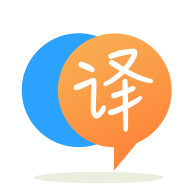
[英]Pyspark - How to apply a function only to a subset of columns in a DataFrame?
[英]How to apply a function to a set of columns of a PySpark dataframe by rows?
給定一個數據框,如:
A0 A1 A2 A3
0 9 1 2 8
1 9 7 6 9
2 1 7 4 6
3 0 8 4 8
4 0 1 6 0
5 7 1 4 3
6 6 3 5 9
7 3 3 2 8
8 6 3 0 8
9 3 2 7 1
我需要將一個函數逐行應用於一組列,以使用此函數的結果創建一個新列。
Pandas 中的一個例子是:
df = pd.DataFrame(data=None, columns=['A0', 'A1', 'A2', 'A3'])
df['A0'] = np.random.randint(0, 10, 10)
df['A1'] = np.random.randint(0, 10, 10)
df['A2'] = np.random.randint(0, 10, 10)
df['A3'] = np.random.randint(0, 10, 10)
df['mean'] = df.mean(axis=1)
df['std'] = df.iloc[:, :-1].std(axis=1)
df['any'] = df.iloc[:, :-2].apply(lambda x: np.sum(x), axis=1)
結果是:
A0 A1 A2 A3 mean std any
0 9 1 2 8 5.00 4.082483 20
1 9 7 6 9 7.75 1.500000 31
2 1 7 4 6 4.50 2.645751 18
3 0 8 4 8 5.00 3.829708 20
4 0 1 6 0 1.75 2.872281 7
5 7 1 4 3 3.75 2.500000 15
6 6 3 5 9 5.75 2.500000 23
7 3 3 2 8 4.00 2.708013 16
8 6 3 0 8 4.25 3.500000 17
9 3 2 7 1 3.25 2.629956 13
如何在 PySpark 中做類似的事情?
對於 Spark 2.4+,您可以使用aggregate
函數。 首先,使用所有數據框列創建數組列values
。 然后,像這樣計算std
、 means
和any
列:
any
:聚合以求和數組元素mean
:將any
列除以數組values
的大小std
: 聚合和總和(x - mean) ** 2
然后除以數組的length - 1
這是相關的代碼:
from pyspark.sql.functions import expr, sqrt, size, col, array
data = [
(9, 1, 2, 8), (9, 7, 6, 9), (1, 7, 4, 6),
(0, 8, 4, 8), (0, 1, 6, 0), (7, 1, 4, 3),
(6, 3, 5, 9), (3, 3, 2, 8), (6, 3, 0, 8),
(3, 2, 7, 1)
]
df = spark.createDataFrame(data, ['A0', 'A1', 'A2', 'A3'])
cols = df.columns
df.withColumn("values", array(*cols)) \
.withColumn("any", expr("aggregate(values, 0D, (acc, x) -> acc + x)")) \
.withColumn("mean", col("any") / size(col("values"))) \
.withColumn("std", sqrt(expr("""aggregate(values, 0D,
(acc, x) -> acc + power(x - mean, 2),
acc -> acc / (size(values) -1))"""
)
)) \
.drop("values") \
.show(truncate=False)
#+---+---+---+---+----+----+------------------+
#|A0 |A1 |A2 |A3 |any |mean|std |
#+---+---+---+---+----+----+------------------+
#|9 |1 |2 |8 |20.0|5.0 |4.08248290463863 |
#|9 |7 |6 |9 |31.0|7.75|1.5 |
#|1 |7 |4 |6 |18.0|4.5 |2.6457513110645907|
#|0 |8 |4 |8 |20.0|5.0 |3.8297084310253524|
#|0 |1 |6 |0 |7.0 |1.75|2.8722813232690143|
#|7 |1 |4 |3 |15.0|3.75|2.5 |
#|6 |3 |5 |9 |23.0|5.75|2.5 |
#|3 |3 |2 |8 |16.0|4.0 |2.70801280154532 |
#|6 |3 |0 |8 |17.0|4.25|3.5 |
#|3 |2 |7 |1 |13.0|3.25|2.6299556396765835|
#+---+---+---+---+----+----+------------------+
火花 < 2.4 :
您可以使用functools.reduce
和operator.add
對列求和。 邏輯和上面一樣:
from functools import reduce
from operator import add
df.withColumn("any", reduce(add, [col(c) for c in cols])) \
.withColumn("mean", col("any") / len(cols)) \
.withColumn("std", sqrt(reduce(add, [(col(c) - col("mean")) ** 2 for c in cols]) / (len(cols) -1)))\
.show(truncate=False)
上面的答案很好,但是我看到 OP 正在使用 Python/PySpark,如果您不理解 Spark SQL 表達式,則上述邏輯不是 100% 清楚。
我建議使用 Pandas UDAF,與 UDF 不同的是,它們是矢量化的並且非常有效。 這已添加到 Spark API 中,以降低從 Pandas 遷移到 Spark 所需的學習曲線。 這也意味着,如果您的大多數同事(例如我的同事)更熟悉 Pandas/Python,則您的代碼更易於維護。
這些是可用的 Pandas UDAF 類型及其等效的 Pandas
例如 SparkUdafType → df.pandasEquivalent(...) 作用於 → 返回
SCALAR → df.transform(...), 映射系列 → 系列
GROUPED_MAP → df.apply(...) , Group & MapDataFrame → DataFrame
GROUPED_AGG → df.aggregate(...), Reduce Series → Scalar
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.