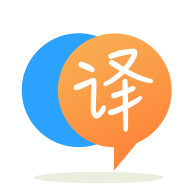
[英]How can I add generate more conversations automatic when typing the int field numbers?
[英]How can I add a Conversations on top level above the Dialogues?
在對話框觸發腳本頂部,我添加了一個新的List類型字符串:
using System.Collections;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using UnityEngine;
public class DialogueTrigger : MonoBehaviour
{
public List<string> conversation = new List<string>();
public List<Dialogue> dialogue = new List<Dialogue>();
[HideInInspector]
public int dialogueNum = 0;
private bool triggered = false;
private List<Dialogue> oldDialogue;
private void Start()
{
//oldDialogue = dialogue.ToList();
}
public void TriggerDialogue()
{
if (triggered == false)
{
if (FindObjectOfType<DialogueManager>() != null)
{
FindObjectOfType<DialogueManager>().StartDialogue(dialogue[dialogueNum]);
dialogueNum += 1;
}
triggered = true;
}
}
private void Update()
{
if (DialogueManager.dialogueEnded == true)
{
if (dialogueNum == dialogue.Count)
{
return;
}
else
{
FindObjectOfType<DialogueManager>().StartDialogue(dialogue[dialogueNum]);
DialogueManager.dialogueEnded = false;
dialogueNum += 1;
}
}
}
}
然后在編輯器腳本中:
using System.Collections;
using System.Collections.Generic;
using UnityEditor;
using UnityEditorInternal;
using UnityEngine;
[CustomEditor(typeof(DialogueTrigger))]
public class DialogueTriggerEditor : Editor
{
private SerializedProperty _dialogues;
private SerializedProperty _conversations;
private void OnEnable()
{
// do this only once here
_dialogues = serializedObject.FindProperty("dialogue");
_conversations = serializedObject.FindProperty("conversation");
}
public override void OnInspectorGUI()
{
//base.OnInspectorGUI();
serializedObject.Update();
_conversations.arraySize = EditorGUILayout.IntField("Conversation Size", _conversations.arraySize);
// Ofcourse you also want to change the list size here
_dialogues.arraySize = EditorGUILayout.IntField("Dialogue Size", _dialogues.arraySize);
for (int x = 0; x < _conversations.arraySize; x++)
{
for (int i = 0; i < _dialogues.arraySize; i++)
{
var dialogue = _dialogues.GetArrayElementAtIndex(i);
EditorGUILayout.PropertyField(dialogue, new GUIContent("Dialogue " + i), true);
}
}
// Note: You also forgot to add this
serializedObject.ApplyModifiedProperties();
}
}
我正在_對話循環上進行_conversations循環。 但這不是我想要的。
我希望在頂層的檢查器中只有“對話大小”。
如果“對話大小”為0,則沒有任何內容。 但是,例如,如果“會話大小”為5。
然后將創建5個會話(會話1,會話2 ......會話5)。 在每個對話下都有一個Dialouge大小,然后我可以在每個對話中進行許多對話。
這樣,以后識別對話和對話將變得更加容易。 而是像以前那樣進行了長時間的對話。
在此之前,我添加了會話列表,只是沒有會話並且工作正常,但現在我想添加會話。 因此,每個對話都有自己的對話。
這是根據解決方案的腳本:
創建了類:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[System.Serializable]
public class Conversation
{
public string Id;
public List<Dialogue> Dialogues = new List<Dialogue>();
}
然后觸發腳本:
using System.Collections;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using UnityEngine;
public class DialogueTrigger : MonoBehaviour
{
public List<Conversation> conversations = new List<Conversation>();
public List<Dialogue> dialogue = new List<Dialogue>();
[HideInInspector]
public int dialogueNum = 0;
private bool triggered = false;
private List<Dialogue> oldDialogue;
private void Start()
{
//oldDialogue = dialogue.ToList();
}
public void TriggerDialogue()
{
if (triggered == false)
{
if (FindObjectOfType<DialogueManager>() != null)
{
FindObjectOfType<DialogueManager>().StartDialogue(dialogue[dialogueNum]);
dialogueNum += 1;
}
triggered = true;
}
}
private void Update()
{
if (DialogueManager.dialogueEnded == true)
{
if (dialogueNum == dialogue.Count)
{
return;
}
else
{
FindObjectOfType<DialogueManager>().StartDialogue(dialogue[dialogueNum]);
DialogueManager.dialogueEnded = false;
dialogueNum += 1;
}
}
}
}
最后是觸發器編輯器腳本:
using System.Collections;
using System.Collections.Generic;
using UnityEditor;
using UnityEditorInternal;
using UnityEngine;
[CustomEditor(typeof(DialogueTrigger))]
public class DialogueTriggerEditor : Editor
{
private SerializedProperty _dialogues;
private SerializedProperty _conversations;
private void OnEnable()
{
_conversations = serializedObject.FindProperty("conversations");
}
public override void OnInspectorGUI()
{
//base.OnInspectorGUI();
serializedObject.Update();
_conversations.arraySize = EditorGUILayout.IntField("Conversations Size", _conversations.arraySize);
for (int x = 0; x < _conversations.arraySize; x++)
{
var conversation = _conversations.GetArrayElementAtIndex(x);
var Id = conversation.FindPropertyRelative("Id");
EditorGUILayout.PropertyField(Id);
_dialogues = conversation.FindPropertyRelative("Dialogues");
_dialogues.arraySize = EditorGUILayout.IntField("Dialogues size", _dialogues.arraySize);
for (int i = 0; i < _dialogues.arraySize; i++)
{
var dialogue = _dialogues.GetArrayElementAtIndex(i);
EditorGUILayout.PropertyField(dialogue, new GUIContent("Dialogue " + i), true);
}
}
serializedObject.ApplyModifiedProperties();
}
}
屏幕截圖結果:
我認為檢查器中的所有Id應該是“對話”大小的子級,所有“對話”大小應該是Id的子級,然后“對話”應該是“對話大小”的子級。
Conversation Size
Id 1
Dialogues Size
Dialogue 1
Name
Sentences
Dialogue 2
這樣的事情。
您應該上一堂適當的課
[Serializable]
public class Conversation
{
public string Id;
public List<Dialogue> Dialogues = new List<Dialogue>();
}
而不是在其中列出
public DialogueTrigget : MonoBehaviour
{
public List<Conversation> Conversations = new List<Conversation> ();
...
}
比起您上次使用的腳本,您可以使用完全相同的腳本,但是
_conversations = serializedObject.FindProperty("Conversations");
因此一個for循環更類似於您已經嘗試過的..類似
[CustomEditor(typeof(DialogueTrigger))]
public class DialogueTriggerEditor : Editor
{
private SerializedProperty _dialogues;
private SerializedProperty _conversations;
private void OnEnable()
{
_conversations = serializedObject.FindProperty("conversation");
}
public override void OnInspectorGUI()
{
//base.OnInspectorGUI();
serializedObject.Update();
_conversations.arraySize = EditorGUILayout.IntField("Conversation Size", _conversations.arraySize);
for (int x = 0; x < _conversations.arraySize; x++)
{
var conversation = _conversations.GetArrayElementAtIndex(x);
var id = conversation.FindPropertyRelative("I'd");
EditorGUI.indentLevel ++;
EditorGUILayout.PropertyField(Id);
var dialogues = conversation.FindPropertyRelative("Dialogues");
dialogues.arraySize = EditorGUILayout.IntField("Dialogues size", dialogues.arraySize);
for (int i = 0; i < dialogues.arraySize; i++)
{
var dialogue = _dialogues.GetArrayElementAtIndex(i);
EditorGUI.indentLevel++;
EditorGUILayout.PropertyField(dialogue, new GUIContent("Dialogue " + i), true);
EditorGUI.indentLevel--;
}
EditorGUI.indentLevel--;
}
serializedObject.ApplyModifiedProperties();
}
}
或者簡單地
EditorGUILayout.PropertyField(_conversations, new GUIContent ("Conversations"), true);
但這不會打印“對話1,對話2”等
同樣,我強烈建議您查看嵌套的ReorderableList ,一旦工作,它就會很棒。
在我的智能手機上鍵入內容,因此沒有保修
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.