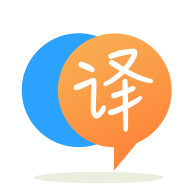
[英]Python — How can I find the square matrix of a lower triangular numpy matrix? (with a symmetrical upper triangle)
[英]How can I create a correlation matrix from 2 vectors of its upper and lower triangle in python?
我有兩個向量,每個向量對應一個相關矩陣的一半。 說,
a = [0.1 0.2 0.3 0.4 0.5 0.6]
b = [0.11 0.22 0.33 0.44 0.55 0.66]
我想做的是將它們組裝成這樣的矩陣:
correlation_matrix = [1 0.1 0.2 0.3
0.11 1 0.4 0.5 <-- a
b --> 0.22 0.44 1 0.6
0.33 0.55 0.66 1]
是否有一個函數在python中執行它?
這是使用numpy
做到這一點的一種方法。
import numpy as np
a = np.array([0.1, 0.2, 0.3, 0.4, 0.5, 0.6])
b = np.array([0.11, 0.22, 0.33, 0.44, 0.55, 0.66])
首先設置您期望的最終相關矩陣的大小,並創建一個單位矩陣:
n = 4
results = np.identity(n)
獲得上三角指數和分配的值a
。 切換行和列索引並指定b
值:
rows, cols = np.triu_indices(n, 1) # The 1 denotes offset from diagonal
results[rows, cols] = a
results[cols, rows] = b
結果:
array([[1. , 0.1 , 0.2 , 0.3 ],
[0.11, 1. , 0.4 , 0.5 ],
[0.22, 0.44, 1. , 0.6 ],
[0.33, 0.55, 0.66, 1. ]])
您可以使用基本的for循環和if語句:
a = [0.1, 0.2 ,0.3,0.4 ,0.5 ,0.6]
b = [0.11, 0.22, 0.33 ,0.44, 0.55 ,0.66]
count_a = 0
count_b = 0
for i in range(4):
for j in range(4):
if j == i :
print ("{:<5}".format(1), end = " ")
elif (i>j):
print ("{:<5}".format(b[count_b]), end = " ")
count_b += 1
elif (i<j):
print ("{:<5}".format(a[count_a]), end = " ")
count_a += 1
print()
輸出:
1 0.1 0.2 0.3
0.11 1 0.4 0.5
0.22 0.33 1 0.6
0.44 0.55 0.66 1
編輯:
a = [0.1, 0.2, 0.3, 0.4, 0.5, 0.6]
b = [0.11, 0.22, 0.33, 0.44, 0.55, 0.66]
Matrix = []
count_a = 0
count_b = 0
for i in range(4):
row = []
for j in range(4):
if j == i:
row.append(1)
elif (i > j):
row.append(b[count_b])
count_b += 1
elif (i < j):
row.append(a[count_a])
count_a += 1
Matrix.append(row)
print (Matrix)
輸出:
[[1, 0.1, 0.2, 0.3],
[0.11, 1, 0.4, 0.5],
[0.22, 0.33, 1, 0.6],
[0.44, 0.55, 0.66, 1]]
要么:
import numpy as np
print(np.matrix(Matrix))
輸出:
[[1. 0.1 0.2 0.3 ]
[0.11 1. 0.4 0.5 ]
[0.22 0.33 1. 0.6 ]
[0.44 0.55 0.66 1. ]]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.