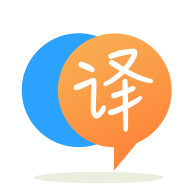
[英]Sort an array and return index of arrays according to elements size in c#
[英]sorting many arrays according to a sorted index of an array C#
int[] a = {120, 60, 50, 40, 30, 20};
int[] b = {12, 29, 37, 85, 63, 11};
int[] c = {30, 23, 90 ,110, 21, 34};
現在我想對a進行排序,並使用其索引對b和c進行排序
例如:
sorted a = {20,30,40,50,60,120};
sorted b should be ={ 11,63,85,37,29,12};
and sorted c should be = { 34,21,110,90,23,30};
如何在C#中做到
一種選擇:
int[] a = {120, 60, 50, 40, 30, 20};
int[] b = {12, 29, 37, 85, 63, 11};
int[] c = {30, 23, 90 ,110, 21, 34};
var ordered = a.Select((item, index) =>
Tuple.Create(item, b[index], c[index]))
.OrderBy(tuple => tuple.Item1).ToArray();
a = ordered.Select(tuple => tuple.Item1).ToArray();
b = ordered.Select(tuple => tuple.Item2).ToArray();
c = ordered.Select(tuple => tuple.Item3).ToArray();
您可以使用間接層來完成此操作,如下所示:
如果需要,可以使用索引定義的順序制作a,b和c的新副本。 但是您也可以選擇不修改原始數組。
不修改原始數組的此選項非常有趣。 它允許您同時激活多個同時訂購。
感到無聊,所以我嘗試最小化新對象的創建和排序。
static void Main(string[] args)
{
int[] a = { 120, 60, 50, 40, 30, 20 };
int[] b = { 12, 29, 37, 85, 63, 11 };
int[] c = { 30, 23, 90, 110, 21, 34 };
var indexes = Enumerable.Range(0, a.Length).OrderBy(i => a[i]).ToArray();
var temp = new int[a.Length];
foreach (var arr in new[] { a, b, c })
{
for (int i = 0; i < a.Length; i++) temp[i] = arr[indexes[i]];
for (int i = 0; i < a.Length; i++) arr[i] = temp[i];
}
Console.WriteLine(String.Join(", ", a));
Console.WriteLine(String.Join(", ", b));
Console.WriteLine(String.Join(", ", c));
Console.ReadLine();
}
可能不是最好的(我敢肯定您可以以某種方式擺脫temp數組)-但這是一個不同的解決方案。 在性能成為問題之前,我將支持堅持使用LINQesque解決方案。
您可以為此使用SortedList。 SortedList使用鍵對項目進行排序。 此外,它還允許您將新項目添加到您的收藏中。
SortedList Class:
Represents a collection of key/value pairs that are sorted by the keys and are accessible by key and by index.
我建議使用LINQ,因為Eli Arbel提供了答案。 但是對於不了解LINQ的用戶,這是另一種解決方案。
class Program
{
public static int get_key(int key , int [,] keylist)
{
for (int i = 0; i <= keylist.GetUpperBound(0); ++i)
{
if (keylist[i, 0] == key)
return keylist[i, 1];
}
return -1;
}
public static int[] Sort_by_index(int [] arr , int [] key , int [,] index_list)
{
int[] _out = new int[arr.Length];
for (int i = 0; i < key.Length; i++)
{
//Get key index
int key_index = get_key(key[i], index_list);
_out[i] = arr[key_index];
}
return _out;
}
static void Main(string[] args)
{
int[] a = { 120, 60, 50, 40, 30, 20 };
int[] b = { 12, 29, 37, 85, 63, 11 };
int[] c = { 30, 23, 90, 110, 21, 34 };
int[,] a_index = { { 120, 0 }, { 60, 1 }, { 50, 2 }, { 40, 3 }, { 30, 4 }, { 20, 5 } };
Array.Sort(a);
b =Sort_by_index(b, a, a_index);
c =Sort_by_index(c, a, a_index);
Console.WriteLine("Result A");
Console.WriteLine(string.Join(", ",a));
Console.WriteLine("Result B");
Console.WriteLine(string.Join(", ",b));
Console.WriteLine("Result C");
Console.WriteLine(string.Join(", ",c));
Console.ReadKey(false);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.