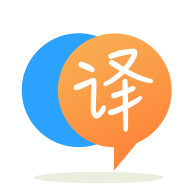
[英]How to 'clone' an incoming ASP.NET MVC Multipart request to send to a Web API controller
[英]Reading every incoming request (URL) in ASP.NET WEB API
我使用的是ASP.NET MVC
框架。 在此框架中,我們檢查了某些密鑰的每個傳入請求(url)並將其分配給屬性。 我們創建了一個派生自Controller
類的自定義類,我們重寫 OnActionExecuting()
以提供我們的自定義邏輯。
我們如何在ASP.NET WEB API中實現相同的目標?
//Implementation from ASP.NET MVC
public class ApplicationController : Controller
{
public string UserID { get; set; }
protected override void OnActionExecuting(ActionExecutingContext filterContext)
{
if (!string.IsNullOrEmpty(Request.Params["uid"]))
UserID = Request.Params["uid"];
base.OnActionExecuting(filterContext);
}
}
我在ASP.NET WEB API中嘗試過的: - 雖然這是有效的,但我想知道這是否是正確的方法?
創建了一個基本控制器
public class BaseApiController : ApiController
{
public string UserID { get; set; }
}
創建了另一個繼承ActionFilterAttribute類的類,我重寫了OnActionExecuting()
public class TokenFilterAttribute : ActionFilterAttribute
{
public override void OnActionExecuting(System.Web.Http.Controllers.HttpActionContext actionContext)
{
var queryString = actionContext.Request.RequestUri.Query;
var items = HttpUtility.ParseQueryString(queryString);
var userId = items["uid"];
((MyApi.Data.Controllers.BaseApiController)(actionContext.ControllerContext.Controller)).UserID = userId;
}
}
現在注冊這個課程
public static void Register(HttpConfiguration config)
{
config.Filters.Add(new TokenFilterAttribute());
}
您可以使用ASP.NET Web API中的消息處理程序。 當您需要從查詢字符串,URL或HTTP標頭獲取一些用戶令牌時,這是一種典型的安全方案
http://www.asp.net/web-api/overview/advanced/http-message-handlers
1.當您只需從URL中提取userId時,請將其用作Api方法的參數,ASP.NET WebAPI將為您工作,如
[HttpGet, Route("{userId}/roles")]
public UserRoles GetUserRoles(string userId, [FromUri] bool isExternalUser = true)
它適用於此類請求
http://.../15222/roles?isExternalUser=false
2.如果是安全方案,請參考http://www.asp.net/web-api/overview/security/authentication-and-authorization-in-aspnet-web-api基本上你需要一些MessageHandler或者你也可以使用過濾器屬性,它是ASP.NET Web API中攔截每個調用的機制。
如果您需要處理每個請求,那么MessageHandler就是您的方式。 您需要實現MessageHanler然后注冊它。
簡單地說,典型的MessageHandler是從MessageHandler派生的類或者使用SendAsync方法覆蓋的DelegatingHandler:
class AuthenticationHandler : DelegatingHandler
{
protected override Task<HttpResponseMessage> SendAsync(HttpRequestMessage request, CancellationToken cancellationToken)
{
// Your code here
return base.SendAsync(request, cancellationToken);
}
}
And you need register it
static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Other code for WebAPI registerations here
config.MessageHandlers.Add(new AuthenticationHandler());
}
}
並從Global.asax.cs調用它
WebApiConfig.Register(GlobalConfiguration.Configuration);
這種處理程序的虛擬hypotetical實現的一些示例(這里你需要從IPrincipal和IIdentity的UidIdentity中補充你的UidPrincipal)
public class AuthenticationHandler : DelegatingHandler
{
protected override Task<HttpResponseMessage> SendAsync(HttpRequestMessage request, CancellationToken cancellationToken)
{
try
{
var queryString = actionContext.Request.RequestUri.Query;
var items = HttpUtility.ParseQueryString(queryString);
var userId = items["uid"];
// Here check your UID and maybe some token, just dummy logic
if (userId == "D8CD2165-52C0-41E1-937F-054F24266B65")
{
IPrincipal principal = new UidPrincipal(new UidIdentity(uid), null);
// HttpContext exist only when hosting as asp.net web application in IIS or IISExpress
if (HttpContext.Current != null)
{
HttpContext.Current.User = principal;
}
else
{
Thread.CurrentPrincipal = principal;
}
return base.SendAsync(request, cancellationToken);
}
catch (Exception ex)
{
this.Log().Warn(ex.ToString());
return this.SendUnauthorizedResponse(ex.Message);
}
}
else
{
return this.SendUnauthorizedResponse();
}
}
catch (SecurityTokenValidationException)
{
return this.SendUnauthorizedResponse();
}
}
}
並允許從一些ASP.NET WebApi方法或WebAPI類中的某些屬性訪問它
var uid = ((UidIdentity)User.Identity).Uid
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.