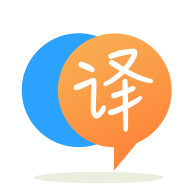
[英]Find longest path less than or equal to given value of an acyclic, directed graph in Python
[英]Shortest and longest path in a topologically sorted unweighted directed acyclic graph using an adjacency matrix (Python, or pseudo-code)
我正在嘗試解決我在做作業時遇到的一個問題,感覺就像是我對算法的思考過多,希望這里的人可以將我推向正確的方向。
我將得到一個輸入txt文件,它看起來像這樣:
1 // n number of graphs
4 // n number of vertices for graph 1
4 // n number of edges for graph 1
1 2 // edges given in pairs
2 3
2 4
3 4
我應該使用此數據來創建代表圖形的n個鄰接矩陣。 然后,我需要對鄰接矩陣中的數據實現3種方法:
我很難很好地實現前兩個部分。 這是我到目前為止的內容:
def main():
numGraphs = input()
for x in xrange(0, numGraphs):
numVerts = input()
numEdges = input()
adjMat = [[0 for x in xrange(numVerts)] for x in xrange(numVerts)]
for x in xrange(0, numEdges):
edges = raw_input()
i, padding, j = edges.rpartition(" ")
i = int(i)
j = int(j)
i -= 1
j -= 1
adjMat[i][j] = 1
numPaths = [0 for x in xrange(numVerts)]
numPaths[0] = 1
longest_path = 1
shortest_path = numVerts
for i in xrange(0, numVerts):
current_path = 0
for j in xrange(0, numVerts):
if adjMat[i][j] == 1:
numPaths[j] += numPaths[i]
current_path += 1
if current_path > longest_path:
longest_path = current_path
if current_path < shortest_path:
shortest_path = current_path
print "shortest: %d, longest: %d, total %d" % (shortest_path, longest_path, numPaths[numVerts-1])
if __name__ == "__main__":
main()
顯然,當它達到0時,shortest_path更新為0,並且不起作用。 另外,將其初始化為0時將不起作用。如果我可以獲得一些偽代碼,或者可能對較長或較短的方法有所幫助,我敢肯定我可以寫相反的東西,或者我可能完全不合時宜。
感謝您的任何投入。
編輯:
所以我想通了。 如果有人遇到類似問題並需要幫助,這是我完成的代碼。
numGraphs = input()
for x in xrange(0, numGraphs):
numVerts = input()
numEdges = input()
adjMat = [[0 for x in xrange(numVerts)] for x in xrange(numVerts)]
for x in xrange(0, numEdges):
edges = raw_input()
i, padding, j = edges.rpartition(" ")
i = int(i)
j = int(j)
i -= 1
j -= 1
adjMat[i][j] = 1
numPaths = [0 for x in xrange(numVerts)]
numPaths[0] = 1
currentPath = [0 for x in xrange(numVerts)]
maxPath = 1
minPath = numVerts -1
for i in xrange(0, numVerts):
for j in xrange(1, numVerts):
if adjMat[i][j] == 1:
numPaths[j] += numPaths[i]
currentPath[j-i] += 1
if (currentPath[j-i] is not 0):
minPath = currentPath[j-i]
maxPath = max(currentPath)
print "shortest: %d, longest: %d, total %d" % (minPath, maxPath, numPaths[numVerts-1])
弄清楚了。 這是我的最終解決方案。
numGraphs = input()
for x in xrange(0, numGraphs):
numVerts = input()
numEdges = input()
adjMat = [[0 for x in xrange(numVerts)] for x in xrange(numVerts)]
for x in xrange(0, numEdges):
edges = raw_input()
i, padding, j = edges.rpartition(" ")
i = int(i)
j = int(j)
i -= 1
j -= 1
adjMat[i][j] = 1
numPaths = [0 for x in xrange(numVerts)]
numPaths[0] = 1
currentPath = [0 for x in xrange(numVerts)]
maxPath = 1
minPath = numVerts -1
for i in xrange(0, numVerts):
for j in xrange(1, numVerts):
if adjMat[i][j] == 1:
numPaths[j] += numPaths[i]
currentPath[j-i] += 1
if (currentPath[j-i] is not 0):
minPath = currentPath[j-i]
maxPath = max(currentPath)
print "shortest: %d, longest: %d, total %d" % (minPath, maxPath, numPaths[numVerts-1])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.