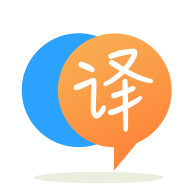
[英]Python - List of Tuples, sum of tuples having same first value in this case?
[英]How do I sum tuples in a list where the first value is the same?
我有一個作為元組的股票和頭寸列表。 買入為正,賣出為負。 例子:
p = [('AAPL', 50), ('AAPL', -50), ('RY', 100), ('RY', -43)]
我怎樣才能總結股票的頭寸,以獲得當前的持股量?
result = [('AAPL', 0), ('RY', 57)]
這個怎么樣? 您可以閱讀有關collections.defaultdict
的信息。
>>> from collections import defaultdict
>>> testDict = defaultdict(int)
>>> p = [('AAPL', 50), ('AAPL', -50), ('RY', 100), ('RY', -43)]
>>> for key, val in p:
testDict[key] += val
>>> testDict.items()
[('AAPL', 0), ('RY', 57)]
這是一個不涉及導入的解決方案:
>>> p = [('AAPL', 50), ('AAPL', -50), ('RY', 100), ('RY', -43)]
>>> d = {x:0 for x,_ in p}
>>> for name,num in p: d[name] += num
...
>>> Result = map(tuple, d.items())
>>> Result
[('AAPL', 0), ('RY', 57)]
>>>
請注意,這是針對 Python 2.x 的。 在 3.x 中,您需要執行以下操作: Result = list(map(tuple, d.items()))
。
我會使用collections.Counter
來做到這一點:
In [2]: from collections import Counter
In [3]: c = Counter()
In [4]: for k, v in p:
...: c[k] += v
...:
In [5]: c
Out[5]: Counter({'AAPL': 0, 'RY': 57})
然后您可以調用Counter
對象的most_common
方法來獲取按值降序排序的元組列表。
In [5]: c.most_common()
Out[5]: [('RY', 57), ('AAPL', 0)]
如果您需要按元組的第一個元素對元組進行排序,請使用sorted(c.items())
:
In [6]: sorted(c.items())
Out[6]: [('AAPL', 0), ('RY', 57)]
沒有任何顯式循環:
>>> from itertools import groupby
>>> from operator import itemgetter
>>> p = [('AAPL', 50), ('AAPL', -50), ('RY', 100), ('RY', -43)]
>>> map(lambda g: (g[0], sum(map(itemgetter(1), g[1]))),
... groupby(sorted(p), itemgetter(0)))
[('AAPL', 0), ('RY', 57)]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.