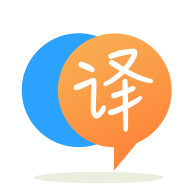
[英]Create a list of nested dictionaries from a single csv file in python
[英]Python: How to create a csv string (no file) from a list of dictionaries?
在 Python 我有一個這樣的字典列表:
[
{
"col2": "2",
"id": "1",
"col3": "3",
"col1": "1"
},
{
"col2": "4",
"id": "2",
"col3": "6",
"col1": "2"
},
{
"col1": "1",
"col2": "4",
"id": "3",
"col3": "7"
}
]
我需要將其轉換為 csv 格式的字符串,包括 header 行。 (對於初學者來說,讓我們不關心列和行分隔符......)所以,理想的結果是:
id,col1,col2,col3
1,1,2,3
2,2,4,6
3,1,4,7
(“理想情況下”,因為列順序並不重要;雖然首先有“id”列會很好......)
我搜索了 SOF 並且有許多類似的問題,但答案總是涉及使用 csv.DictWriter 創建一個 csv文件。 我不想創建文件,我只想要那個字符串!
當然,我可以遍歷列表並在此循環內循環遍歷字典鍵,並以這種方式使用字符串操作創建 csv 字符串。 但是肯定有一些更優雅和有效的方法來做到這一點?
另外,我知道 Pandas 庫,但我試圖在一個非常有限的環境中執行此操作,我寧願只使用內置模塊。
最簡單的方法是使用 pandas:
import pandas as pd
df = pd.DataFrame.from_dict(your_list_of_dicts)
print(df.to_csv(index=False))
結果:
col1,col2,col3,id
1,2,3,1
2,4,6,2
1,4,7,3
如果你想重新排序列,沒有比這更容易的了:
col_order = ['id', 'col1', 'col2', 'col3']
df[col_order].to_csv(index=False)
或者,只是確保id
列是第一個:
df.set_index('id', inplace=True) # the index is always printed first
df.to_csv() # leave the index to True this time
具有內置功能:
from collections import OrderedDict
ord_d = OrderedDict().fromkeys(('id', 'col1', 'col2', 'col3'))
s = ','.join(ord_d.keys()) + '\n'
for d in lst:
ord_d.update(d)
s += ','.join(ord_d.values()) + '\n'
print(s)
output:
id,col1,col2,col3
1,1,2,3
2,2,4,6
3,1,4,7
這個想法是獲取所有可能的鍵並獲取所有值。 假設數據是您擁有的字典列表。 這應該有效:
output = ''
all_keys = set().union(*(d.keys() for d in data))
output += ",".split(all_keys) + '\n'
for item in data:
item_str = ",".split([data[key] for key in all_keys if key in data else ''])
output += item_str + '\n'
您可以使用io.StringIO
寫入“字符串”而不是文件。 以csv.DictWriter
為例,我們得到以下代碼:
import csv
import io
data = [...] # your list of dicts
with io.StringIO() as csvfile:
fieldnames = ['id', 'col1', 'col2', 'col3']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for row in data:
writer.writerow(row)
print(csvfile.getvalue())
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.