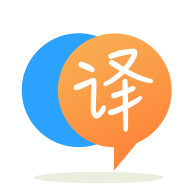
[英]How can I declare an abstract generic class that inherits from another abstract class?
[英]How do I make a variable that requires a type of a class that inherits an abstract?
我希望變量只包含繼承特定抽象的類的類型。 在此示例中,變量“MyLetter”應該只包含LetterA,LetterB或繼承LetterAbstract的任何類型。
我怎么做到這一點?
abstract class LetterAbstract
{
public char Letter;
}
class LetterA : LetterAbstract
{
void LetterA()
{
Letter = 'A';
}
}
// valid
TLetterAbstract MyLetter = typeof(LetterA);
// invalid
TLetterAbstract MyLetter = typeof(string);
在這種情況下,您需要使用數據類型LetterAbstract
(所謂的基類)聲明變量。
LetterAbstract letter;
letter = new LetterA(); // valid
letter = "asdasd"; // invalid
我想你首先需要閱讀有關繼承的內容,參見“ 繼承(C#編程指南) ”。
:編輯
關於
我不想要LetterA的實例。 我想要類型LetterA。
你可以用泛型來搗亂一些東西,但我不知道用例可能是什么:
class TypeVar<A>
where A: LetterAbstract
{
public void SetValue<B>()
where B: A
{
mValue = typeof(B);
}
public System.Type GetValue()
{
return mValue;
}
private System.Type mValue;
}
var x =new TypeVar<LetterAbstract>();
x.SetValue<LetterA>(); // valid
x.SetValue<string>(); // invalid
x.GetValue(); // get the type
我不想要LetterA的實例。 我想要類型LetterA
雖然你想要的東西對我來說似乎並不清楚。 嘗試這個。
請注意,如果要限制編碼,則除非您聲明變量Letter
否則無法進行編碼,但您可以確定變量是否是某種類型的子類。
static bool IsLetter(object obj)
{
Type type = obj.GetType();
return type.IsSubclassOf(typeof(Letter)) || type == typeof(Letter);
}
樣品:
class Foo
{
}
abstract class Letter
{
}
class LetterA : Letter
{
}
class LetterAA : LetterA
{
}
class LetterAB : LetterA
{
}
用法:
LetterAA aa = new LetterAA();
LetterAB ab = new LetterAB();
Foo foo = new Foo();
bool isAA = IsLetter(aa);
bool isAB = IsLetter(ab);
bool isLetter = IsLetter(foo);
Console.WriteLine(isAA); // True
Console.WriteLine(isAB); // True
Console.WriteLine(isLetter); // False
您是否考慮過TLetterAbstract
類? 您可以將其定義為:
public class TLetterAbstract : Type
{
private Type _T;
public TLetterAbstract(object o)
{
if (o is LetterAbstract)
{
_T = o.GetType();
}
throw new ArgumentException("Wrong input type");
}
public TLetterAbstract(Type t)
{
if (t.IsInstanceOfType(typeof(LetterAbstract)))
{
_T = t;
}
throw new ArgumentException("Wrong input type");
}
public override object[] GetCustomAttributes(bool inherit)
{
return _T.GetCustomAttributes(inherit);
}
// and some more abstract members of Type implemented by simply forwarding to the instance
}
然后你必須改變你的代碼
TLetterAbstract MyLetter = typeof(LetterA);
至
TLetterAbstract MyLetter = new TLetterAbstract(typeof(LetterA));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.