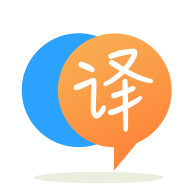
[英]Find numbers in Python list which are within a certain distance of each other
[英]In Python, how can I check if 2 numbers in a list are within a certain percentage of each other?
我有很多數字,我想看看它們中的任何一個是否近似相等。 如果2個數字“大約相等”(就我的目的而言),則兩者都在10%之內(請參閱以下兩個示例。)然后,我要將它們分類為大約相等的數字的單獨列表。
示例1比較5.0和5.5:5.5 +/- 10%= 4.95到6.05(並且5.0在此范圍內)5.0 +/- 10%= 4.50到5.50(並且5.5在此范圍內)因此,5.0和5.5是大致相等。
示例#2比較5.0和5.6:5.6 +/- 10%= 5.04至6.16(且5.0處於此范圍內)5.0 +/- 10%= 4.50至5.50(且5.6不在此范圍內)因此,5.0和5.6不近似相等。
我需要做的總結:輸入= {4.0,4.1,4.2,4.0,9.0,9.4,8.9,4.3}所需的輸出= {4.0,4.1,4.2,4.0,4.3}和{9.0,9.4,8.9}
input_list = [4.0, 4.1, 4.2, 4.0, 9.0, 9.4, 8.9, 4.3]
results = {input_list[0]: [input_list[0]]} # Start with first value
for value in input_list[1:]: # loop through our entire list after first value
hi = value * 1.1
low = value * 0.9
print("Value: {0}\tHi: {1}\tLow:{2}".format(value, hi, low))
for existing in results: # search through our result set
found_similar = False
if low < existing < hi: # if we find a match
results[existing].append(value) # we add our value to the list for that set
found_similar = True
break
if not found_similar: # if we looped through our entire results without a match
results[value] = [value] # Create a new entry in our results dictionary
for entry in results:
print(results[entry])
會給:
results = { 9.0: [9.0, 9.4, 8.9],
4.0: [4.0, 4.1, 4.2, 4.0, 4.3] }
該代碼以列表中的第一個值開頭,並查找該值的10%以內的所有后續值。 因此,在您的示例中,它以4開頭,並找到所有相似的值。 不在10%之內的任何值都會添加到新的“集合”中。
因此,一旦它達到9.0,就會發現它不匹配,因此它將新的結果集添加到results
字典中,鍵為9.0
。 現在考慮9.4時,它在4.0列表中找不到匹配項,但在9.0列表中找到了匹配項。 因此,它將此值添加到第二個結果集中。
這是基於生成器/集合的方法。
def set_gen(nums):
for seed in sorted(nums):
yield tuple([n for n in nums if seed <= n and n/seed <= 1.1])
def remove_subsets(sets):
for s in sets.copy():
[sets.remove(s2) for s2 in sets.difference([s]) if set(s2).issubset(s)]
>>> nums = [4.0, 4.1, 4.2, 4.0, 9.0, 9.4, 8.9, 4.3]
>>> x = set(num for num in set_gen(nums))
>>> remove_subsets(x)
>>> list(x)
[(9.0, 9.4, 8.9), (4.0, 4.1, 4.2, 4.0, 4.3)]
>>> nums = [1.0, 1.1, 1.2, 1.3, 1.4, 1.5, 1.6, 1.7, 1.8, 1.9, 2.0]
>>> x = set(num for num in set_gen(nums))
>>> remove_subsets(x)
>>> list(x)
[(1.9, 1.8), (1.5, 1.4), (1.4, 1.3), (1.2, 1.1), (1.7, 1.6), (1.5, 1.6), (1.3, 1.2), (1.9, 2.0), (1.0, 1.1), (1.8, 1.7)]
您可以這樣做:
Input = {4.0, 4.1, 4.2, 4.0, 9.0, 9.4, 8.9, 4.3}
wl=sorted(Input,reverse=True)
apr=.1
out={}
while wl:
wn=wl.pop()
out[wn]=[wn]
while wl and wl[-1]<=wn*(1+apr):
out[wn].append(wl.pop())
print [(k,out[k]) for k in sorted(out.keys())]
印刷品:
[(4.0, [4.0, 4.1, 4.2, 4.3]), (8.9, [8.9, 9.0, 9.4])]
嘗試注釋中的示例:
>>> Input = {1.0, 1.1, 1.2, 1.3, 1.4, 1.5, 1.6, 1.7, 1.8, 1.9, 2.0}
印刷品:
[(1.0, [1.0, 1.1]), (1.2, [1.2, 1.3]), (1.4, [1.4, 1.5]), (1.6, [1.6, 1.7]), (1.8, [1.8, 1.9]), (2.0, [2.0])]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.