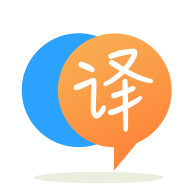
[英]StrokeThickness of Line is not working properly when we draw the line at the right end of the Grid WPF
[英]Draw line with high StrokeThickness in silverlight
我有一個應用程序,您可以在畫布上繪制(如“畫圖”)。 C#代碼基本上如下所示:
private void startDrawing(object sender, System.Windows.Input.MouseButtonEventArgs e)
{
_drawingStart = e.GetPosition((UIElement)sender);
_isDrawing = true;
}
private void stopDrawing(object sender, System.Windows.Input.MouseButtonEventArgs e)
{
_isDrawing = false;
}
private void doDrawing(object sender, System.Windows.Input.MouseEventArgs e)
{
if (_isDrawing)
{
Point current = e.GetPosition((UIElement)sender);
Line line = new Line() { X1 = _drawingStart.X, Y1 = _drawingStart.Y, X2 = current.X, Y2 = current.Y };
line.Stroke = Color;
line.StrokeThickness = StrokeThickness;
DrawingCanvas.Children.Add(line);
_drawingStart = current;
}
}
和畫布:
<Canvas x:Name="DrawingCanvas"
Grid.Row="1"
Grid.Column="1"
Background="Transparent"
MouseLeftButtonDown="startDrawing"
MouseLeftButtonUp="stopDrawing"
MouseMove="doDrawing" />
當StrokeThickness較小時,一切正常。 但是,如果將StrokeThickness設置為較大的數字(例如100),則該線以“ zig-zag”樣式繪制,而不是“實心”。 有什么想法,如何避免這種情況? 或者也許如何實現圓角線(圓角線的兩端)? 我認為這可以解決問題。
您應該將Line的StrokeLineJoin
屬性設置為Bevel
或Round
。
var line = new Line
{
X1 = _drawingStart.X,
Y1 = _drawingStart.Y,
X2 = current.X,
Y2 = current.Y,
Stroke = Color,
StrokeThickness = StrokeThickness,
StrokeLineJoin = PenLineJoin.Round
};
有關詳細信息,請參見MSDN上的PenLineJoin枚舉頁面。
或者,您可以將StrokeMiterLimit
設置為合適的值。
也就是說,一個更優雅的解決方案是將點添加到折線的“ Points
集合中:
private Polyline currentPolyline;
private void startDrawing(object sender, MouseButtonEventArgs e)
{
var canvas = (Canvas)sender;
currentPolyline = new Polyline
{
Stroke = Color,
StrokeThickness = StrokeThickness,
StrokeStartLineCap = PenLineCap.Round,
StrokeEndLineCap = PenLineCap.Round,
StrokeLineJoin = PenLineJoin.Round
};
currentPolyline.Points.Add(e.GetPosition(canvas));
canvas.Children.Add(currentPolyline);
}
private void stopDrawing(object sender, MouseButtonEventArgs e)
{
currentPolyline = null;
}
private void doDrawing(object sender, MouseEventArgs e)
{
if (currentPolyline != null)
{
currentPolyline.Points.Add(e.GetPosition((UIElement)sender));
}
}
我認為您將對我的回答着迷:
Point _drawingStart;
bool _isDrawing;
private void startDrawing(object sender, System.Windows.Input.MouseButtonEventArgs e)
{
_drawingStart = e.GetPosition((UIElement)sender);
InitializePath();
_isDrawing = true;
}
private void stopDrawing(object sender, System.Windows.Input.MouseButtonEventArgs e)
{
_isDrawing = false;
}
private void doDrawing(object sender, System.Windows.Input.MouseEventArgs e)
{
if (_isDrawing)
{
AddPoint(e.GetPosition((UIElement)sender));
}
}
private void AddPoint(Point newpoint)
{
LineSegment l = new LineSegment() { Point = newpoint };
pathFigure.Segments.Add(l);
pathFigure.StartPoint = (pathFigure.Segments.First() as LineSegment).Point;
}
PathFigure pathFigure;
Path path;
private void InitializePath()
{
path = new Path()
{
StrokeLineJoin = PenLineJoin.Bevel,
StrokeDashCap = PenLineCap.Round,
StrokeEndLineCap = PenLineCap.Round,
StrokeStartLineCap = PenLineCap.Round,
StrokeThickness = 100.0,
Stroke = new SolidColorBrush(Colors.Red)
};
pathFigure = new PathFigure();
PathGeometry pathGeometry = new PathGeometry();
pathGeometry.Figures = new PathFigureCollection();
pathGeometry.Figures.Add(pathFigure);
path.Data = pathGeometry;
DrawingCanvas.Children.Add(path);
}
更平滑,因為它創建的是真實路徑而不是許多行,希望您發現它有用
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.