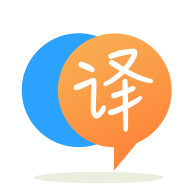
[英]How to map a linear index to 2D index for array of list in python?
[英]How to use a linear index to access a 2D array in Python
我在 MATLAB 中有一段代碼,我嘗試將該代碼轉換為 Python。 在 MATLAB 中,我可以這樣寫:
x = [1,2,3;4,5,6;7,8,9];
這只是一個 3*3 矩陣。 然后,如果我使用x(1:5)
,MATLAB 將首先將矩陣x
轉換為 1*9 向量,然后返回一個 1*5 向量,如下所示: ans=[1,4,7,2,5];
那么你能告訴我python中哪一段簡單的代碼可以產生相同的結果嗎?
您可以將矩陣轉換為numpy
數組,然后使用unravel_index
將線性索引轉換為下標,然后可以使用這些下標索引到原始矩陣中。 請注意,下面的所有命令都使用'F'
輸入來使用列主要排序(MATLAB的默認值)而不是行主要排序( numpy
的默認值)
import numpy as np
a = np.array([[1,2,3],[4,5,6],[7,8,9]])
inds = np.arange(5);
result = a[np.unravel_index(inds, a.shape, 'F')]
# array([1, 4, 7, 2, 5])
另外,如果你想要像MATLAB一樣壓縮矩陣,你也可以這樣做:
a.flatten('F')
# array([1, 4, 7, 2, 5, 8, 3, 6, 9])
如果你要將一堆MATLAB代碼轉換為python,強烈建議使用numpy
並查看有關顯着差異的文檔
我不確定MATLAB的x(1:5)
語法應該做什么,但根據你想要的輸出,它似乎是轉置矩陣,展平它,然后返回一個切片。 這是在Python中如何做到這一點:
>>> from itertools import chain
>>>
>>> x = [[1,2,3],
... [4,5,6],
... [7,8,9]]
>>>
>>> list(chain(*zip(*x)))[0:5]
[1, 4, 7, 2, 5]
直接訪問二維數組而不進行轉換副本的另一種方法是使用整數除法和模運算符。
import numpy as np
# example array
rect_arr = np.array([[1, 2, 3, 10], [4, 5, 6, 11], [7, 8, 9, 12]])
rows, cols = rect_arr.shape
print("Array is:\n", rect_arr)
print(f"rows = {rows}, cols = {cols}")
# Access by Linear Indexing
# Reference:
# https://upload.wikimedia.org/wikipedia/commons/4/4d/Row_and_column_major_order.svg
total_elems = rect_arr.size
# Row major order
print("\nRow Major Sequence:")
for linear_index in range(total_elems):
# do something with rect_arr[linear_index // cols][linear_index % cols]
# Sequence will be 1, 2, 3, 10, 4, 5, 6, 11, 7, 8, 9, 12
print(rect_arr[linear_index // cols][linear_index % cols])
# Columnn major order
print("\nColumn Major Sequence:")
for linear_index in range(total_elems):
# do something with rect_arr[linear_index % rows][linear_index // rows]
# Sequence will be 1, 4, 7, 2, 5, 8, 3, 6, 9, 10, 11, 12
print(rect_arr[linear_index % rows][linear_index // rows])
# With unravel_index
# Row major order
row_indices = range(total_elems)
row_transformed_arr = rect_arr[np.unravel_index(row_indices, rect_arr.shape, "C")]
print(row_transformed_arr)
# Columnn major order
col_indices = range(total_elems)
col_transformed_arr = rect_arr[np.unravel_index(row_indices, rect_arr.shape, "F")]
print(col_transformed_arr)
用於在子圖中繪圖:
# <df> is a date-indexed dataframe with 8 columns containing time-series data
fig, axs = plt.subplots(nrows=4, ncols=2)
rows, cols = axs.shape
# Order plots in row-major
for i, colname in enumerate(df):
df[colname].plot(ax=axs[i // cols][i % cols], title=colname)
plt.show()
# Order plots in column-major
for i, colname in enumerate(df):
df[colname].plot(ax=axs[i % rows][i // rows], title=colname)
plt.show()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.