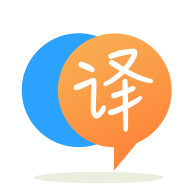
[英]Is Array.Copy safe when the source and destination are the same array?
[英]Example of Dijkstra algorithm when source and destination are the same
給定以下有向加權圖,如何找到在B中開始和結束的最短路徑?
我正在嘗試Dijkstra,並且存在路徑和不存在路徑的兩種情況都運行良好,但是找不到一個示例來解決我上面所問的情況。
到目前為止,這是我的代碼
public static int ShortestDistance(Graph graph, Node from, Node to)
{
var distances = new Dictionary<Node, int>();
var actualNodes = graph.GetNodes() as List<Node> ?? Graph.GetNodes().ToList();
foreach (var node in actualNodes) distances[node] = node.Equals(from) ? 0 : int.MaxValue;
while (actualNodes.Count() != 0)
{
var actualShortest = actualNodes.OrderBy(n => distances[n]).First();
actualNodes.Remove(actualShortest);
if (distances[actualShortest] == int.MaxValue) break;
if (actualShortest.Equals(to)) return distances[actualShortest];
foreach (var adjacent in graph.GetAdjacentsByNode(actualShortest))
{
var actualDistance = distances[actualShortest] + adjacent.Weight;
if (actualDistance >= distances[adjacent.To]) continue;
distances[adjacent.To] = actualDistance;
}
}
throw new Exception($"There's no such route from '{from}' to '{to}'.");
}
如果允許零長度路由:
如果按路線表示長度> 0的路徑:
從源代碼運行Dijkstra,獲取數組sp [],以便sp [x]存儲從源代碼到x的最短路徑(這是Dijkstra的常規用法)
現在考慮所有傳入源的邊。
假設邊是x->權重為w的源
因此我們可以到達路徑> 0長度且總權重為sp [x] + w的源
在所有此類路線中,至少選擇一個。
做到這一點的規范方法是使用相同的輸入和輸出邊緣和權重來復制(或“陰影”)節點B
(稱為BB
)。
現在,應用Dijkstra算法找到從B
到BB
的最短路徑。 您已經有了該代碼(即“我們現在已將問題減少到以前已解決的問題”)。
將節點分為兩個節點:
S
保留所有傳出邊緣 D
保留所有傳入邊緣 現在通常將S
作為源,將D
作為目標。
您對該算法的實現非常慢,但是可以正常工作。 如果要搜索從節點到其自身的> 0路徑,則可以像這樣更改初始化:
public static int ShortestDistance(Graph graph, Node from, Node to)
{
var distances = new Dictionary<Node, int>();
var actualNodes = graph.GetNodes() as List<Node> ?? Graph.GetNodes().ToList();
foreach (var node in actualNodes) distances[node] = int.MaxValue;
foreach (var adjacent in graph.GetAdjacentsByNode(from))
{
distances[adjacent.To] = adjacent.Weight;
}
while (actualNodes.Count() != 0)
{
var actualShortest = actualNodes.OrderBy(n => distances[n]).First();
actualNodes.Remove(actualShortest);
if (distances[actualShortest] == int.MaxValue) break;
if (actualShortest.Equals(to)) return distances[actualShortest];
foreach (var adjacent in graph.GetAdjacentsByNode(actualShortest))
{
var actualDistance = distances[actualShortest] + adjacent.Weight;
if (actualDistance >= distances[adjacent.To]) continue;
distances[adjacent.To] = actualDistance;
}
}
throw new Exception($"There's no such route from '{from}' to '{to}'.");
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.