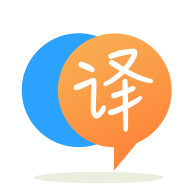
[英]In Python, how can I check if 2 numbers in a list are within a certain percentage of each other?
[英]Find numbers in Python list which are within a certain distance of each other
我在Python中有一個數字列表。 它看起來像這樣:
a = [87, 84, 86, 89, 90, 2014, 1000, 1002, 997, 999]
我希望將所有數字保持在彼此的+或 - 7之內並丟棄其余的數字。 有沒有一種簡單的方法在Python中執行此操作? 我已經閱讀了有關列表過濾器方法的內容,但我不確定如何獲得我想要使用它的內容。
我是Python的新手。
更新
理想情況下,輸出為[84,86,87,89,90]和另一個列表[997,999,1000,1002]。 我想檢索序列而不是異常值。 希望這是有道理的。
這是算法問題,試試這個:
def get_blocks(values):
mi, ma = 0, 0
result = []
temp = []
for v in sorted(values):
if not temp:
mi = ma = v
temp.append(v)
else:
if abs(v - mi) < 7 and abs(v - ma) < 7:
temp.append(v)
if v < mi:
mi = v
elif v > ma:
ma = v
else:
if len(temp) > 1:
result.append(temp)
mi = ma = v
temp = [v]
return result
a = [87, 84, 86, 89, 90, 2014, 1000, 1002, 997, 999]
print get_blocks(a)
輸出:
[[84, 86, 87, 89, 90], [997, 999, 1000, 1002]]
如果你的問題允許傳遞關系,即x在群組中,只要距離群組中的任何元素最多7個,那么這在我看來就像是一個圖論問題。 更具體地說,您需要找到所有連接的組件 。
使用遞歸算法很容易解決問題本身。 您首先要創建一個字典,其中每個鍵都是其中一個元素,每個值都是一個元素列表,與該元素相距最多7個。 對於你的例子,你會有這樣的事情:
for element in elements:
connections[element] = []
visited[element] = False
for another in elements:
if abs(element - another) <= limit:
connections[element].append(another)
哪個會給你這樣的東西
{
84: [86, 87, 89, 90],
86: [84, 87, 89, 90],
...
997: [999, 1000, 1002]
...
2014: []
}
現在你需要編寫一個遞歸函數,它將把元素和列表作為輸入,只要它能找到一個與當前元素最多7的元素,它將繼續在列表中添加元素。
def group_elements(element, group):
if visited[element]:
return
visited[element] = True
group.append(element)
for another in connections[element]:
group_elements(another, group)
在代碼中的某處,您還需要記住已經訪問過的元素,以確保不會進入無限循環。
visited = {}
您需要為列表中的每個元素調用該函數。
groups = []
for element in elements:
if not visited[element]:
group = []
group_elements(element, group)
groups.append(group)
print group
此代碼應為您的輸入提供以下輸出:
[[87, 84, 86, 89, 90], [2014], [1000, 1002, 997, 999]]
a = [87, 84, 86, 89, 90, 2014, 1000, 1002, 997, 999]
temp=a[0]
result=[]
temp1=[]
counter =len(a)
for i in a:
if i in range(temp-7,temp+7):
temp1.append(i)
if counter==1:
result.append(temp1)
else:
if temp1:
result.append(sorted(temp1))
temp1=[]
temp=i
counter=counter-1
print result
對於像這樣的任何問題,我的第一個調用端口是Python itertools模塊 。 我在代碼中使用的那個鏈接的成對函數在more-itertools模塊中可用。
from more_itertools import pairwise
results = []
chunk = []
a = [87, 84, 86, 89, 90, 2014, 1000, 1002, 997, 999]
a.sort()
for v1, v2 in pairwise(a):
if v2 - v1 <= 7:
chunk.append(v1)
elif chunk:
chunk.append(v1)
results.append(chunk)
chunk = []
print(results)
[[84, 86, 87, 89, 90], [997, 999, 1000, 1002]]
我沒有測試邊緣情況,所以它的買家要小心:)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.