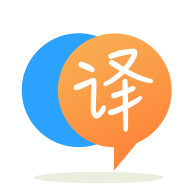
[英]What are the differences between a list, sorted list, and an array list? (c#)
[英]Find all differences in sorted array
我有一個實際值的排序(升序)數組,稱之為(可能重復)。 我希望在給定一系列值[x,y]的情況下,找到索引j存在的所有值(i)的索引,使得:j> i和x <= a [j] -a [i] <=或者簡單地說,找到在給定范圍內存在“前向差異”的值。
輸出是一個長度為a.Length的布爾數組。 由於數組是對所有前向差異進行排序,因此x和y為正。
我設法做的最好的是從每個索引開始查看它前面的子陣列並執行二進制搜索x + a [i]並檢查是否[j] <= y + a [i]。 我認為這是O(n log n)。 有更好的方法嗎? 或者我可以做些什么來加快速度。
我應該注意到,最終我想在同一個數組a上搜索許多這樣的范圍[x,y],但是范圍的數量遠小於數組的長度(小4-6個數量級) - 因此我更關心搜索的復雜性。
例:
a= 0, 1, 46, 100, 185, 216, 285
范圍x,y = [99,101]應該返回:
[true, true, false, false, true, false, false]
僅對於值0,1和185在該范圍內具有前向差異。
內存中的代碼可能有一些錯誤:
int bin_search_closesmaller(int arr[], int key, int low, int high)
{
if (low > high) return high;
int mid = (high - low)/2;
if (arr[mid] > key) return bin_search_closesmaller(arr, key, low, mid - 1);
if (arr[mid] < key) return bin_search_closesmaller(arr, key, mid + 1, high);
return mid;
}
bool[] findDiffs(int[] a, int x, int y)
{
bool[] result = new bool[a.Length];
for(int i=0; i<a.Length-1;i++)
{
int idx=bin_search_closesmaller(a, y+a[i], i+1, a.Length-1);
if (idx==-1) continue;
if (a[idx]-a[i] >= x) result[i]=true;
}
}
謝謝!
讓兩個指標left
和right
,通過陣列走。 Right
索引移動直到超出當前left
索引的范圍,然后檢查前一個元素是否在范圍內。 索引僅向前移動,因此算法是線性的
right=2
for left = 0 to n-1:
while A[right] < A[left] + MaxRangeValue
right++
Result[left] = (A[right - 1] <= A[left] + MinRangeValue)
關於這個算法的另一種觀點:
- 差異太小,增量正確
- 差異太大,增量左
只要輸入數組已排序,就可以找到問題的線性解決方案。 關鍵是使用兩個索引來遍歷數組a
。
bool[] findDiffs(int[] a, int x, int y)
{
bool[] result = new boolean[a.Length];
int j = 0;
for (int i = 0; i < a.Length; ++i) {
while (j < a.Length && a[j] - a[i] < x) {
++j;
}
if (j < a.Length) {
result[i] = a[j] - a[i] <= y;
}
}
return result;
}
a = [0,100,1000,1100]
和(x,y) = (99,100)
:
i = 0, j = 0 => a[j] - a[i] = 0 < x=99 => ++j
i = 0, j = 1 => a[j] - a[i] = 100 <= y=100 => result[i] = true; ++i
i = 1, j = 1 => a[j] - a[i] = 0 < x=99 => ++j
i = 1, j = 2 => a[j] - a[i] = 900 > y=100 => result[i] = false; ++i
i = 2, j = 2 => a[j] - a[i] = 0 <= x=99 => ++j
i = 2, j = 3 => a[j] - a[i] = 100 <= y=100 => result[i] = true; ++i
i = 3, j = 3 => a[j] - a[i] = 0 <= x=99 => exit loop
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.