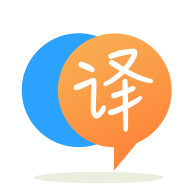
[英]“Win32 exception occurred releasing IUnknown at…” error using Pylons and WMI
[英]Win32 exception upon releasing virtualbox
我正在嘗試在線程中運行Virtualbox。 在while循環的第二次迭代中,th變量被重新分配,應用程序崩潰並生成Win32異常。 這似乎是由vbox和會話的發布引起的。 我的問題是如何正確手動釋放它們?
Win32 exception occurred releasing IUnknown at 0x035e40b8
Win32 exception occurred releasing IUnknown at 0x04babcb0
我的應用程序的基礎知識(來自pyvbox和virtualbox sdk的virtualbox)
import virtualbox
import threading
import time
class ThreadExecutor(threading.Thread):
def __init__(self):
self.vbox = None
self.session = None
self.vm = None
super().__init__()
def run(self):
self.vbox = virtualbox.VirtualBox()
self.session = virtualbox.Session()
self.vm = self.vbox.find_machine("Ubuntu")
self.vm.launch_vm_process(self.session, 'gui', '')
time.sleep(30)
if int(self.session.state) == 1:
print('Boot failed!')
return
else:
print('Powering down')
self.session.console.power_down()
print('Operation completed')
return
if __name__ == '__main__':
while True:
print('Input')
if input():
th = ThreadExecutor()
th.start()
print('Thread started')
time.sleep(5)
while th.isAlive():
print('App running')
time.sleep(5)
print('Execution finished')
為了可靠地釋放會話,您應該使用try / except / finally結構,如下所示:
try:
#Your code
except:
print("something unexpected happend")
finally:
self.session.console.power_down()
我修改了代碼(沒有運行它):
為了解決問題並進行調試( 無意於對其進行任何修復 -至少不在此階段)。
code.py :
#!/usr/bin/env python3
import sys
import virtualbox
import threading
import time
DEFAULT_DEBUG_MSG_INDENT = " "
def debug(text, indent_count, indent=DEFAULT_DEBUG_MSG_INDENT, leading_eoln_count=0):
print("{:s}{:s}[TID: {:06d}]: {:s}".format("\n" * leading_eoln_count, indent * indent_count, threading.get_ident(), text))
class ThreadExecutor(threading.Thread):
def __init__(self):
super().__init__()
self.vbox = virtualbox.VirtualBox()
self.session = virtualbox.Session()
if not self.vbox or not self.session:
raise RuntimeError("virtualbox initialization failed")
self.vm = self.vbox.find_machine("Ubuntu")
if not self.vm:
raise ValueError("VM not found")
def run(self):
start_wait_time = 15
debug("Starting VM...", 1)
self.vm.launch_vm_process(self.session, "gui", "")
debug("Sleeping {:d} secs...".format(start_wait_time), 1)
time.sleep(start_wait_time)
if int(self.session.state) == 1:
debug("Boot failed!", 1)
return
else:
debug("Powering down", 1)
self.session.console.power_down()
debug("Operation completed", 1)
def run(threaded=True):
debug("RUNNING with{:s} threads".format("" if threaded else "out"), 0, leading_eoln_count=1)
sleep_time = 5
while input("{:s}Press ANY key followed by ENTER to continue, ENTER ONLY to queet: ".format(DEFAULT_DEBUG_MSG_INDENT)):
th = ThreadExecutor()
if threaded:
debug("Starting thread...", 0)
th.start()
debug("Thread started", 1)
time.sleep(sleep_time)
while th.isAlive():
debug("App running", 1)
time.sleep(sleep_time)
else:
debug("Running...", 0)
th.run()
debug("Execution finished", 1)
debug("Done", 0)
def main():
run(threaded=False)
run()
if __name__ == "__main__":
print("Python {:s} on {:s}\n".format(sys.version, sys.platform))
main()
而且有效!!!
輸出 :
(py35x64_test) e:\\Work\\Dev\\StackOverflow\\q051136288>"e:\\Work\\Dev\\VEnvs\\py35x64_test\\Scripts\\python.exe" code.py Python 3.5.4 (v3.5.4:3f56838, Aug 8 2017, 02:17:05) [MSC v.1900 64 bit (AMD64)] on win32 [TID: 036256]: RUNNING without threads Press ANY key followed by ENTER to continue, ENTER ONLY to queet: a [TID: 036256]: Running... [TID: 036256]: Starting VM... [TID: 036256]: Sleeping 15 secs... [TID: 036256]: Powering down [TID: 036256]: Operation completed [TID: 036256]: Execution finished Press ANY key followed by ENTER to continue, ENTER ONLY to queet: a [TID: 036256]: Running... [TID: 036256]: Starting VM... [TID: 036256]: Sleeping 15 secs... [TID: 036256]: Powering down [TID: 036256]: Operation completed [TID: 036256]: Execution finished Press ANY key followed by ENTER to continue, ENTER ONLY to queet: [TID: 036256]: Done [TID: 036256]: RUNNING with threads Press ANY key followed by ENTER to continue, ENTER ONLY to queet: a [TID: 036256]: Starting thread... [TID: 038520]: Starting VM... [TID: 036256]: Thread started [TID: 038520]: Sleeping 15 secs... [TID: 036256]: App running [TID: 036256]: App running [TID: 036256]: App running [TID: 038520]: Powering down [TID: 038520]: Operation completed [TID: 036256]: Execution finished Press ANY key followed by ENTER to continue, ENTER ONLY to queet: a [TID: 036256]: Starting thread... [TID: 028884]: Starting VM... [TID: 036256]: Thread started [TID: 028884]: Sleeping 15 secs... [TID: 036256]: App running [TID: 036256]: App running [TID: 036256]: App running [TID: 028884]: Powering down [TID: 028884]: Operation completed [TID: 036256]: Execution finished Press ANY key followed by ENTER to continue, ENTER ONLY to queet: [TID: 036256]: Done
原始代碼被拋出(僅相關部分):
pywintypes.com_error: (-2147221008, 'CoInitialize has not been called.', None, None)
我懷疑在初始化程序中移動virualbox init可能是行為更改的原因(盡管正如我所說,我只是移動了代碼,因為我認為初始化程序是正確的位置)-正確:將代碼移回運行再次觸發異常。
在對virtualbox和vboxapi代碼進行了簡短檢查之后,我認為這與發生CoInitializeEx的位置(線程)有關,但是我無法動彈 。
我還嘗試在主塊中手動調用該函數( pythoncom.CoInitializeEx(0)
),但是僅import pythoncom
會觸發在第一次迭代中出現的異常。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.