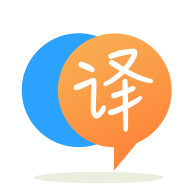
[英]Text of ListView Subitems not updated when using AddRange (WinForms)
[英]Find exact text inside Listview subitems?
在我的應用程序中,我想在列表視圖中添加3個子項
第一個子項目是:該項目的索引號
第二個子項目是:該項目的說明
最后一個子項是:目錄路徑
例:
在添加新項目之前,我嘗試搜索列表視圖是否已經包含具有我已創建的功能的第三個子項目(目錄路徑):
' Find ListView Text
Private Function Find_ListView_Text(ByVal ListView As ListView, ByVal Text As String) As Boolean
Try : Return Convert.ToBoolean(ListView.FindItemWithText(Text)) : Catch : Return True : End Try
End Function
...現在,問題是,例如,如果我首先在列表視圖中添加一個包含目錄“ C:\\ electro”的項目作為圖像中的第三個子項目,那么以后我將無法添加帶有“ C:\\”目錄,因為我的函數未在搜索全文,當我搜索“ C:\\”時,我的函數會找到“ C:\\ Electro”,它會搜索一段文本,否則我需要。
然后,我需要改進在列表視圖內搜索全文而不是文本的功能。
最后一個例子:
如果我在列表視圖中有一個字符串為“ C:\\ Electro”的項目,並且搜索列表視圖中的項目是否存在“ C:\\”,則所需結果為“ FALSE”(目錄C:\\不存在,為C :\\電)
更新:
如果您想了解我的意思,請從該類中提取一個代碼示例...
Private Sub TextBoxes_Sendto_TextChanged(sender As Object, e As EventArgs) _
Handles TextBox_Sendto_Directory.TextChanged, _
TextBox_Sendto_Description.TextChanged
If TextBox_Sendto_Description.TextLength <> 0 _
AndAlso TextBox_Sendto_Directory.TextLength <> 0 Then
If Not Find_ListView_Text(ListView_Sendto, TextBox_Sendto_Directory.Text) Then
Label_Sendto_Status.Text = "Directory ready to add"
Label_Sendto_Status.ForeColor = Color.YellowGreen
Button_Sendto_Add.Enabled = True
Else
Label_Sendto_Status.Text = "Directory already added"
Label_Sendto_Status.ForeColor = Color.Red
Button_Sendto_Add.Enabled = False
End If
Else
Button_Sendto_Add.Enabled = False
End If
End Sub
Private Sub Button_Sendto_Add_Click(sender As Object, e As EventArgs) Handles Button_Sendto_Add.Click
Dim item = ListView_Sendto.AddItem(ListView_Sendto.Items.Count + 1)
item.SubItems.Add(TextBox_Sendto_Description.Text)
item.SubItems.Add(TextBox_Sendto_Directory.Text)
End Sub
' Find ListView Text
Private Function Find_ListView_Text(ByVal ListView As ListView, ByVal Text As String) As Boolean
Try : Return Convert.ToBoolean(ListView.FindItemWithText(Text)) : Catch : Return True : End Try
End Function
這不是一個正確的答案,但是您可以嘗試以下一種技巧:
只需再添加一列(隱藏)
並輸入"^" + Directory Path + "^"
,然后搜索^C:\\^
,它將只找到C:\\
而不是C:\\Electro
這是一個簡單的小函數,它接受ListViewItemcollection,從零開始的列索引和搜索字符串,並返回該字符串中任何子項目中是否存在搜索字符串:
Private Function FindItem(ItemList As ListView.ListViewItemCollection, ColumnIndex As Integer, SearchString As String) As Boolean
For Each Item As ListViewItem In ItemList
If Item.SubItems(ColumnIndex).Text = SearchString Then
Return True
End If
Next
Return False
End Function
您可以這樣稱呼它:
If Not FindItem(ListView_Sendto, 2, TextBox_Sendto_Directory.Text) Then
比較不區分大小寫。 如果要區分大小寫,可以使用CompareTo方法而不是相等方法。
如有必要,您可以添加錯誤捕獲,以防列索引超出范圍。
終於...我做了摘錄:
#Region " [ListView] Find ListView Text "
' [ListView] Find ListView Text Function
'
' // By Elektro H@cker
'
' Examples :
' MsgBox(Find_ListView_Text(ListView1, "Test"))
' MsgBox(Find_ListView_Text(ListView1, "Test", 2, True, True))
' If Find_ListView_Text(ListView1, "Test") Then...
Private Function Find_ListView_Text(ByVal ListView As ListView, _
ByVal SearchString As String, _
Optional ByVal ColumnIndex As Int32 = Nothing, _
Optional ByVal MatchFullText As Boolean = True, _
Optional ByVal IgnoreCase As Boolean = True) As Boolean
Dim ListViewColumnIndex As Int32 = ListView.Columns.Count - 1
Select Case ColumnIndex
Case Is < 0, Is > ListViewColumnIndex ' ColumnIndex is out of range
Throw New Exception("ColumnIndex is out of range. " & vbNewLine & _
"ColumnIndex Argument: " & ColumnIndex & vbNewLine & _
"ColumnIndex ListView: " & ListViewColumnIndex)
Case Nothing ' ColumnIndex is nothing
If MatchFullText AndAlso IgnoreCase Then ' Match full text, All columns, IgnoreCase
For Each Item As ListViewItem In ListView.Items
For X As Int32 = 0 To ListViewColumnIndex
If Item.SubItems(X).Text.ToLower = SearchString.ToLower Then Return True
Next
Next
ElseIf MatchFullText AndAlso Not IgnoreCase Then ' Match full text, All columns, CaseSensitive
For Each Item As ListViewItem In ListView.Items
For X As Int32 = 0 To ListViewColumnIndex
If Item.SubItems(X).Text = SearchString Then Return True
Next
Next
ElseIf Not MatchFullText AndAlso IgnoreCase Then ' Match part of text, All columns, IgnoreCase
If ListView1.FindItemWithText(SearchString) IsNot Nothing Then _
Return True _
Else Return False
ElseIf Not MatchFullText AndAlso Not IgnoreCase Then ' Match part of text, All columns, CaseSensitive
For Each Item As ListViewItem In ListView.Items
For X As Int32 = 0 To ListViewColumnIndex
If Item.SubItems(X).Text.Contains(SearchString) Then Return True
Next
Next
End If
Case Else ' ColumnIndex is other else
If MatchFullText AndAlso IgnoreCase Then ' Match full text, ColumnIndex, IgnoreCase
For Each Item As ListViewItem In ListView.Items
If Item.SubItems(ColumnIndex).Text.ToLower = SearchString.ToLower Then Return True
Next
ElseIf MatchFullText AndAlso Not IgnoreCase Then ' Match full text, ColumnIndex, CaseSensitive
For Each Item As ListViewItem In ListView.Items
If Item.SubItems(ColumnIndex).Text = SearchString Then Return True
Next
ElseIf Not MatchFullText AndAlso IgnoreCase Then ' Match part of text, ColumnIndex, IgnoreCase
For Each Item As ListViewItem In ListView.Items
If Item.SubItems(ColumnIndex).Text.ToLower.Contains(SearchString.ToLower) Then Return True
Next
ElseIf Not MatchFullText AndAlso Not IgnoreCase Then ' Match part of text, ColumnIndex, CaseSensitive
For Each Item As ListViewItem In ListView.Items
If Item.SubItems(ColumnIndex).Text.Contains(SearchString) Then Return True
Next
End If
End Select
Return False
End Function
#End Region
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.