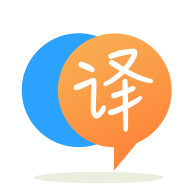
[英]Normalize output of fft using Libgdx in Android, from accelerometer data
[英]How to get degrees by Accelerometer data (Java libGDX)
如何從加速度計的值中獲取度數? 我在Android Studio中使用libGDX和Java代碼。 我有一個精靈動畫,它可以直走。 視點從頂部是正交的,傾斜智能手機時我想旋轉精靈。
我如何在屏幕上獲得360度,例如像指南針那樣,它只是指向北,它應該指向智能手機傾斜的方向。 加速度傳感器怎么辦? 或者我還有什么其他可能性?
對不起我的英語不好
一種簡單的方法是使用SensorManager並實現SensorEventListener。 基本思想是使用SensorManager注冊方向傳感器,然后在通過SensorEventListener實現的onSensorChanged委托方法中響應設備方向的更改。 確保在onPause()上取消注冊偵聽器,否則將耗盡電池電量。
作為高級示例:
public class SensorActivity extends Activity implements SensorEventListener {
private Sensor mOrientationSensor;
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
SensorManager mSensorManager = (SensorManager) getActivity().getSystemService(Context.SENSOR_SERVICE);
mOrientationSensor = mSensorManager.getDefaultSensor(Sensor.TYPE_ORIENTATION);
}
@Override
public void onResume() {
super.onResume();
if (mSensorManager != null) {
mSensorManager.registerListener(this, mOrientationSensor, SensorManager.SENSOR_DELAY_UI);
}
}
@Override
public void onPause() {
super.onPause();
mSensorManager.unregisterListener(this, mOrientationSensor);
}
@Override
public void onSensorChanged(SensorEvent event) {
float degree = Math.round(event.values[0]);
// do something here
}
注意:雖然我仍然認為方向傳感器效果最好,但已經棄用了方向傳感器。 如果您想嘗試更新的方法,請參見下面的方法。
來自android文檔: https : //developer.android.com/guide/topics/sensors/sensors_position.html#sensors-pos-orient
public class SensorActivity extends Activity implements SensorEventListener {
private SensorManager mSensorManager;
private final float[] mAccelerometerReading = new float[3];
private final float[] mMagnetometerReading = new float[3];
private final float[] mRotationMatrix = new float[9];
private final float[] mOrientationAngles = new float[3];
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
mSensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE);
}
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) {
// Do something here if sensor accuracy changes.
// You must implement this callback in your code.
}
@Override
protected void onResume() {
super.onResume();
// Get updates from the accelerometer and magnetometer at a constant rate.
// To make batch operations more efficient and reduce power consumption,
// provide support for delaying updates to the application.
//
// In this example, the sensor reporting delay is small enough such that
// the application receives an update before the system checks the sensor
// readings again.
mSensorManager.registerListener(this, Sensor.TYPE_ACCELEROMETER,
SensorManager.SENSOR_DELAY_NORMAL, SensorManager.SENSOR_DELAY_UI);
mSensorManager.registerListener(this, Sensor.TYPE_MAGNETIC_FIELD,
SensorManager.SENSOR_DELAY_NORMAL, SensorManager.SENSOR_DELAY_UI);
}
@Override
protected void onPause() {
super.onPause();
// Don't receive any more updates from either sensor.
mSensorManager.unregisterListener(this);
}
// Get readings from accelerometer and magnetometer. To simplify calculations,
// consider storing these readings as unit vectors.
@Override
public void onSensorChanged(SensorEvent event) {
if (event.sensor == Sensor.TYPE_ACCELEROMETER) {
System.arraycopy(event.values, 0, mAccelerometerReading,
0, mAccelerometerReading.length);
}
else if (event.sensor == Sensor.TYPE_MAGNETIC_FIELD) {
System.arraycopy(event.values, 0, mMagnetometerReading,
0, mMagnetometerReading.length);
}
}
// Compute the three orientation angles based on the most recent readings from
// the device's accelerometer and magnetometer.
public void updateOrientationAngles() {
// Update rotation matrix, which is needed to update orientation angles.
mSensorManager.getRotationMatrix(mRotationMatrix, null,
mAccelerometerReading, mMagnetometerReading);
// "mRotationMatrix" now has up-to-date information.
mSensorManager.getOrientation(mRotationMatrix, mOrientationAngles);
// "mOrientationAngles" now has up-to-date information.
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.