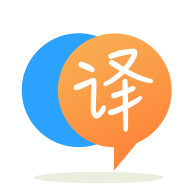
[英]The tiles of my scrolling tile-based platformer aren't loading onto the screen
[英]How to render an isometric tile-based world in Python?
我在使用 Python 和 Pygame 使用以下代碼渲染基於等距 2D 瓷磚的世界時遇到了一些麻煩:
'''
Map Rendering Demo
rendermap.py
By James Walker (trading as Ilmiont Software).
Copyright (C)Ilmiont Software 2013. All rights reserved.
This is a simple program demonstrating rendering a 2D map in Python with Pygame from a list of map data.
Support for isometric or flat view is included.
'''
import pygame
from pygame.locals import *
pygame.init()
DISPLAYSURF = pygame.display.set_mode((640, 480), DOUBLEBUF) #set the display mode, window title and FPS clock
pygame.display.set_caption('Map Rendering Demo')
FPSCLOCK = pygame.time.Clock()
map_data = [
[1, 1, 1, 1, 1],
[1, 0, 0, 0, 1],
[1, 0, 0, 0, 1],
[1, 0, 0, 0, 1],
[1, 0, 0, 0, 1],
[1, 1, 1, 1, 1]
] #the data for the map expressed as [row[tile]].
wall = pygame.image.load('wall.png').convert() #load images
grass = pygame.image.load('grass.png').convert()
tileWidth = 64 #holds the tile width and height
tileHeight = 64
currentRow = 0 #holds the current map row we are working on (y)
currentTile = 0 #holds the current tile we are working on (x)
for row in map_data: #for every row of the map...
for tile in row:
tileImage = wall
cartx = currentTile * 64 #x is the index of the currentTile * the tile width
print(cartx)
carty = currentRow * 64 #y is the index of the currentRow * the tile height
print(carty)
x = cartx - carty
print(x)
y = (cartx + carty) / 2
print(y)
print('\n\n')
currentTile += 1 #increase the currentTile holder so we know that we are starting rendering a new tile in a moment
DISPLAYSURF.blit(tileImage, (x, y)) #display the actual tile
currentTile = 0 #reset the current working tile to 0 (we're starting a new row remember so we need to render the first tile of that row at index 0)
currentRow += 1 #increment the current working row so we know we're starting a new row (used for calculating the y coord for the tile)
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
if event.type == KEYUP:
if event.key == K_ESCAPE:
pygame.quit()
sys.exit()
pygame.display.flip()
FPSCLOCK.tick(30)
使用的圖塊大小為 64x64; 上面的代碼在運行時生成以下輸出: 瓷磚都有透明的邊緣,在這個例子中只有“牆”瓷磚是特色,但顯然有些地方出了問題,因為瓷磚相距太遠。
我試過在線閱讀一些教程,但我似乎找不到真正用 Python 編寫的教程,所以請告訴我我哪里出錯了。
提前致謝, Ilmiont
試試這個:
print(carty)
x = (cartx - carty) / 2
print(x)
y = (cartx + carty)/4*3
並且,經過測試,將convert()修改為convert_alpha(),因為優化殺死了convert()上的alpha
我還修改了 y ( /4*3 ),以考慮您的形象。 這對我有用。
您可以通過將顏色鍵設置為黑色來擺脫黑色三角形,我復制了您的示例並能夠通過更改來修復它:
for row in map_data: #for every row of the map...
for tile in row:
tileImage = wall
到
for row in map:
for tile in row:
tileImage = wall
tileImage.set_colorkey((0,0,0))
來自: https : //www.pygame.org/docs/ref/surface.html#pygame.Surface.set_colorkey
可能有更好的方法來為所有人設置色鍵,但我試過了,它似乎有效。 我看到了關於 alpha 的評論,我相信它也能更好地工作。
關於定位,您只需要從屏幕中間開始渲染。 我可以通過簡單的更改來使用您的代碼執行此操作:
x = cartx - carty
print(x)
y = (cartx + carty) / 2
到
x = 320 + ((cartx - carty) / 2)
y = ((cartx+carty) / 4 * 3)
我希望這對這個線程的任何新人都有幫助!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.