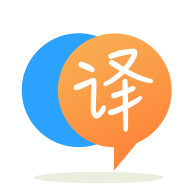
[英]WPF Client with WCF Service from Localhost dev to prod server?
[英]WCF NamedPipes Service and WPF Client
我正在使用Windows服務,我也想在其中添加GUI。 我通過服務創建ServiceHost對象並托管WCF命名管道服務進行了概念驗證,然后通過i Console應用程序使用了WCF服務,還從該服務獲取了回調響應(從服務器發送到連接的客戶端的消息)。 我的控制台應用程序可以很好地運行,並且可以不中斷或延遲地從服務獲得響應。
但是,當在我的WPF GUI應用程序中做同樣的事情時,單擊一個按鈕然后調用WCF服務,它將凍結整個UI線程,然后在幾分鍾后引發異常,然后用消息回調更新UI(服務器發送消息發送給已連接的客戶端),但由於引發了異常,因此服務的所有返回值都將丟失。
我收到的兩條異常消息是論文(最常見的是第一條):
1: 發送到net.pipe:/// localhost / PipeGUI的請求操作在指定的超時時間(00:00:59.9989999)內未收到響應。 分配給該操作的時間可能是較長超時的一部分。 這可能是因為該服務仍在處理該操作,或者是因為該服務無法發送回復消息。 提高操作期限(通過將通道/代理輸入到“圖標文本通道”並設置屬性“操作超時”),並驗證服務是否可以連接到客戶端。
2: 通信對象System.ServiceModel.Channels.ServiceChannel,由於已被取消,因此無法用於通信。
有人知道為什么會這樣嗎? 如果需要,我可以發布更多代碼。
UPDATE,添加了代碼以供參考
public interface IClientCallback
{
[OperationContract(IsOneWay = true)]
void MessageRecived(string message);
}
[ServiceContract(SessionMode = SessionMode.Required, CallbackContract = typeof(IClientCallback))]
public interface IPipeServiceContract
{
[OperationContract]
string Hello();
[OperationContract]
void Message(string msg);
[OperationContract(IsInitiating = true)]
void Connect();
[OperationContract(IsTerminating = true)]
void Disconnect();
}
[ServiceBehavior(InstanceContextMode = InstanceContextMode.Single, IncludeExceptionDetailInFaults = true, UseSynchronizationContext = false)]
public class PipeService : IPipeServiceContract
{
List<IClientCallback> _clients = new List<IClientCallback>();
public string Hello()
{
PublishMessage("Hello World.");
return "Return from method!";
}
public void Connect()
{
_clients.Add(OperationContext.Current.GetCallbackChannel<IClientCallback>());
}
public void Disconnect()
{
IClientCallback callback = OperationContext.Current.GetCallbackChannel<IClientCallback>();
_clients.Remove(callback);
}
void PublishMessage(string message)
{
for (int i = _clients.Count - 1; i > 0; i--)
{
try
{
_clients[i].MessageRecived(message);
}
catch (CommunicationObjectAbortedException coae)
{
_clients.RemoveAt(i);
}
catch(CommunicationObjectFaultedException cofe)
{
_clients.RemoveAt(i);
}
}
}
public void Message(string msg)
{
PublishMessage(msg);
}
}
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window, INotifyPropertyChanged, IClientCallback
{
public ServiceController Service { get; set; }
protected IPipeServiceContract Proxy { get; set; }
protected DuplexChannelFactory<IPipeServiceContract> PipeFactory { get; set; }
public ObservableCollection<ServerActivityNotification> Activity { get; set; }
public override void BeginInit()
{
base.BeginInit();
PipeFactory = new DuplexChannelFactory<IPipeServiceContract>(this, new NetNamedPipeBinding(), new EndpointAddress("net.pipe://localhost/PipeGUI"));
}
public MainWindow()
{
InitializeComponent();
Activity = new ObservableCollection<ServerActivityNotification>();
Service = ServiceController.GetServices().First(x => x.ServiceName == "Server Service");
NotifyPropertyChanged("Service");
var timer = new DispatcherTimer();
timer.Tick += new EventHandler(OnUpdate);
timer.Interval = new TimeSpan(0, 0, 0, 0, 850);
timer.Start();
if (Service.Status == ServiceControllerStatus.Running)
{
Proxy = PipeFactory.CreateChannel();
Proxy.Connect();
}
}
void OnUpdate(object sender, EventArgs e)
{
Service.Refresh();
NotifyPropertyChanged("Service");
StartButton.IsEnabled = Service.Status != ServiceControllerStatus.Running ? true : false;
StopButton.IsEnabled = Service.Status != ServiceControllerStatus.Stopped ? true : false;
if (PipeFactory != null && Service.Status == ServiceControllerStatus.Running)
{
Proxy = PipeFactory.CreateChannel();
Proxy.Connect();
}
}
public event PropertyChangedEventHandler PropertyChanged;
public void NotifyPropertyChanged(string name)
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(name));
}
private void OnStart(object sender, RoutedEventArgs e)
{
try
{
Service.Start();
}
catch
{
Service.Refresh();
}
}
private void OnStop(object sender, RoutedEventArgs e)
{
try
{
if (Proxy != null)
{
Proxy.Disconnect();
PipeFactory.Close();
}
Service.Stop();
}
catch
{
Service.Refresh();
}
}
public void MessageRecived(string message)
{
Dispatcher.BeginInvoke(DispatcherPriority.Background, new Action(() =>
{
ServerActivityNotification log = new ServerActivityNotification { Activity = message, Occured = DateTime.Now };
Activity.Add(log);
ListBoxLog.ScrollIntoView(log);
NotifyPropertyChanged("Activity");
}));
}
private void OnHello(object sender, RoutedEventArgs e)
{
try
{
Proxy.Message(txtSendMessage.Text);
}
catch (Exception ex)
{
Debug.WriteLine(ex.Message);
}
}
}
}
嘗試將服務行為的UseSynchronizationContext
屬性設置為false
:
[ServiceBehavior(UseSynchronizationContext = false)]
class MyService
{
}
[ServiceContract]
public interface IMyService
{
}
我相信默認情況下將其設置為true
,因此您當前正在嘗試在同一線程上使用和運行WCF服務,從而導致死鎖。
在任何情況下,聽起來您都想在WPF應用程序的UI線程上使用WCF服務。 通常,建議您在后台線程上執行可能會長時間運行的任務,因為即使您的服務呼叫需要花費幾秒鍾/分鍾,也可以使界面保持響應狀態。
編輯:
我嘗試並成功地復制了您的問題。 嘗試在UI線程上調用服務會導致UI凍結。 但是,當我更改代碼以在后台任務上調用服務(見下文)時,我能夠調用服務並接收回調:
private void Button_Click(object sender, RoutedEventArgs e)
{
Task.Factory.StartNew(() =>
{
var myService = DuplexChannelFactory<IMyService>.CreateChannel(new CallbackImplementation(),
new WSDualHttpBinding(),
new EndpointAddress(
@"http://localhost:4653/myservice"));
myService.CallService();
string s = "";
});
}
我不得不承認,我並不完全確定為什么會這樣,關於WCF如何確切地管理托管服務實例的線程的任何澄清都將有助於弄清其工作原理。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.