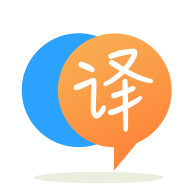
[英]How to write custom serializer for entity with MobogDB C# Driver?
[英]how to write Custom JSon serializer in C#
我正在使用Newtonsoft json序列化程序將json字符串轉換為對象。 我有如下的json結構-
{
"EmployeeRecords": {
"12": {
"title": "Mr",
"Name": "John"
},
"35":{
"title": "Mr",
"Name": "Json"
}
}
}
我希望將此Json放到下面的課程中-
public class Employee
{
public string EmployeeNumber { get; set; }
public string title { get; set; }
public string Name { get; set; }
}
public class EmployeeRecords
{
public List<Employee> Employees { get; set; }
}
此處Employeenumber是12和35。
請指導我如何編寫自定義序列化程序,該序列化程序將從父節點讀取Employee編號並將其包括在子節點的EmployeeNumber屬性中。
您可以反序列化為字典,然后在循環中分配EmployeeNumbers。
public class DataModel
{
public Dictionary<string, Employee> EmployeeRecords { get; set; }
}
反序列化后評估數字:
var records = JsonConvert.DeserializeObject<DataModel>(json);
foreach (var item in records.EmployeeRecords)
{
item.Value.EmployeeNumber = item.Key;
}
您可以使用LINQ to JSON( JObject
和朋友)輕松地做到這一點。 這是一個簡短但完整的示例:
using System;
using System.Collections.Generic;
using Newtonsoft.Json.Linq;
public class Employee
{
public string EmployeeNumber { get; set; }
public string title { get; set; }
public string Name { get; set; }
public JProperty ToJProperty()
{
return new JProperty(EmployeeNumber,
new JObject {
{ "title", title },
{ "Name", Name }
});
}
}
public class EmployeeRecords
{
public List<Employee> Employees { get; set; }
public JObject ToJObject()
{
var obj = new JObject();
foreach (var employee in Employees)
{
obj.Add(employee.ToJProperty());
}
return new JObject {
new JProperty("EmployeeRecords", obj)
};
}
}
class Test
{
static void Main()
{
var records = new EmployeeRecords {
Employees = new List<Employee> {
new Employee {
EmployeeNumber = "12",
title = "Mr",
Name = "John"
},
new Employee {
EmployeeNumber = "35",
title = "Mr",
Name = "Json"
},
}
};
Console.WriteLine(records.ToJObject());
}
}
它可能不是最簡單的代碼(如果您樂於更改結構,Ufuk的方法非常有用),但是它顯示了所有內容的可定制性。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.