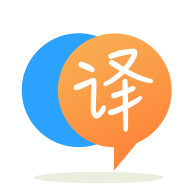
[英]LWP::UserAgent for XML POST to HTTPS server not working
[英]Submit HTTP POST request using LWP::UserAgent giving XML file contents as body
#!/usr/bin/perl -w
use strict;
use FileHandle;
use LWP::UserAgent;
use HTTP::Request;
sub printFile($) {
my $fileHandle = $_[0];
while (<$fileHandle>) {
my $line = $_;
chomp($line);
print "$line\n";
}
}
my $message = new FileHandle;
open $message, '<', 'Request.xml' or die "Could not open file\n";
printFile($message);
my $url = qq{https://host:8444};
my $ua = new LWP::UserAgent(ssl_opts => { verify_hostname => 0 });
$ua->proxy('http', 'proxy:8080');
$ua->no_proxy('localhost');
my $req = new HTTP::Request(POST => $url);
$req->header('Host' => "host:8444");
$req->content_type("application/xml; charset=utf-8");
$req->content($message);
$req->authorization_basic('TransportUser', 'TransportUser');
my $response = $ua->request($req);
my $content = $response->decoded_content();
print $content;
我收到以下錯誤。
我想使用LWP::UserAgent
提交發布請求,並且想給出XML文件的位置作為正文。 我收到無效的請求正文錯誤。 請求正文無效
我不了解printFile
的用途,但是您正在將文件句柄 $message
作為消息正文而不是文件內容進行傳遞。
請注意以下幾點
始終 use warnings
而不是-w
注釋行選項
切勿使用子例程原型。 sub printFile($)
應該只是sub printFile
無需use FileHandle
處理文件
之所以一個文件open
失敗是$!
。 您應該始終將其包含在die
字符串中
切勿使用間接對象符號。 new LWP::UserAgent
應該是LWP::UserAgent->new
此版本的代碼可能會更好一些,但我無法對其進行測試
#!/usr/bin/perl
use strict;
use warnings;
use LWP;
my $message = do {
open my $fh, '<', 'Request.xml' or die "Could not open file: $!";
local $/;
<$fh>;
};
my $url = 'https://host:8444';
my $ua = LWP::UserAgent->new(ssl_opts => { verify_hostname => 0 });
$ua->proxy(qw/ http proxy:8080 /);
$ua->no_proxy('localhost');
my $req = HTTP::Request->new(POST => $url);
$req->header(Host => 'host:8444');
$req->content_type('application/xml; charset=utf-8');
$req->content($message);
$req->authorization_basic('TransportUser', 'TransportUser');
my $response = $ua->request($req);
my $content = $response->decoded_content;
print $content;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.