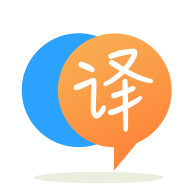
[英]I am having a bit of trouble with this hangman program. Can someone help me figure it out?
[英]I am having trouble creating moving platforms in pygames. I am getting an error, can anyone help me out please?
因此,我剛剛開始學習如何編程,並且一直在嘗試使用移動平台來創建這款小型游戲。 我可以使常規的牆/平台正常工作,但似乎無法弄清楚如何使這些活動的牆/平台正常工作。 我不斷收到如下所示的回溯:
Traceback (most recent call last):
File "C:\Users\Jacob\Desktop\gametest.py", line 227, in <module>
walls.update()
File "C:\Python34\lib\site-packages\pygame\sprite.py", line 462, in update
s.update(*args)
File "C:\Users\Jacob\Desktop\gametest.py", line 111, in update
hit = pygame.sprite.collide_rect(self, self.player)
File "C:\Python34\lib\site-packages\pygame\sprite.py", line 1300, in collide_rect
return left.rect.colliderect(right.rect)
AttributeError: 'NoneType' object has no attribute 'rect'
我認為問題與我擁有的其中一些代碼有關,但我不確定。
class Wall(pygame.sprite.Sprite):
def __init__(self, width, height):
#creates the wall
pygame.sprite.Sprite.__init__(self)
self.image = pygame.Surface([width, height])
self.image.fill(blue)
self.rect = self.image.get_rect()
######################################################
class MovingEnemy(Wall):
change_x = 0
change_y=0
boundary_top = 0
boundary_bottom = 0
boundary_left = 0
boundary_right = 0
player = None
level = None
def update(self):
# Move left/right
self.rect.x += self.change_x
# See if we hit the player
hit = pygame.sprite.collide_rect(self, self.player)
if hit:
# We did hit the player. Shove the player around and
# assume he/she won't hit anything else.
# If we are moving right, set our right side
# to the left side of the item we hit
if self.change_x < 0:
self.player.rect.right = self.rect.left
else:
# Otherwise if we are moving left, do the opposite.
self.player.rect.left = self.rect.right
# Move up/down
self.rect.y += self.change_y
# Check and see if we the player
hit = pygame.sprite.collide_rect(self, self.player)
if hit:
# We did hit the player. Shove the player around and
# assume he/she won't hit anything else.
# Reset our position based on the top/bottom of the object.
if self.change_y < 0:
self.player.rect.bottom = self.rect.top
else:
self.player.rect.top = self.rect.bottom
# Check the boundaries and see if we need to reverse
# direction.
if self.rect.bottom > self.boundary_bottom or self.rect.top < self.boundary_top:
self.change_y *= -1
cur_pos = self.rect.x - self.level.world_shift
if cur_pos < self.boundary_left or cur_pos > self.boundary_right:
self.change_x *= -1
wall = MovingEnemy(70,40)
wall.rect.x = 500
wall.rect.y = 400
wall.boundary_left = 250
wall.boundary_right = 800
wall.change_x = 1
walls.add(wall)
我不確定是否已提供正確的信息以獲取幫助,但我正在誠實地嘗試。 我已經在互聯網上瀏覽了數小時,以尋找一種方法來執行此操作,而我嘗試的所有操作似乎均無效。 如果有人能理解我的這種混亂狀況並幫助我,我將非常感激。
編輯:我確實有一個玩家班級,是否應該將MovingEnemy中的玩家設置為該班級? 我不確定這是否可行,或者我到底應該將其設置為什么。 這是我的球員課,如果這樣做更容易。
class Player(pygame.sprite.Sprite):
#Sets the starting speed
change_x = 0
change_y = 0
walls = None
def __init__(self, x, y):
#creates the sprite for the player
pygame.sprite.Sprite.__init__(self)
#sets the size
self.image = pygame.Surface([25,25])
self.image.fill(green)
self.rect = self.image.get_rect()
self.rect.y = y
self.rect.x = x
def movement(self, x, y):
self.change_x += x
self.change_y += y
def update(self):
#changes the position of the player moving left and right
self.rect.x += self.change_x
#checks to see if the player sprite hits the wall
collision = pygame.sprite.spritecollide(self, self.walls, False)
for block in collision:
# If the player hits a block while moving right, it is set back
# to the left side of the block that was hit.
if self.change_x > 0:
self.rect.right = block.rect.left
# Does the same as above, except with moving left.
else:
self.rect.left = block.rect.right
#changes the position of the player moving up and down
self.rect.y += self.change_y
collision = pygame.sprite.spritecollide(self, self.walls, False)
for block in collision:
# Does the same as the above "for" except for moving up and down
if self.change_y > 0:
self.rect.bottom = block.rect.top
else:
self.rect.top = block.rect.bottom
在MovingEnemy
類中,有一個名為player
的屬性。 player
的在開始時None
。 沒有在代碼中的任何地方將player
更改為除None
任何內容,因此播放器的類型為none或NoneType
。 沒有沒有方法rect
它使用的collide_rect
您調用方法。
您的編譯器告訴您出了什么問題。
walls.update()
首先,您的程序調用wall.update,這是麻煩開始的地方。
hit = pygame.sprite.collide_rect(self, self.player)
然后,您嘗試檢測牆壁和播放器之間的碰撞檢測。
File "C:\Python34\lib\site-packages\pygame\sprite.py", line 1300, in collide_rect
return left.rect.colliderect(right.rect)
請注意,這條線是下一條線索,因為這最終是引發問題的那條線,它是這樣說的:
AttributeError: 'NoneType' object has no attribute 'rect'
您的牆(self)或(self.player)的類型均為None,因此沒有屬性“ rect”可用來確定碰撞。 現在注意:
player = None
您從未設置過玩家! 因此,玩家的類型為None,並且沒有屬性“ rect”來進行碰撞檢測。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.