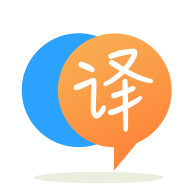
[英]Best way to perform multiple amount of Pandas lookups between two DataFrames
[英]Fastest way to perform string lookups?
假設我們有一定數量的可能字符串:
possible_strings_list = ['foo', 'bar', 'baz', 'qux', 'spam', 'ham', 'eggs']
並接收已知是其中之一的新字符串。 例如,我們要為每個新字符串分配一個整數
if new_string == 'foo':
return 0
elif new_string == 'bar':
return 1
...
在Python 3.6中最快的方法是什么? 我嘗試了幾種方法,到目前為止,使用字典是最快的:
list_index 2.7494255019701086
dictionary 0.9412809460191056
if_elif_else 2.10705983400112
lambda_function 2.6321219780365936
tupple_index 2.751029207953252
ternary 1.931659944995772
np_where 15.610908019007184
但是,我或多或少是Python新手,如果有其他更快的解決方案,我很感興趣。 你有什么建議嗎?
我完整的testig代碼:
import timeit
import random
import numpy as np
def list_index(i):
return(possible_strings_list.index(i))
def dictionary(i):
return possible_strings_dict[i]
def tupple_index(i):
return possible_strings_tup.index(i)
def if_elif_else(i):
if i == 'foo':
return 1
elif i == 'bar':
return 2
elif i == 'baz':
return 3
elif i == 'qux':
return 4
elif i == 'spam':
return 5
elif i == 'ham':
return 6
elif i == 'eggs':
return 7
def ternary(i):
return 0 if i == 'foo' else 1 if i == 'baz' else 2 if i == 'bar' else 3 if i == 'qux' else 4 if i == 'spam'else 5 if i == 'ham' else 6
n = lambda i: 0 if i == 'foo' else 1 if i == 'baz' else 2 if i == 'bar' else 3 if i == 'qux' else 4 if i == 'spam'else 5 if i == 'ham' else 6
def lambda_function(i):
return n(i)
def np_where(i):
return np.where(possible_strings_array == i)[0][0]
##
def check(function):
for i in testlist:
function(i)
possible_strings_list = ['foo', 'bar', 'baz', 'qux', 'spam', 'ham', 'eggs']
testlist = [random.choice(possible_strings_list) for i in range(1000)]
possible_strings_dict = {'foo':0, 'bar':1, 'baz':2, 'qux':3, 'spam':4, 'ham':5, 'eggs':6}
possible_strings_tup = ('foo', 'bar', 'baz', 'qux', 'spam', 'ham', 'eggs')
allfunctions = [list_index, dictionary, if_elif_else, lambda_function, tupple_index, ternary, np_where]
for function in allfunctions:
t = timeit.Timer(lambda: check(function))
print(function.__name__, t.timeit(number=10000))
字典查找是執行此搜索的最快方法。 在進行這樣的分析時,通常可以比較每個過程的時間復雜度 。
對於字典查找,時間復雜度為“恆定時間”或O(1)。 盡管這通常意味着算法可以采用的整數步長,但在這種情況下實際上是一個整數。
其他方法將需要迭代(或者在if else遍歷的情況下-本質上是類似的方法)。 這些范圍從需要查看所有值O(n)到需要查看某些值O(log n)。
由於n是檢查集的大小,並且隨着檢查集的變大,結果的差異也將隨之增加,其中字典始終優於顯示的其他選項。
沒有比O(1)更快的方法。 您展示的方法的唯一缺點是,隨着集合的增長,可能需要更多的內存,這被稱為算法的空間復雜度。 但是,在這種情況下,由於我們只需要每個值一個值,因此空間復雜度為O(n),可以忽略不計。
在一般意義上的優化中,重要的是要考慮當前解決方案中存在多少復雜性,以及對提高該復雜性有多大意義。 如果要進行改進,則應針對不同級別的性能,例如從O(n)到O(log n)或O(log n)到O(1)。
圖片提供: http : //bigocheatsheet.com/
微觀優化往往是在相同的復雜度層上進行優化的情況,而這些優化本身並不具有建設性。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.