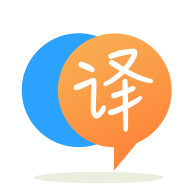
[英]Best way to rotate a 3D grid (nxnxn) of values in Python with interpolation?
[英]rotate an nxnxn matrix in python
我有一個大小為 64x64x64 的二進制數組,其中 40x40x40 的體積設置為“1”,其余為“0”。 我一直在嘗試使用skimage.transform.rotate
和 Opencv 圍繞 z 軸的中心旋轉這個立方體:
def rotateImage(image, angle):
row, col = image.shape
center = tuple(np.array([row, col]) / 2)
rot_mat = cv2.getRotationMatrix2D(center, angle, 1.0)
new_image = cv2.warpAffine(image, rot_mat, (col, row))
return new_image
在 openCV 的情況下,我嘗試對立方體中的每個單獨切片進行 2D 旋轉(Cube[:,:,n=1,2,3...p])。
旋轉后,數組中值的總和發生變化。 這可能是由旋轉期間的插值引起的。 如何在不向陣列添加任何內容的情況下旋轉這種 3D 陣列?
好的,我現在明白你在問什么。 我能想到的最接近的是 scipy.ndimage。 但是有一種方法可以與 python 中的 imagej 接口,如果這可能更容易的話。 但這是我對 scipy.ndimage 所做的:
from scipy.ndimage import interpolation
angle = 25 #angle should be in degrees
Rotatedim = interpolation.rotate(yourimage, angle, reshape = False,output = np.int32, order = 5,prefilter = False)
這適用於某些角度以保留某些角度而不是其他角度,也許通過更多地使用參數,您可能能夠獲得所需的結果。
一種選擇是轉換為稀疏的,並使用矩陣旋轉變換坐標。 然后變回稠密。 在二維中,這看起來像:
import numpy as np
import scipy.sparse
import math
N = 10
space = np.zeros((N, N), dtype=np.int8)
space[3:7, 3:7].fill(1)
print(space)
print(np.sum(space))
space_coo = scipy.sparse.coo_matrix(space)
Coords = np.array(space_coo.nonzero()) - 3
theta = 30 * 3.1416 / 180
R = np.array([[math.cos(theta), math.sin(theta)], [-math.sin(theta), math.cos(theta)]])
space2_coords = R.dot(Coords)
space2_coords = np.round(space2_coords)
space2_coords += 3
space2_sparse = scipy.sparse.coo_matrix(([1] * space2_coords.shape[1], (space2_coords[0], space2_coords[1])), shape=(N, N))
space2 = space2_sparse.todense()
print(space2)
print(np.sum(space2))
輸出:
[[0 0 0 0 0 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]
[0 0 0 1 1 1 1 0 0 0]
[0 0 0 1 1 1 1 0 0 0]
[0 0 0 1 1 1 1 0 0 0]
[0 0 0 1 1 1 1 0 0 0]
[0 0 0 0 0 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]]
16
[[0 0 0 0 0 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]
[0 0 0 1 0 0 0 0 0 0]
[0 0 1 1 1 1 0 0 0 0]
[0 0 1 1 1 1 1 0 0 0]
[0 1 1 0 1 1 0 0 0 0]
[0 0 0 1 1 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]
[0 0 0 0 0 0 0 0 0 0]]
16
優點是您將在轉換之前和之后獲得完全相同的1
值。 缺點是您可能會得到“洞”,如上所述,和/或重復坐標,在最終密集矩陣中給出“2”值。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.