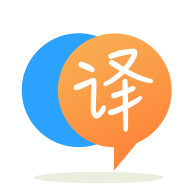
[英]How to read a binary file quickly in c#? (ReadOnlySpan vs MemoryStream)
[英]C# ReadOnlySpan<char> vs Substring for string dissection
我有一個相當簡單的字符串擴展方法,它在我所做的很多字符串操作的系統中經常被調用。 我讀了這篇文章( String.Substring()似乎是這個代碼的瓶頸 )並且認為我會嘗試相同的方法,看看我是否可以通過改變我讀取字符串的方式來找到一些性能。 我的結果並不是我所期待的(我期待ReadOnlySpan提供顯着的性能提升),我想知道為什么會這樣。 在我的實際運行代碼中,我發現性能略有下降。
我使用我關心的字符生成了一個包含~115萬行字符串的文件,在每個字符串上調用方法,並將結果轉儲到控制台。
我的結果(以毫秒為單位的運行時間)是:
ReadOnlySpan.IndexOf Framework 4.7.1: 68538
ReadOnlySpan.IndexOf Core 2.1: 64486
ReadOnlySpan.SequenceEqual Framework 4.7.1: 63650
ReadOnlySpan.SequenceEqual Core 2.1: 65071
substring Framework 4.7.1: 63508
substring Core 2.1: 64125
代碼(從完整框架到核心2.1完全相同):
調用代碼:
static void Main(string[] args)
{
Stopwatch sw = new Stopwatch();
sw.Start();
var f = File.ReadAllLines("periods.CSV");
foreach (string s in f)
{ Console.WriteLine(s.CountOccurrences(".")); }
sw.Stop();
Console.WriteLine("Done in " + sw.ElapsedMilliseconds + " ms");
Console.ReadKey();
}
我方法的原始子串形式:
public static int CountOccurrencesSub(this string val, string searchFor)
{
if (string.IsNullOrEmpty(val) || string.IsNullOrEmpty(searchFor))
{ return 0; }
int count = 0;
for (int x = 0; x <= val.Length - searchFor.Length; x++)
{
if (val.Substring(x, searchFor.Length) == searchFor)
{ count++; }
}
return count;
}
ReadOnlySpan版本(我用IndexOf和SequenceEqual測試了相等性檢查):
public static int CountOccurrences(this string val, string searchFor)
{
if (string.IsNullOrEmpty(val) || string.IsNullOrEmpty(searchFor))
{ return 0; }
int count = 0;
ReadOnlySpan<char> vSpan = val.AsSpan();
ReadOnlySpan<char> searchSpan = searchFor.AsSpan();
for (int x = 0; x <= vSpan.Length - searchSpan.Length; x++)
{
if (vSpan.Slice(x, searchSpan.Length).SequenceEqual(searchSpan))
{ count++; }
}
return count;
}
相等比較是否在我正在調用的方法中進行分配,因此沒有提升? 這對ReadOnlySpan來說不是一個好的應用程序嗎? 我只是簡單地失去了什么?
雖然我有點遲到了,但我想我仍然可以在這個主題上添加相關信息。
首先,關於其他海報測量的一些話。
OP的結果顯然不正確。 正如評論中指出的那樣,I / O操作完全扭曲了統計數據。
接受的答案的海報是在正確的軌道上。 他的方法消除了緩慢的I / O操作,並明確關注基准測試的主題。 但是,他沒有提到使用的環境(尤其是.NET運行時),他的“熱身方法”是值得商榷的。
績效評估是一項非常棘手的業務,很難做到正確。 如果我想獲得有效的結果,我甚至不會嘗試自己編碼。 所以我決定使用廣泛采用的Benchmark.NET庫來查看這個問題。 為了使這更有趣,我添加了第三個候選人。 這個實現使用String.CompareOrdinal進行出現計數,我期望得到相當好的結果。
在測量開始之前(在全局設置階段),我生成1,000,000行lorem ipsum文本。 該數據在整個測量過程中使用。
每種方法都使用1,000和1,000,000行,並使用較短(5個字符長)和較長(39個字符長)的搜索文本。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Running;
namespace MyBenchmarks
{
#if NETCOREAPP2_1
[CoreJob]
#else
[ClrJob]
#endif
[RankColumn, MarkdownExporterAttribute.StackOverflow]
public class Benchmark
{
static readonly string[] words = new[]
{
"lorem", "ipsum", "dolor", "sit", "amet", "consectetuer",
"adipiscing", "elit", "sed", "diam", "nonummy", "nibh", "euismod",
"tincidunt", "ut", "laoreet", "dolore", "magna", "aliquam", "erat"
};
// borrowed from greg (https://stackoverflow.com/questions/4286487/is-there-any-lorem-ipsum-generator-in-c)
static IEnumerable<string> LoremIpsum(Random random, int minWords, int maxWords, int minSentences, int maxSentences, int numLines)
{
var line = new StringBuilder();
for (int l = 0; l < numLines; l++)
{
line.Clear();
var numSentences = random.Next(maxSentences - minSentences) + minSentences + 1;
for (int s = 0; s < numSentences; s++)
{
var numWords = random.Next(maxWords - minWords) + minWords + 1;
line.Append(words[random.Next(words.Length)]);
for (int w = 1; w < numWords; w++)
{
line.Append(" ");
line.Append(words[random.Next(words.Length)]);
}
line.Append(". ");
}
yield return line.ToString();
}
}
string[] lines;
[Params(1000, 1_000_000)]
public int N;
[Params("lorem", "lorem ipsum dolor sit amet consectetuer")]
public string SearchValue;
[GlobalSetup]
public void GlobalSetup()
{
lines = LoremIpsum(new Random(), 6, 8, 2, 3, 1_000_000).ToArray();
}
public static int CountOccurrencesSub(string val, string searchFor)
{
if (string.IsNullOrEmpty(val) || string.IsNullOrEmpty(searchFor))
{ return 0; }
int count = 0;
for (int x = 0; x <= val.Length - searchFor.Length; x++)
{
if (val.Substring(x, searchFor.Length) == searchFor)
{ count++; }
}
return count;
}
public static int CountOccurrences(string val, string searchFor)
{
if (string.IsNullOrEmpty(val) || string.IsNullOrEmpty(searchFor))
{ return 0; }
int count = 0;
ReadOnlySpan<char> vSpan = val.AsSpan();
ReadOnlySpan<char> searchSpan = searchFor.AsSpan();
for (int x = 0; x <= vSpan.Length - searchSpan.Length; x++)
{
if (vSpan.Slice(x, searchSpan.Length).SequenceEqual(searchSpan))
{ count++; }
}
return count;
}
public static int CountOccurrencesCmp(string val, string searchFor)
{
if (string.IsNullOrEmpty(val) || string.IsNullOrEmpty(searchFor))
{ return 0; }
int count = 0;
for (int x = 0; x <= val.Length - searchFor.Length; x++)
{
if (string.CompareOrdinal(val, x, searchFor, 0, searchFor.Length) == 0)
{ count++; }
}
return count;
}
[Benchmark(Baseline = true)]
public int Substring()
{
int occurences = 0;
for (var i = 0; i < N; i++)
occurences += CountOccurrencesSub(lines[i], SearchValue);
return occurences;
}
[Benchmark]
public int Span()
{
int occurences = 0;
for (var i = 0; i < N; i++)
occurences += CountOccurrences(lines[i], SearchValue);
return occurences;
}
[Benchmark]
public int Compare()
{
int occurences = 0;
for (var i = 0; i < N; i++)
occurences += CountOccurrencesCmp(lines[i], SearchValue);
return occurences;
}
}
public class Program
{
public static void Main(string[] args)
{
BenchmarkRunner.Run<Benchmark>();
}
}
}
NET Core 2.1
BenchmarkDotNet=v0.11.0, OS=Windows 7 SP1 (6.1.7601.0)
Intel Core i3-4360 CPU 3.70GHz (Haswell), 1 CPU, 4 logical and 2 physical cores
Frequency=3604970 Hz, Resolution=277.3948 ns, Timer=TSC
.NET Core SDK=2.1.400
[Host] : .NET Core 2.1.2 (CoreCLR 4.6.26628.05, CoreFX 4.6.26629.01), 64bit RyuJIT
Core : .NET Core 2.1.2 (CoreCLR 4.6.26628.05, CoreFX 4.6.26629.01), 64bit RyuJIT
Job=Core Runtime=Core
Method | N | SearchValue | Mean | Error | StdDev | Median | Scaled | ScaledSD | Rank |
---------- |-------- |--------------------- |---------------:|----------------:|----------------:|---------------:|-------:|---------:|-----:|
Substring | 1000 | lorem | 2,149.4 us | 2.2763 us | 2.1293 us | 2,149.4 us | 1.00 | 0.00 | 3 |
Span | 1000 | lorem | 555.5 us | 0.2786 us | 0.2470 us | 555.5 us | 0.26 | 0.00 | 1 |
Compare | 1000 | lorem | 1,471.8 us | 0.2133 us | 0.1891 us | 1,471.8 us | 0.68 | 0.00 | 2 |
| | | | | | | | | |
Substring | 1000 | lorem(...)etuer [39] | 2,128.7 us | 1.0414 us | 0.9741 us | 2,128.6 us | 1.00 | 0.00 | 3 |
Span | 1000 | lorem(...)etuer [39] | 388.9 us | 0.0440 us | 0.0412 us | 388.9 us | 0.18 | 0.00 | 1 |
Compare | 1000 | lorem(...)etuer [39] | 1,215.6 us | 0.7016 us | 0.6220 us | 1,215.5 us | 0.57 | 0.00 | 2 |
| | | | | | | | | |
Substring | 1000000 | lorem | 2,239,510.8 us | 241,887.0796 us | 214,426.5747 us | 2,176,083.7 us | 1.00 | 0.00 | 3 |
Span | 1000000 | lorem | 558,317.4 us | 447.3105 us | 418.4144 us | 558,338.9 us | 0.25 | 0.02 | 1 |
Compare | 1000000 | lorem | 1,471,941.2 us | 190.7533 us | 148.9276 us | 1,471,955.8 us | 0.66 | 0.05 | 2 |
| | | | | | | | | |
Substring | 1000000 | lorem(...)etuer [39] | 2,350,820.3 us | 46,974.4500 us | 115,229.1264 us | 2,327,187.2 us | 1.00 | 0.00 | 3 |
Span | 1000000 | lorem(...)etuer [39] | 433,567.7 us | 14,445.7191 us | 42,593.5286 us | 417,333.4 us | 0.18 | 0.02 | 1 |
Compare | 1000000 | lorem(...)etuer [39] | 1,299,065.2 us | 25,474.8504 us | 46,582.2045 us | 1,296,892.8 us | 0.55 | 0.03 | 2 |
NET Framework 4.7.2
BenchmarkDotNet=v0.11.0, OS=Windows 7 SP1 (6.1.7601.0)
Intel Core i3-4360 CPU 3.70GHz (Haswell), 1 CPU, 4 logical and 2 physical cores
Frequency=3604960 Hz, Resolution=277.3956 ns, Timer=TSC
[Host] : .NET Framework 4.7.2 (CLR 4.0.30319.42000), 64bit RyuJIT-v4.7.3062.0
Clr : .NET Framework 4.7.2 (CLR 4.0.30319.42000), 64bit RyuJIT-v4.7.3062.0
Job=Clr Runtime=Clr
Method | N | SearchValue | Mean | Error | StdDev | Median | Scaled | ScaledSD | Rank |
---------- |-------- |--------------------- |---------------:|---------------:|----------------:|---------------:|-------:|---------:|-----:|
Substring | 1000 | lorem | 2,025.8 us | 2.4639 us | 1.9237 us | 2,025.4 us | 1.00 | 0.00 | 3 |
Span | 1000 | lorem | 1,216.6 us | 4.2994 us | 4.0217 us | 1,217.8 us | 0.60 | 0.00 | 1 |
Compare | 1000 | lorem | 1,295.5 us | 5.2427 us | 4.6475 us | 1,293.1 us | 0.64 | 0.00 | 2 |
| | | | | | | | | |
Substring | 1000 | lorem(...)etuer [39] | 1,939.5 us | 0.4428 us | 0.4142 us | 1,939.3 us | 1.00 | 0.00 | 3 |
Span | 1000 | lorem(...)etuer [39] | 944.9 us | 2.6648 us | 2.3622 us | 944.7 us | 0.49 | 0.00 | 1 |
Compare | 1000 | lorem(...)etuer [39] | 1,002.0 us | 0.2475 us | 0.2067 us | 1,002.1 us | 0.52 | 0.00 | 2 |
| | | | | | | | | |
Substring | 1000000 | lorem | 2,065,805.7 us | 2,009.2139 us | 1,568.6619 us | 2,065,555.1 us | 1.00 | 0.00 | 3 |
Span | 1000000 | lorem | 1,209,976.4 us | 6,238.6091 us | 5,835.5982 us | 1,206,554.3 us | 0.59 | 0.00 | 1 |
Compare | 1000000 | lorem | 1,303,321.8 us | 1,257.7418 us | 1,114.9552 us | 1,303,330.1 us | 0.63 | 0.00 | 2 |
| | | | | | | | | |
Substring | 1000000 | lorem(...)etuer [39] | 2,085,652.9 us | 62,651.7471 us | 168,309.8501 us | 1,973,522.2 us | 1.00 | 0.00 | 3 |
Span | 1000000 | lorem(...)etuer [39] | 958,421.2 us | 3,703.5508 us | 3,464.3034 us | 958,324.9 us | 0.46 | 0.03 | 1 |
Compare | 1000000 | lorem(...)etuer [39] | 1,007,936.8 us | 802.1730 us | 750.3531 us | 1,007,680.3 us | 0.49 | 0.04 | 2 |
很明顯,使用Span <T>可以獲得可靠的性能提升 。 有點令人驚訝的是,它在.NET Core上只有4-5倍,在.NET Framework上只有2倍。 那可能是什么原因? 有人有線索嗎?
String.CompareOrdinal也表現得很好。 我預計會有更好的結果,因為理論上它只是逐字節比較,但它並沒有壞。 在.NET Framework上,它無論如何都是可行的選擇。
搜索字符串的長度(當然除了四肢)似乎對結果沒有太大影響。
我很好奇,並試圖重復你的測試。 根據數據集的大小,使用ReadOnlySpan
的代碼執行速度幾乎快兩倍:
CountOccurences Done in 1080 ms
CountOccurencesSub Done in 1789 ms
對於較大的數據集,差異似乎增加(這似乎是合理的,因為Substring分配一個字符串,這會增加GC壓力)。
我用這段代碼來測試:
static void Main(string[] args)
{
var r = new Random();
// generate 100000 lines of 1000 random characters
var text = Enumerable.Range(0, 100000).Select(x => new string(Enumerable.Range(0, 1000).Select(i => (char)r.Next(255)).ToArray())).ToArray();
// warm up
"".CountOccurrencesSub("");
"".CountOccurrences("");
Measure(text, "CountOccurencesSub", s => s.CountOccurrencesSub("."));
Measure(text, "CountOccurences", s => s.CountOccurrences("."));
Console.ReadKey();
}
private static void Measure(string[] text, string test, Action<string> action)
{
Stopwatch sw = new Stopwatch();
sw.Start();
foreach (string s in text)
{
action(s);
}
sw.Stop();
Console.WriteLine($"{test} Done in {sw.ElapsedMilliseconds} ms");
}
使用ReadOnlySpan對我造成最大傷害的性能基准是,當我試圖在一個放入Windows應用商店的應用程序中使用它時,它讓我處於一個受傷的世界。 ReadOnlySpan <>的東西與.NETNative工具鏈的編譯不兼容(但似乎)。 反過來,這可能(或可能不會)觸發一系列后續WACK測試錯誤,這些錯誤將阻止提交到商店。
到目前為止,我花了兩天時間試圖將一個使用ReadOnlySpan <>的應用程序添加到商店中。 到目前為止,遠遠超過了我可能獲得的任何可能的性能改進。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.