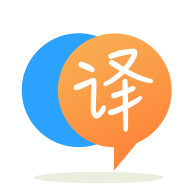
[英]Find longest path less than or equal to given value of an acyclic, directed graph in Python
[英]Shortest and longest path in a topologically sorted unweighted directed acyclic graph using an adjacency matrix (Python, or pseudo-code)
我正在尝试解决我在做作业时遇到的一个问题,感觉就像是我对算法的思考过多,希望这里的人可以将我推向正确的方向。
我将得到一个输入txt文件,它看起来像这样:
1 // n number of graphs
4 // n number of vertices for graph 1
4 // n number of edges for graph 1
1 2 // edges given in pairs
2 3
2 4
3 4
我应该使用此数据来创建代表图形的n个邻接矩阵。 然后,我需要对邻接矩阵中的数据实现3种方法:
我很难很好地实现前两个部分。 这是我到目前为止的内容:
def main():
numGraphs = input()
for x in xrange(0, numGraphs):
numVerts = input()
numEdges = input()
adjMat = [[0 for x in xrange(numVerts)] for x in xrange(numVerts)]
for x in xrange(0, numEdges):
edges = raw_input()
i, padding, j = edges.rpartition(" ")
i = int(i)
j = int(j)
i -= 1
j -= 1
adjMat[i][j] = 1
numPaths = [0 for x in xrange(numVerts)]
numPaths[0] = 1
longest_path = 1
shortest_path = numVerts
for i in xrange(0, numVerts):
current_path = 0
for j in xrange(0, numVerts):
if adjMat[i][j] == 1:
numPaths[j] += numPaths[i]
current_path += 1
if current_path > longest_path:
longest_path = current_path
if current_path < shortest_path:
shortest_path = current_path
print "shortest: %d, longest: %d, total %d" % (shortest_path, longest_path, numPaths[numVerts-1])
if __name__ == "__main__":
main()
显然,当它达到0时,shortest_path更新为0,并且不起作用。 另外,将其初始化为0时将不起作用。如果我可以获得一些伪代码,或者可能对较长或较短的方法有所帮助,我敢肯定我可以写相反的东西,或者我可能完全不合时宜。
感谢您的任何投入。
编辑:
所以我想通了。 如果有人遇到类似问题并需要帮助,这是我完成的代码。
numGraphs = input()
for x in xrange(0, numGraphs):
numVerts = input()
numEdges = input()
adjMat = [[0 for x in xrange(numVerts)] for x in xrange(numVerts)]
for x in xrange(0, numEdges):
edges = raw_input()
i, padding, j = edges.rpartition(" ")
i = int(i)
j = int(j)
i -= 1
j -= 1
adjMat[i][j] = 1
numPaths = [0 for x in xrange(numVerts)]
numPaths[0] = 1
currentPath = [0 for x in xrange(numVerts)]
maxPath = 1
minPath = numVerts -1
for i in xrange(0, numVerts):
for j in xrange(1, numVerts):
if adjMat[i][j] == 1:
numPaths[j] += numPaths[i]
currentPath[j-i] += 1
if (currentPath[j-i] is not 0):
minPath = currentPath[j-i]
maxPath = max(currentPath)
print "shortest: %d, longest: %d, total %d" % (minPath, maxPath, numPaths[numVerts-1])
弄清楚了。 这是我的最终解决方案。
numGraphs = input()
for x in xrange(0, numGraphs):
numVerts = input()
numEdges = input()
adjMat = [[0 for x in xrange(numVerts)] for x in xrange(numVerts)]
for x in xrange(0, numEdges):
edges = raw_input()
i, padding, j = edges.rpartition(" ")
i = int(i)
j = int(j)
i -= 1
j -= 1
adjMat[i][j] = 1
numPaths = [0 for x in xrange(numVerts)]
numPaths[0] = 1
currentPath = [0 for x in xrange(numVerts)]
maxPath = 1
minPath = numVerts -1
for i in xrange(0, numVerts):
for j in xrange(1, numVerts):
if adjMat[i][j] == 1:
numPaths[j] += numPaths[i]
currentPath[j-i] += 1
if (currentPath[j-i] is not 0):
minPath = currentPath[j-i]
maxPath = max(currentPath)
print "shortest: %d, longest: %d, total %d" % (minPath, maxPath, numPaths[numVerts-1])
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.