有点不同。 如果你使用它,你会迷惑任何阅读你日志的人,他们会用头撞他们。
传递exc_info=True
来记录调用是不合适的。 但是,当捕获可恢复的错误并且您还希望使用回溯记录它们(使用例如INFO
级别)时,它很有用,因为log.exception
只生成一个级别的日志 - ERROR
。
而且您绝对应该尽可能避免弄乱sys.exc_info
。 它只是不是一个公共接口,它是一个内部接口——如果你确定自己在做什么,你就可以使用它。 它不仅仅用于打印异常。
traceback.format_exception(exception_object)
如果您只有异常对象,则可以从 Python 3 中的任何代码点获取作为字符串的回溯:
import traceback
''.join(traceback.format_exception(None, exc_obj, exc_obj.__traceback__))
完整示例:
#!/usr/bin/env python3
import traceback
def f():
g()
def g():
raise Exception('asdf')
try:
g()
except Exception as e:
exc_obj = e
tb_str = ''.join(traceback.format_exception(None, exc_obj, exc_obj.__traceback__))
print(tb_str)
输出:
Traceback (most recent call last):
File "./main.py", line 12, in <module>
g()
File "./main.py", line 9, in g
raise Exception('asdf')
Exception: asdf
文档: https ://docs.python.org/3.9/library/traceback.html#traceback.format_exception
另请参阅: 从异常对象中提取回溯信息
在 Python 3.9 中测试
除了Aaron Hall 的回答之外,如果您正在记录,但不想使用logging.exception()
(因为它在 ERROR 级别记录),您可以使用较低级别并传递exc_info=True
。 例如
try:
do_something_that_might_error()
except Exception:
logging.info('General exception noted.', exc_info=True)
我没有在任何其他答案中看到这一点。 如果您出于某种原因传递 Exception 对象...
在 Python 3.5+ 中,您可以使用traceback.TracebackException.from_exception()从 Exception 对象获取跟踪。 例如:
import traceback
def stack_lvl_3():
raise Exception('a1', 'b2', 'c3')
def stack_lvl_2():
try:
stack_lvl_3()
except Exception as e:
# raise
return e
def stack_lvl_1():
e = stack_lvl_2()
return e
e = stack_lvl_1()
tb1 = traceback.TracebackException.from_exception(e)
print(''.join(tb1.format()))
但是,上面的代码导致:
Traceback (most recent call last):
File "exc.py", line 10, in stack_lvl_2
stack_lvl_3()
File "exc.py", line 5, in stack_lvl_3
raise Exception('a1', 'b2', 'c3')
Exception: ('a1', 'b2', 'c3')
这只是堆栈的两个级别,与如果在stack_lvl_2()
中引发异常并且没有被拦截(取消注释# raise
行)会在屏幕上打印的内容相反。
据我了解,这是因为异常只记录堆栈的当前级别,在这种情况下是stack_lvl_3()
。 当它通过堆栈向上传递时,更多的级别被添加到它的__traceback__
中。 但是我们在stack_lvl_2()
中截获了它,这意味着它要记录的只是级别 3 和级别 2。要获得打印在标准输出上的完整跟踪,我们必须在最高(最低?)级别捕获它:
import traceback
def stack_lvl_3():
raise Exception('a1', 'b2', 'c3')
def stack_lvl_2():
stack_lvl_3()
def stack_lvl_1():
stack_lvl_2()
try:
stack_lvl_1()
except Exception as exc:
tb = traceback.TracebackException.from_exception(exc)
print('Handled at stack lvl 0')
print(''.join(tb.stack.format()))
结果是:
Handled at stack lvl 0
File "exc.py", line 17, in <module>
stack_lvl_1()
File "exc.py", line 13, in stack_lvl_1
stack_lvl_2()
File "exc.py", line 9, in stack_lvl_2
stack_lvl_3()
File "exc.py", line 5, in stack_lvl_3
raise Exception('a1', 'b2', 'c3')
请注意堆栈打印不同,缺少第一行和最后一行。 因为它是不同的format()
。
尽可能远离引发异常的地方截取异常可以简化代码,同时提供更多信息。
在 python3(适用于 3.9)中,我们可以定义一个函数,并且可以在我们想要打印详细信息的任何地方使用它。
import traceback
def get_traceback(e):
lines = traceback.format_exception(type(e), e, e.__traceback__)
return ''.join(lines)
try:
1/0
except Exception as e:
print('------Start--------')
print(get_traceback(e))
print('------End--------')
try:
spam(1,2)
except Exception as e:
print('------Start--------')
print(get_traceback(e))
print('------End--------')
输出如下:
bash-3.2$ python3 /Users/soumyabratakole/PycharmProjects/pythonProject/main.py
------Start--------
Traceback (most recent call last):
File "/Users/soumyabratakole/PycharmProjects/pythonProject/main.py", line 26, in <module>
1/0
ZeroDivisionError: division by zero
------End--------
------Start--------
Traceback (most recent call last):
File "/Users/soumyabratakole/PycharmProjects/pythonProject/main.py", line 33, in <module>
spam(1,2)
NameError: name 'spam' is not defined
------End--------
如果你已经有一个 Error 对象,并且你想打印整个东西,你需要做这个有点尴尬的调用:
import traceback
traceback.print_exception(type(err), err, err.__traceback__)
没错, print_exception
接受三个位置参数:异常的类型、实际的异常对象和异常自身的内部回溯属性。
在 python 3.5 或更高版本中, type(err)
是可选的......但它是一个位置参数,所以你仍然必须显式地传递 None 。
traceback.print_exception(None, err, err.__traceback__)
我不知道为什么所有这些不仅仅是traceback.print_exception(err)
。 为什么你会想要打印出一个错误,以及一个不属于该错误的回溯,这超出了我的理解。
为了获得精确的堆栈跟踪,作为一个字符串,如果没有 try/except 可以跨过它,那么它会被引发,只需将它放在捕获有问题的异常的 except 块中。
desired_trace = traceback.format_exc(sys.exc_info())
下面是如何使用它(假设flaky_func
被定义,并且log
调用你最喜欢的日志系统):
import traceback
import sys
try:
flaky_func()
except KeyboardInterrupt:
raise
except Exception:
desired_trace = traceback.format_exc(sys.exc_info())
log(desired_trace)
捕获并重新引发KeyboardInterrupt
是个好主意,这样您仍然可以使用 Ctrl-C 终止程序。 日志记录超出了问题的范围,但一个不错的选择是logging 。 sys和traceback模块的文档。
您需要将 try/except 放在可能发生错误的最内循环中,即
for i in something:
for j in somethingelse:
for k in whatever:
try:
something_complex(i, j, k)
except Exception, e:
print e
try:
something_less_complex(i, j)
except Exception, e:
print e
... 等等
换句话说,您需要将可能在 try/except 中失败的语句尽可能具体地包装在尽可能最内层的循环中。
关于这个答案的评论的评论: print(traceback.format_exc())
对我来说比traceback.print_exc()
做得更好。 对于后者, hello
有时会奇怪地与回溯文本“混合”,例如如果两者都想同时写入 stdout 或 stderr,产生奇怪的输出(至少在从文本编辑器内部构建并查看输出时) “构建结果”面板)。
回溯(最近一次通话最后):
文件“C:\Users\User\Desktop\test.py”,第 7 行,在
地狱do_stuff()
do_stuff 中的文件“C:\Users\User\Desktop\test.py”,第 4 行
1/0
ZeroDivisionError:整数除法或以零为模
○
【0.1s完成】
所以我使用:
import traceback, sys
def do_stuff():
1/0
try:
do_stuff()
except Exception:
print(traceback.format_exc())
print('hello')
import io
import traceback
try:
call_code_that_fails()
except:
errors = io.StringIO()
traceback.print_exc(file=errors)
contents = str(errors.getvalue())
print(contents)
errors.close()
你想要回溯模块。 它将让您像 Python 通常那样打印堆栈转储。 特别是, print_last函数将打印最后一个异常和堆栈跟踪。
这是我在日志文件和控制台上写入错误的解决方案:
import logging, sys
import traceback
logging.basicConfig(filename='error.log', level=logging.DEBUG)
def handle_exception(exc_type, exc_value, exc_traceback):
if issubclass(exc_type, KeyboardInterrupt):
sys.__excepthook__(exc_type, exc_value, exc_traceback)
return
exc_info=(exc_type, exc_value, exc_traceback)
logging.critical("\nDate:" + str(datetime.datetime.now()), exc_info=(exc_type, exc_value, exc_traceback))
print("An error occured, check error.log to see the error details")
traceback.print_exception(*exc_info)
sys.excepthook = handle_exception
蟒蛇3解决方案
stacktrace_helper.py
:
from linecache import getline
import sys
import traceback
def get_stack_trace():
exc_type, exc_value, exc_tb = sys.exc_info()
trace = traceback.format_stack()
trace = list(filter(lambda x: ("\\lib\\" not in x and "/lib/" not in x and "stacktrace_helper.py" not in x), trace))
ex_type = exc_type.__name__
ex_line = exc_tb.tb_lineno
ex_file = exc_tb.tb_frame.f_code.co_filename
ex_message = str(exc_value)
line_code = ""
try:
line_code = getline(ex_file, ex_line).strip()
except:
pass
trace.insert(
0, f'File "{ex_file}", line {ex_line}, line_code: {line_code} , ex: {ex_type} {ex_message}',
)
return trace
def get_stack_trace_str(msg: str = ""):
trace = list(get_stack_trace())
trace_str = "\n".join(list(map(str, trace)))
trace_str = msg + "\n" + trace_str
return trace_str
你可以这样做:
try:
do_stuff()
except Exception, err:
print(Exception, err)
raise err
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.