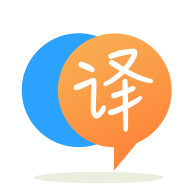
[英]Use Dijkstra's algorithm to get shortest path when distance are as coordinates
[英]Shortest path and distance algorithm for Java?
在下面的代码中,我试图计算两个城市之间的距离。 用户将输入城市名称和城市,然后用户将输入这些城市之间的距离,最后输入这些城市之间的旅行价格。 我还没有对其进行评估,因为我不确定我将如何做到这一点。 我的问题是我正在寻找有关如何做到这一点的指针和建议。
import java.io.*;
import java.util.*;
public class CityCalcultor {
static LinkedList<String> cities = new LinkedList<String>();
static LinkedList<Integer> distance = new LinkedList<Integer>();
static LinkedList<Integer> price = new LinkedList<Integer>();
public static void main(String[] args) throws IOException {
Scanner input = new Scanner(System.in);
String text;
int option = 0;
while (true) {
System.out.println("\nWhat would you like to do:\n"
+ "1. Add a city to the system\n"
+ "2. Add a path to the system\n"
+ "3. Evalute paths\n"
+ "4. Exit\n" + "Your option: ");
text = input.nextLine();
option = Integer.parseInt(text);
switch (option) {
case 1:
EnterCity();
break;
case 2:
EnterPath();
break;
case 3:
EvalutePaths();
break;
case 4:
return;
default:
System.out.println("ERROR INVALID INPUT");
}
}
}
public static void EnterCity() {
String c = "";
LinkedList<String> cities = new LinkedList<String>(Arrays.asList(c));
Scanner City = new Scanner(System.in);
System.out.println("Please enter the city name ");
c = City.nextLine();
cities.add(c);
System.out.println("City " + c + " has been added ");
}
public static void EnterPath() {
Scanner Path = new Scanner(System.in);
int d = 0;
int p = 0;
System.out.println("Enter the starting city ");
System.out.println();
System.out.println(Path.nextLine());
System.out.println("Enter the ending city ");
System.out.println(Path.nextLine());
System.out.println("Enter the distance between the two cities ");
d = Path.nextInt();
for (d = 0; d > 0; d++) {
distance.add(d);
}
System.out.println("Enter the price between the two cities ");
p = Path.nextInt();
for (p = 0; p > 0; p++) {
price.add(p);
}
System.out.println("The route was sucessfully added ");
}
private static void EvalutePaths() {
}
}
计算最短路径的标准方法是A*
我可以通过两种方式来解释您的问题:您要么想评估成对城市之间的每条可能路径(这在技术上是无限的,除非您限制重新访问城市),或者您想找到最短距离/最便宜的出行方式城市到城市。
我不想试图弄清楚第一个,我很确定你的意思是第二个,所以我会这样做。
问题的解决方案是 model 您的城市以及它们之间的路线使用图,其中每个顶点是一个城市,每个边是两个城市之间的直接路径。 边缘根据城市之间的距离或旅行成本加权。
然后,您可以应用Dijkstra 算法(或其变体之一)以找到任何源顶点(出发城市)到所有其他顶点(目的地城市)之间总权重最小(即最小距离或最低成本)的路径。 如果您依次使用每个顶点作为源应用此算法,您将构建任意两个城市之间最便宜的路线的完整 model。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.