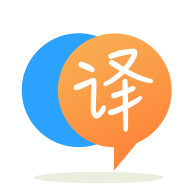
[英]How to find the center of black objects in an image with python opencv?
[英]How to find the center and angle of objects in an image?
我正在使用python和OpenCV。 我試圖找到電池的中心和角度:
比我擁有的代碼是這樣的:
import cv2
import numpy as np
img = cv2.imread('image/baterias2.png')
gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
img2 = cv2.imread('image/baterias4.png',0)
minLineLength = 300
maxLineGap = 5
edges = cv2.Canny(img2,50,200)
cv2.imshow('Canny',edges)
lines = cv2.HoughLinesP(edges,1,np.pi/180,80,minLineLength,maxLineGap)
print lines
salida = np.zeros((img.shape[0],img.shape[1]))
for x in range(0, len(lines)):
for x1,y1,x2,y2 in lines[x]:
cv2.line(salida,(x1,y1),(x2,y2),(125,125,125),0)# rgb
cv2.imshow('final',salida)
cv2.imwrite('result/hough.jpg',img)
cv2.waitKey(0)
有什么想法要解決嗎?
幾乎和我的其他答案之一相同。 PCA似乎工作正常。
import cv2
import numpy as np
img = cv2.imread("test_images/battery001.png") #load an image of a single battery
img_gs = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) #convert to grayscale
#inverted binary threshold: 1 for the battery, 0 for the background
_, thresh = cv2.threshold(img_gs, 250, 1, cv2.THRESH_BINARY_INV)
#From a matrix of pixels to a matrix of coordinates of non-black points.
#(note: mind the col/row order, pixels are accessed as [row, col]
#but when we draw, it's (x, y), so have to swap here or there)
mat = np.argwhere(thresh != 0)
#let's swap here... (e. g. [[row, col], ...] to [[col, row], ...])
mat[:, [0, 1]] = mat[:, [1, 0]]
#or we could've swapped at the end, when drawing
#(e. g. center[0], center[1] = center[1], center[0], same for endpoint1 and endpoint2),
#probably better performance-wise
mat = np.array(mat).astype(np.float32) #have to convert type for PCA
#mean (e. g. the geometrical center)
#and eigenvectors (e. g. directions of principal components)
m, e = cv2.PCACompute(mat, mean = np.array([]))
#now to draw: let's scale our primary axis by 100,
#and the secondary by 50
center = tuple(m[0])
endpoint1 = tuple(m[0] + e[0]*100)
endpoint2 = tuple(m[0] + e[1]*50)
red_color = (0, 0, 255)
cv2.circle(img, center, 5, red_color)
cv2.line(img, center, endpoint1, red_color)
cv2.line(img, center, endpoint2, red_color)
cv2.imwrite("out.png", img)
您可以參考代碼。
import cv2
import imutils
import numpy as np
PIC_PATH = r"E:\temp\Battery.jpg"
image = cv2.imread(PIC_PATH)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
edged = cv2.Canny(gray, 100, 220)
kernel = np.ones((5,5),np.uint8)
closed = cv2.morphologyEx(edged, cv2.MORPH_CLOSE, kernel)
cnts = cv2.findContours(closed.copy(), cv2.RETR_EXTERNAL,
cv2.CHAIN_APPROX_SIMPLE)
cnts = cnts[0] if imutils.is_cv2() else cnts[1]
cv2.drawContours(image, cnts, -1, (0, 255, 0), 4)
cv2.imshow("Output", image)
cv2.waitKey(0)
結果圖片是
要找出對象的中心,可以使用Moments 。 閾值圖像並使用findContours獲取對象的輪廓。 用cv.Moments(arr, binary=0) → moments
。 作為arr
您可以傳遞輪廓。 然后,將中心坐標計算為x = m10/m00
和y = m01/m00
。
要獲得方向,可以在對象周圍繪制最小的Rectangle並計算矩形的較長邊和垂直線之間的角度。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.