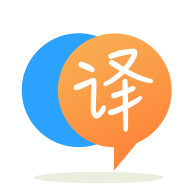
[英]I have a list that contains dictionaries. How can i append to a specific key's value?
[英]I have a list of tuples containing dictionaries. How do I edit my code to find the values in a separate list, inside these dictionaries?
我有一個數據結構L = [(int,dict {key:values}),(int,dict {key:values})...]。
給定輸入列表[0,1]我想找到任何字典鍵,其中存在輸入列表[0,1]的兩個/所有值。
目前,我的錯誤是,如果我使用input_list = [0,1],該函數將返回一個匹配,其中字典值只是[0],其中值是[0,1]。 只有這第二個結果是可取的。 我覺得這是一個微小的變化,但我無法理解。 為了實現這一目標,我該怎么做?
碼
#Python3
L = [(0, {0: [0], 1: [0, 1], 2: [0, 2], 3: [0, 3], 4: [0, 4]}), (1, {0: [1, 0], 1: [1], 2: [5, 1, 2,], 3: [1, 3], 4: [1, 4]}), (2, {0: [2, 0], 1: [2, 1], 2: [2], 3: [2, 3], 4: [2, 4]}), (3, {0: [3, 0], 1: [3, 1], 2: [3, 2], 3: [3], 4: [3, 4]}), (4, {0: [4, 0], 1: [4, 1], 2: [4, 2], 3: [4, 3], 4: [4]})]
#input_list = (eval(input('Enter your list: ')))
#input_list = ([0,1])
print('Input: ' + str(input_list))
for tupl in L:
dict_a = (tupl[1])
matching_key = ([key for key, value in dict_a.items() if all(v in input_list for v in value)])
print('Node: ' + str(tupl[0]) + ' Match at key(s): ' + str(matching_key))
產量
L = [(0, {0: [0], 1: [0, 1], 2: [0, 2], 3: [0, 3], 4: [0, 4]}), (1, {0: [1, 0], 1: [1], 2: [5, 1, 2,], 3: [1, 3], 4: [1, 4]}), (2, {0: [2, 0], 1: [2, 1], 2: [2], 3: [2, 3], 4: [2, 4]}), (3, {0: [3, 0], 1: [3, 1], 2: [3, 2], 3: [3], 4: [3, 4]}), (4, {0: [4, 0], 1: [4, 1], 2: [4, 2], 3: [4, 3], 4: [4]})]
Enter your list: [0,1]
Input: [0, 1]
Node: 0 Match at key(s): [0, 1]
Node: 1 Match at key(s): [0, 1]
Node: 2 Match at key(s): []
Node: 3 Match at key(s): []
Node: 4 Match at key(s): []
Enter your list: [1,5,2]
Input: [1, 5, 2]
Node: 0 Match at key(s): []
Node: 1 Match at key(s): [1, 2]
Node: 2 Match at key(s): [1, 2]
Node: 3 Match at key(s): []
Node: 4 Match at key(s): []
謝謝:)
你有代碼檢查value
包含input_list
的所有項目。 all(v in input_list for v in value)
檢查可以從input_list
找到value
中的所有項。 如果你改變它,反過來它將按預期工作:
all(v in value for v in input_list)
請注意,如果要將input_list
轉換為set
,則可以輕松檢查input_list
是否為value
的子集。 這將更容易理解,更有效:
L = [(0, {0: [0], 1: [0, 1], 2: [0, 2], 3: [0, 3], 4: [0, 4]}), (1, {0: [1, 0], 1: [1], 2: [5, 1, 2,], 3: [1, 3], 4: [1, 4]}), (2, {0: [2, 0], 1: [2, 1], 2: [2], 3: [2, 3], 4: [2, 4]}), (3, {0: [3, 0], 1: [3, 1], 2: [3, 2], 3: [3], 4: [3, 4]}), (4, {0: [4, 0], 1: [4, 1], 2: [4, 2], 3: [4, 3], 4: [4]})]
input_list = set([0,1])
for tupl in L:
dict_a = tupl[1]
matching_key = [key for key, value in dict_a.items() if input_list <= set(value)]
print('Node: ' + str(tupl[0]) + ' Match at key(s): ' + str(matching_key))
輸出:
Node: 0 Match at key(s): [1]
Node: 1 Match at key(s): [0]
Node: 2 Match at key(s): []
Node: 3 Match at key(s): []
Node: 4 Match at key(s): []
您可以使用set subtraction來解決:
#Python3
L = [(0, {0: [0], 1: [0, 1], 2: [0, 2], 3: [0, 3], 4: [0, 4]}), (1, {0: [1, 0], 1: [1], 2: [5, 1, 2,], 3: [1, 3], 4: [1, 4]}), (2, {0: [2, 0], 1: [2, 1], 2: [2], 3: [2, 3], 4: [2, 4]}), (3, {0: [3, 0], 1: [3, 1], 2: [3, 2], 3: [3], 4: [3, 4]}), (4, {0: [4, 0], 1: [4, 1], 2: [4, 2], 3: [4, 3], 4: [4]})]
input_list = (eval(input('Enter your list: ')))
#input_list = [1,5,2]
print('Input: ' + str(input_list))
for tupl in L:
dict_a = (tupl[1])
matching_key = []
for key, lst in tupl[1].items():
if not (set(lst) - set(input_list)):
matching_key.append(key)
print('Node: ' + str(tupl[0]) + ' Match at key(s): ' + str(matching_key))
我建議你避免像這樣的構造[key for key, value in dict_a.items() if all(v in input_list for v in value)]
因為它混淆了,它使你的代碼難以理解。
請閱讀:
檢查兩個無序列表是否相等 , 如何在python中比較兩個有序列表?
根據您在檢查相等性時要使用的語義,可以使用一種解決方案或另一種解決方案。 如果你想進行簡單的有序列表比較,我會選擇:
for tupl in L:
dict_a = (tupl[1])
if dict_a != input_list:
continue
print('Node: ' + str(tupl[0]) + ' Match at key(s): ' + str(matching_key))
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.