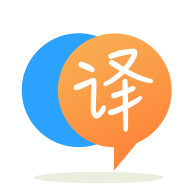
[英]Register IPipelineBehavior in ASP.NET Core 3 with autofac
[英]AutoFac / .NET Core - Register DBcontext
我有一個新的 .NET Core Web API 項目,它具有以下項目結構:
API -> 業務/領域 -> 基礎設施
API 非常精簡,只有 API 方法。 業務/領域層包含我所有的業務邏輯。 最后,我的基礎設施層擁有使用 EF Core 2.0 的數據庫類。
我知道使用 .NET Core 內置依賴注入,我可以將 API 項目的引用添加到基礎結構項目,然后在 StartUp.cs 文件中添加以下代碼:
services.AddDbContext<MyContext>(options => options.UseSqlServer(connectionString));
但是,我想保持更傳統的關注點分離。 到目前為止,我已經在我的基礎設施層添加了一個模塊,它嘗試像這樣進行注冊:
builder.Register(c =>
{
var config = c.Resolve<IConfiguration>();
var opt = new DbContextOptionsBuilder<MyContext>();
opt.UseSqlServer(config.GetSection("ConnectionStrings:MyConnection:ConnectionString").Value);
return new MyContext(opt.Options);
}).AsImplementedInterfaces().InstancePerLifetimeScope();
但是,DBContext 並未注冊。 任何嘗試訪問注入的 DBContext 的類都無法解析該參數。
有沒有辦法在 .NET Core Web API 項目中使用 AuftoFac 在單獨的項目中注冊 DBContext?
我使用 Autofac 注冊HttpContextAccessor
和DbContext
。
builder
.RegisterType<HttpContextAccessor>()
.As<IHttpContextAccessor>()
.SingleInstance();
builder
.RegisterType<AppDbContext>()
.WithParameter("options", DbContextOptionsFactory.Get())
.InstancePerLifetimeScope();
數據庫上下文選項工廠
public class DbContextOptionsFactory
{
public static DbContextOptions<AppDbContext> Get()
{
var configuration = AppConfigurations.Get(
WebContentDirectoryFinder.CalculateContentRootFolder());
var builder = new DbContextOptionsBuilder<AppDbContext>();
DbContextConfigurer.Configure(
builder,
configuration.GetConnectionString(
AppConsts.ConnectionStringName));
return builder.Options;
}
}
數據庫上下文配置器
public class DbContextConfigurer
{
public static void Configure(
DbContextOptionsBuilder<AppDbContext> builder,
string connectionString)
{
builder.UseNpgsql(connectionString).UseLazyLoadingProxies();
}
}
我認為問題在於您正在嘗試使用MyContext()
注冊MyContext()
AsImplementedInterfaces()
。 這不是 DbContext 通常注冊的方式。 您應該注冊並解析類本身。
Autofac 4.8.1 版的另一個簡單解決方案
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc().AddControllersAsServices();
services.AddDbContext<MyContext>(options => options.UseSqlServer(Configuration.GetConnectionString("ConnectionStrings:MyConnection:ConnectionString")));
var builder = new ContainerBuilder();
builder.Populate(services);
//...
// Your interface registration
//...
builder.Build(Autofac.Builder.ContainerBuildOptions.None);
}
這是我使用的一個實現 - 它模仿EF Core 3.1 注冊到 Autofac 4.9.4。 請務必根據您的要求調整范圍。
public void RegisterContext<TContext>(ContainerBuilder builder)
where TContext : DbContext
{
builder.Register(componentContext =>
{
var serviceProvider = componentContext.Resolve<IServiceProvider>();
var configuration = componentContext.Resolve<IConfiguration>();
var dbContextOptions = new DbContextOptions<TContext>(new Dictionary<Type, IDbContextOptionsExtension>());
var optionsBuilder = new DbContextOptionsBuilder<TContext>(dbContextOptions)
.UseApplicationServiceProvider(serviceProvider)
.UseSqlServer(configuration.GetConnectionString("MyConnectionString"),
serverOptions => serverOptions.EnableRetryOnFailure(5, TimeSpan.FromSeconds(30), null));
return optionsBuilder.Options;
}).As<DbContextOptions<TContext>>()
.InstancePerLifetimeScope();
builder.Register(context => context.Resolve<DbContextOptions<TContext>>())
.As<DbContextOptions>()
.InstancePerLifetimeScope();
builder.RegisterType<TContext>()
.AsSelf()
.InstancePerLifetimeScope();
}
在所需的項目中,您可以創建一個擴展方法,將上下文添加到集合中
public static class MyDataExtensions {
public static IServiceCollection AddMyData(this IServiceCollection services) {
//...
services.AddDbContext<MyContext>(options => options.UseSqlServer(connectionString));
//...
}
}
有了它,然后在您啟動時,只需調用從其他項目公開的擴展即可
services.AddMyData();
//...other settings
API 項目是組合根,因此無論如何它都需要知道所有相關的依賴項。 至少使用此擴展,您不必直接引用使用的 db 上下文,
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.