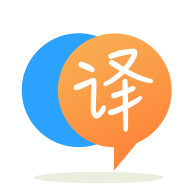
[英]How to implement Composite Primary key and Composite Foreign Key using JPA,Hibernate, Springboot
[英]JPA & Hibernate - Composite primary key with foreign key
所以我有兩個表,我想在 Spring memory 中進行查詢。 我已經成功地管理了一個名為 Medicine 的條目中的“Drugs”表 model,但是現在需要 model 的“drugInteraction”表 - 它將具有 drug_id(葯物表中的 PK 稱為 id)和 drugInteraction 中的 drug_name 的組合表,作為組合主鍵。
python 中使用的模式:
cursor.execute("CREATE TABLE drugs(id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), description TEXT, toxicity TEXT)")
cursor.execute("CREATE TABLE drugInteractions (drug_id INT NOT NULL, name VARCHAR(90), description TEXT, PRIMARY KEY(drug_id, name), FOREIGN KEY (drug_id) REFERENCES drugs (id))")
drugInteractions 表的一些示例數據:
drug_id name description
1 "Abciximab" "The risk or severity of bleeding can be increased when Abciximab is combined with Lepirudin."
1 "Aceclofenac" "The risk or severity of bleeding and hemorrhage can be increased when Aceclofenac is combined with Lepirudin."
1. "Acemetacin" "The risk or severity of bleeding and hemorrhage can be increased when Lepirudin is combined with Acemetacin."
葯物表的一些示例數據:
id. name. description
1 "Lepirudin" "Lepirudin is identical to...."
2 "Cetuximab" "Cetuximab is an epidermal growth..."
這是我的 Medicine.java:
package com.example.configbackendspring;
import net.minidev.json.JSONObject;
import javax.persistence.*;
@Entity
@Table(name = "drugs")
public class Medicine {
@Id
@GeneratedValue
@Column(name = "id")
private Integer id;
@Column(name = "name")
private String name;
@Column(name = "description")
private String description;
@Column(name = "toxicity")
private String toxicity;
public Medicine(int id, String name, String description, String toxicity) {
this.id=id;
this.name=name;
this.description=description;
this.toxicity=toxicity;
}
public Medicine(){}
public int getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getToxicity() {
return toxicity;
}
public void setToxicity(String toxicity) {
this.toxicity = toxicity;
}
public JSONObject toJSONObject(){
JSONObject object = new JSONObject();
JSONObject medicineObject = new JSONObject();
medicineObject.appendField("name", this.name);
medicineObject.appendField("description", this.description);
medicineObject.appendField("toxicity", this.toxicity);
medicineObject.appendField("id", this.id);
object.appendField("medicine", medicineObject);
return object;
}
}
這就是我所擁有的 drugInteraction.java ......這不起作用
package com.example.configbackendspring;
import net.minidev.json.JSONObject;
import javax.persistence.*;
import javax.resource.cci.Interaction;
import java.io.Serializable;
@Entity
@Table(name = "drugInteractions")
public class DrugInteraction {
@EmbeddedId
private InteractionId interactionId;
@Column(name = "description")
private String description;
public DrugInteraction(int drug_id, String name, String description) {
this.interactionId.drug_name = name;
this.interactionId.drug_id = drug_id;
this.description=description;
}
public DrugInteraction(){}
public Integer getId() {
return interactionId.drug_id;
}
public String getName() {
return interactionId.drug_name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public JSONObject toJSONObject(){
JSONObject object = new JSONObject();
JSONObject interactionObject = new JSONObject();
interactionObject.appendField("name", interactionId.drug_name);
interactionObject.appendField("description", this.description);
interactionObject.appendField("drug_id", interactionId.drug_id);
object.appendField("drugInteraction", interactionObject);
return object;
}
}
這是 InteractionId.java
package com.example.configbackendspring;
import lombok.*;
import java.io.Serializable;
@RequiredArgsConstructor
@NoArgsConstructor
@Getter
@Setter
@ToString
@EqualsAndHashCode
public class InteractionId implements Serializable
{
// public InteractionId(int drug_id, String drug_name){
// this.drug_id=drug_id;
// this.drug_name=drug_name;
//
// }
@NonNull
public int drug_id;
@NonNull
public String drug_name;
}
我目前的問題是我無法弄清楚如何將葯物中的外鍵 id 與復合鍵鏈接起來。 上面的代碼正在編譯,但數據庫是空的,所以導入一定是在某處失敗
請有人建議我如何將文件更改為 model 上述行為? 我無法弄清楚如何使用外鍵 model 復合 ID
你有很多地方錯了。 如果您使用的是@EmbeddedId
,那么您的 ID class 需要是可嵌入的。
@Embeddable
public class InteractionId {
@Column(name="name")
String name;
Long drugId; //type should be same as for ID field on Medicine
//equals and hashcode etc.
}
您還需要從DrugInteraction
到用MapsId
注釋的Medicine
的關系:
@Entity
@Table(name = "drugInteractions")
public class DrugInteraction {
@EmbeddedId
private InteractionId interactionId;
@MapsId("drugId")//value corresponds to property in the ID class
@ManyToOne
@JoinColumn(name = "drug_id")
private Medicine medicine;
}
要保存新實例:
DrugInteraction di = new DrugInteraction();
Medicine medicine = //an existing medicine
di.setName("Some Name");
di.setMedicine(medicine);
//save
作為替代方案,也可以使用IDClass
而不是EmbeddedId
執行此操作:
//not an embeddable
public class InteractionId {
String name;
Long drugId; //type should be same as for ID field on Medicine
//equals and hashcode etc.
}
並更改映射:
@Entity
@Table(name = "drugInteractions")
@IdClass(InteractionId.class) //specify the ID class
public class DrugInteraction {
@Id
private String name;
@Id
@ManyToOne
@JoinColumn(name = "drug_id")
private Medicine medicine;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.