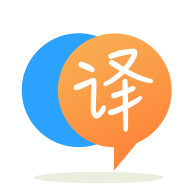
[英]Dijkstra algorithm to find shortest path between two nodes in big graph?
[英]Does Dijkstra algorithm keep track of visited nodes to find the shortest path?
我發現Dijkstra algorithm
這個代碼可以在加權圖中找到兩個節點之間的最短路徑。 我看到的是代碼沒有跟蹤被訪問的節點。 但它適用於我嘗試的所有輸入。 我添加了一行代碼來跟蹤訪問過的節點。 它仍然可以正常工作。 我在這段代碼中已經注釋掉了。 那么是否要求訪問節點? 它有什么影響O
import java.util.PriorityQueue;
import java.util.List;
import java.util.ArrayList;
import java.util.Collections;
class Vertex implements Comparable<Vertex>
{
public final String name;
public Edge[] adjacencies;
public double minDistance = Double.POSITIVE_INFINITY;
public Vertex previous;
public Vertex(String argName) { name = argName; }
public String toString() { return name; }
public int compareTo(Vertex other)
{
return Double.compare(minDistance, other.minDistance);
}
}
class Edge
{
public final Vertex target;
public final double weight;
public Edge(Vertex argTarget, double argWeight)
{ target = argTarget; weight = argWeight; }
}
public class Dijkstra
{
public static void computePaths(Vertex source)
{
source.minDistance = 0.;
PriorityQueue<Vertex> vertexQueue = new PriorityQueue<Vertex>();
//Set<Vertex> visited = new HashSet<Vertex>();
vertexQueue.add(source);
while (!vertexQueue.isEmpty()) {
Vertex u = vertexQueue.poll();
// Visit each edge exiting u
for (Edge e : u.adjacencies)
{
Vertex v = e.target;
double weight = e.weight;
double distanceThroughU = u.minDistance + weight;
//if (!visited.contains(u)){
if (distanceThroughU < v.minDistance) {
vertexQueue.remove(v);
v.minDistance = distanceThroughU ;
v.previous = u;
vertexQueue.add(v);
visited.add(u)
//}
}
}
}
}
public static List<Vertex> getShortestPathTo(Vertex target)
{
List<Vertex> path = new ArrayList<Vertex>();
for (Vertex vertex = target; vertex != null; vertex = vertex.previous)
path.add(vertex);
Collections.reverse(path);
return path;
}
public static void main(String[] args)
{
Vertex v0 = new Vertex("Redvile");
Vertex v1 = new Vertex("Blueville");
Vertex v2 = new Vertex("Greenville");
Vertex v3 = new Vertex("Orangeville");
Vertex v4 = new Vertex("Purpleville");
v0.adjacencies = new Edge[]{ new Edge(v1, 5),
new Edge(v2, 10),
new Edge(v3, 8) };
v1.adjacencies = new Edge[]{ new Edge(v0, 5),
new Edge(v2, 3),
new Edge(v4, 7) };
v2.adjacencies = new Edge[]{ new Edge(v0, 10),
new Edge(v1, 3) };
v3.adjacencies = new Edge[]{ new Edge(v0, 8),
new Edge(v4, 2) };
v4.adjacencies = new Edge[]{ new Edge(v1, 7),
new Edge(v3, 2) };
Vertex[] vertices = { v0, v1, v2, v3, v4 };
computePaths(v0);
for (Vertex v : vertices)
{
System.out.println("Distance to " + v + ": " + v.minDistance);
List<Vertex> path = getShortestPathTo(v);
System.out.println("Path: " + path);
}
}
}
Dijkstra的算法不需要跟蹤被訪問的頂點,因為它優先考慮具有最短總路徑的那些頂點。
對於未立即連接到起始節點的頂點,當算法啟動時,它們被認為具有無限長的路徑。 一旦訪問了一個頂點,其所有鄰居的總距離都會根據到當前頂點的距離加上兩者之間的旅行成本進行更新。
代碼本來可以更簡單,但無論如何,Djikstra都很貪婪,所以在每個節點,我們都試圖找到最短路徑的節點。 除非存在負邊緣,否則已經訪問過的節點已經填充了最短路徑,因此自然地,條件if(distanceThroughU <v.minDistance)對於訪問節點永遠不會成立。
關於運行時復雜性,兩個實現之間沒有太大區別。
評論行中沒有一行包含負責將Vertex 對象添加到訪問集的代碼。 看起來像:
(!visited.contains(u))
永遠是真的:)
除此之外,您不需要知道訪問過的節點就可以使用算法。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.