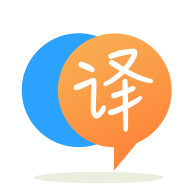
[英]Error when checking input: expected conv2d_1_input to have shape (50, 50, 1) but got array with shape (50, 50, 3)
[英]Keras CONV1D: Error when checking target: expected decoded output to have shape (50, 50) but got array with shape (50, 1)
我遇到了這個問題: 檢查目標時出錯:預期 decoded_output 的形狀為 (50, 50) 但得到的數組的形狀為 (50, 1)解碼輸出):
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
TAM_VECTOR = X_train.shape[1]
input_tweet = Input(shape=(TAM_VECTOR,X_train.shape[2]))
encoded = Conv1D(64, kernel_size=1, activation='relu')(input_tweet)
encoded = Conv1D(32, kernel_size=1, activation='relu')(encoded)
decoded = Conv1D(32, kernel_size=1, activation='relu')(encoded)
decoded = Conv1D(64, kernel_size=1, activation='relu')(decoded)
decoded = Conv1D(TAM_VECTOR, kernel_size=1, activation='relu', name='decode_output')(decoded)
encoded = Flatten()(encoded)
second_output = Dense(1, activation='linear', name='second_output')(encoded)
autoencoder = Model(inputs=input_tweet, outputs=[decoded, second_output])
autoencoder.compile(optimizer="adam",
loss={'decode_output': 'mse', 'second_output': 'mse'},
loss_weights={'decode_output': 0.001, 'second_output': 0.999},
metrics=["mae"])
autoencoder.fit([X_train], [X_train, y_train], epochs=10, batch_size=32)
輸入 (X) 具有形狀 (50000,50) 但由於 Conv1D 接收到 3D 輸入,因此我將其整形為:
X = np.reshape(X, (X.shape[0], X.shape[1], -1))
(50000,50,1)
y(第二個輸出)是
y.shape
(50000,1)
這里是 model 總結
Layer (type) Output Shape Param # Connected to
==================================================================================================
input_43 (InputLayer) (None, 50, 1) 0
__________________________________________________________________________________________________
conv1d_169 (Conv1D) (None, 50, 64) 128 input_43[0][0]
__________________________________________________________________________________________________
conv1d_170 (Conv1D) (None, 50, 32) 2080 conv1d_169[0][0]
__________________________________________________________________________________________________
conv1d_171 (Conv1D) (None, 50, 32) 1056 conv1d_170[0][0]
__________________________________________________________________________________________________
conv1d_172 (Conv1D) (None, 50, 64) 2112 conv1d_171[0][0]
__________________________________________________________________________________________________
flatten_62 (Flatten) (None, 1600) 0 conv1d_170[0][0]
__________________________________________________________________________________________________
decode_output (Conv1D) (None, 50, 50) 3250 conv1d_172[0][0]
__________________________________________________________________________________________________
pib_output (Dense) (None, 1) 1601 flatten_62[0][0]
==================================================================================================
Total params: 10,227
Trainable params: 10,227
Non-trainable params: 0
下一行中的 TAM_VECTOR 應替換為 1。
代替
decoded = Conv1D(TAM_VECTOR, kernel_size=1, activation='relu', name='decode_output')(decoded)
和
decoded = Conv1D(1, kernel_size=1, activation='relu', name='decode_output')(decoded)
解碼后的 output 形狀應與自動編碼器的輸入形狀 (50,1) 匹配。
from keras.layers import Conv1D, Flatten, Dense, Input
from keras.models import Model
import numpy as np
TAM_VECTOR = 50
input_tweet = Input(shape=(TAM_VECTOR,1))
encoded = Conv1D(64, kernel_size=1, activation='relu')(input_tweet)
encoded = Conv1D(32, kernel_size=1, activation='relu')(encoded)
decoded = Conv1D(32, kernel_size=1, activation='relu')(encoded)
decoded = Conv1D(64, kernel_size=1, activation='relu')(decoded)
decoded = Conv1D(1, kernel_size=1, activation='relu', name='decode_output')(decoded)
encoded = Flatten()(encoded)
second_output = Dense(1, activation='linear', name='second_output')(encoded)
autoencoder = Model(inputs=input_tweet, outputs=[decoded, second_output])
autoencoder.compile(optimizer="adam",
loss={'decode_output': 'mse', 'second_output': 'mse'},
loss_weights={'decode_output': 0.001, 'second_output': 0.999},
metrics=["mae"])
autoencoder.fit(np.ones((1,50,1)), [np.ones((1,50,1)), np.ones((1,1))])
1/1 [==============================] - 0s 407ms/步 - 損失:0.9112 - decode_output_loss:0.9549 - second_output_loss :0.9111 - decode_output_mean_absolute_error:0.9772 - second_output_mean_absolute_error:0.9545
這是錯誤:錯誤1):
InvalidArgumentError: 2 root error(s) found.
(0) Invalid argument: You must feed a value for placeholder tensor 'decode_output_sample_weights_32' with dtype float and shape [?]
[[{{node decode_output_sample_weights_32}}]]
[[loss_2/second_output_loss/Mean_3/_3217]]
(1) Invalid argument: You must feed a value for placeholder tensor 'decode_output_sample_weights_32' with dtype float and shape [?]
[[{{node decode_output_sample_weights_32}}]]
0 successful operations.
0 derived errors ignored.
錯誤2):
InvalidArgumentError: You must feed a value for placeholder tensor 'decode_output_target_17' with dtype float and shape [?,?,?] [[{{node decode_output_target_17}}]]
錯誤3):
UnknownError: 2 root error(s) found.
(0) Unknown: Failed to get convolution algorithm. This is probably because cuDNN failed to initialize, so try looking to see if a warning log message was printed above.
[[{{node conv1d_1/convolution}}]]
[[loss/add/_157]]
(1) Unknown: Failed to get convolution algorithm. This is probably because cuDNN failed to initialize, so try looking to see if a warning log message was printed above.
[[{{node conv1d_1/convolution}}]]
0 successful operations.
0 derived errors ignored.
錯誤 4):
UnknownError: 2 root error(s) found.
(0) Unknown: Failed to get convolution algorithm. This is probably because cuDNN failed to initialize, so try looking to see if a warning log message was printed above.
[[{{node conv1d_25/convolution}}]]
[[loss_6/second_output_loss/Mean_3/_1025]]
(1) Unknown: Failed to get convolution algorithm. This is probably because cuDNN failed to initialize, so try looking to see if a warning log message was printed above.
[[{{node conv1d_25/convolution}}]]
0 successful operations.
0 derived errors ignored.
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.