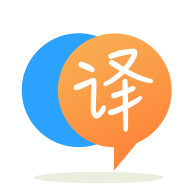
[英]C++ OpenCV: Convert vector<vector<Point>> to vector<vector<Point2f>>
[英]convert OpenCV 2 vector<Point2i> to vector<Point2f>
OpenCV 2 轮廓查找器返回一个vector<Point2i>
,但有时您希望将它们与需要vector<Point2f>
的函数一起使用。 什么是最快、最优雅的转换方式?
这里有一些想法。 任何可以转换为Mat
非常通用的转换函数:
template <class SrcType, class DstType>
void convert1(std::vector<SrcType>& src, std::vector<DstType>& dst) {
cv::Mat srcMat = cv::Mat(src);
cv::Mat dstMat = cv::Mat(dst);
cv::Mat tmpMat;
srcMat.convertTo(tmpMat, dstMat.type());
dst = (vector<DstType>) tmpMat;
}
但这使用了额外的缓冲区,因此并不理想。 这是一种预先分配向量然后调用copy()
:
template <class SrcType, class DstType>
void convert2(std::vector<SrcType>& src, std::vector<DstType>& dst) {
dst.resize(src.size());
std::copy(src.begin(), src.end(), dst.begin());
}
最后,使用back_inserter
:
template <class SrcType, class DstType>
void convert3(std::vector<SrcType>& src, std::vector<DstType>& dst) {
std::copy(src.begin(), src.end(), std::back_inserter(dst));
}
假设 src 和 dst 是向量,在 OpenCV 2.x 中你可以说:
cv::Mat(src).copyTo(dst);
在 OpenCV 2.3.x 中,你可以说:
cv::Mat(src).convertTo(dst, cv::Mat(dst).type());
注意: type()是Mat的函数,而不是std::vector类的函数。 因此,您不能调用dst.type() 。 如果您使用 dst 作为输入创建一个 Mat 实例,那么您可以为新创建的对象调用函数type() 。
请注意,从 cv::Point2f 转换为 cv::Point2i 可能会产生意外结果。
float j = 1.51;
int i = (int) j;
printf("%d", i);
将导致“1”。
尽管
cv::Point2f j(1.51, 1.49);
cv::Point2i i = f;
std::cout << i << std::endl;
将导致“2, 1”。
这意味着, Point2f 到 Point2i 将四舍五入,而类型转换将截断。
http://docs.opencv.org/modules/core/doc/basic_structures.html#point
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.