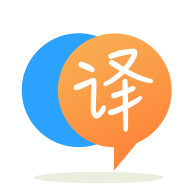
[英]ValueError: Error when checking target: expected dense_2 to have 4 dimensions, but got array with shape (7942, 1)
[英]ValueError: Error when checking target: expected dense_19 to have 3 dimensions, but got array with shape (5, 3)
我有以下代碼使用帶有 TensorFlow 后端的 Keras 創建 LSTM 網絡。 這段代碼運行良好。
import numpy as np
import pandas as pd
from sklearn import model_selection
from keras.models import Sequential
from keras.layers import Dense, Activation, Dropout
from keras.layers.recurrent import LSTM
from keras.utils import np_utils
flights = {
'flight_stage': [1,0,1,1,0,0,1],
'scheduled_hour': [16,16,17,17,17,18,18],
'delay_category': [1,0,2,2,1,0,2]
}
columns = ['flight_stage', 'scheduled_hour', 'delay_category']
df = pd.DataFrame(flights, columns=columns)
X = df.drop('delay_category',1)
y = df['delay_category']
X_train, X_test, y_train, y_test = model_selection.train_test_split(X, y, test_size=0.25, random_state=42)
nb_features = X_train.shape[1]
nb_classes = y.nunique()
hidden_neurons = 32
timestamps = X_train.shape[0]
# Reshape input data to 3D array
X_train = X_train.values.reshape(1, X_train.shape[0], X_train.shape[1])
X_test = X_test.values.reshape(1, X_test.shape[0], X_test.shape[1])
y_train = np_utils.to_categorical(y_train, nb_classes)
y_test = np_utils.to_categorical(y_test, nb_classes)
model = Sequential()
model.add(LSTM(
units=hidden_neurons,
return_sequences=True,
input_shape=(timestamps,nb_features)
)
)
model.add(Dropout(0.2))
model.add(Dense(activation='softmax', units=nb_classes))
model.compile(loss="categorical_crossentropy",
optimizer='adadelta')
但是當我開始訓練模型時,它失敗了:
history = model.fit(X_train, y_train, validation_split=0.25, epochs=500, batch_size=2, shuffle=True, verbose=0)
錯誤:
ValueError: Error when checking target: expected dense_19 to have 3 dimensions, but got array with shape (5, 3)
此錯誤指的是最終的 Dense 層。 我使用model.summary()
來獲得精確的尺寸。 密集層的輸出形狀是(None, 5, 3)
。 但是我不明白為什么它有 3 個維度以及None
代表什么(它是如何出現在最后一層的)?
3 是最后一層返回的單元數。 它是 softmax 激活的類數
5 是 lstm 返回的單元數,表示返回的序列的大小
None 是最后一層的批次元素數。 它只是意味着最后一層可以接受每批形狀 [5, 3] 的張量的不同大小
X_train shape: (1, 5, 2),
X_test shape: (1, 2, 2),
y_train shape: (5,3),
y_test shape: (2,3)
查看數據形狀,特征的批量大小與標簽的批量大小之間顯然存在不匹配。 最左邊的數字在特征形狀 X 和標簽形狀 y 之間應該相等。 它是批量大小。
'1', 5, 2 => batch size of 1
'2', 3 => batch size of 2
這里有一個不匹配。 同樣為了解決 lstm 層的輸出和最后一層的輸入之間的問題,可以使用layer.flatten
nb_classes = 3
hidden_neurons = 32
model = Sequential()
model.add(LSTM(
units=hidden_neurons,
return_sequences=True,
input_shape=(5, 2)
)
)
model.add(Dropout(0.2))
model.add(Flatten())
model.add(Dense(activation='softmax', units=nb_classes))
model.compile(loss="categorical_crossentropy",
optimizer='adadelta')
model.compile(loss='categorical_crossentropy',
optimizer='adam')
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.