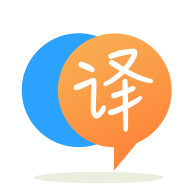
[英]graph-tools ValueError with graph_union for filtered graphs
[英]Get all possible graphs with a given number of nodes and edges (with graph-tools)
我對圖形很陌生,我的知識接近於 0。但我需要構建一個 model 以獲得具有給定邊數、給定節點數和每個節點之間的最大度數的所有可能圖形。
因此,例如,我想獲得所有具有 8 個節點和每個節點之間恰好有 3 個連接(邊)的圖形可能性。
我也想把所有的可能性都當作一本字典,就像這樣:
{ 1 : [2,3,4],
2 : [5,3,1],
3 : [6,2,7]
... and so on
當然,一條邊不能與其自身相連。
到目前為止,我嘗試使用圖形工具庫( here )
我嘗試的是:
from graph_tool.all import *
def degree () :
return 3,3
g = random_graph(8, degree)
a = g.get_edges([g.edge_index])
print(a)
其中 output 我:
[[ 0 7 0]
[ 0 5 2]
[ 0 2 1]
[ 1 7 12]
[ 1 5 14]
[ 1 6 13]
[ 2 3 9]
[ 2 4 10]
[ 2 1 11]
[ 3 6 22]
[ 3 0 23]
[ 3 1 21]
[ 4 3 3]
[ 4 1 5]
[ 4 0 4]
[ 5 2 20]
[ 5 0 19]
[ 5 4 18]
[ 6 7 15]
[ 6 5 16]
[ 6 4 17]
[ 7 6 8]
[ 7 3 7]
[ 7 2 6]]
有人可以解釋一下我在這里做錯了什么嗎? (例如為什么第一個列表是 0,7,0 (這是什么意思......我再次對這種東西完全陌生))
如果我只定義了 8 個節點,為什么會有大於 7 的數字?
我怎樣才能得到所有的可能性(8 個節點的所有圖和每個節點之間的 3 個連接)?
我不確定您要實現什么目標,但我編寫了一個代碼來解決類似問題,並從使用圖形工具庫生成的隨機不同圖形生成數據結構。
它可能會幫助您獲得所需的東西。
如果您有任何問題,請告訴我。
from graph_tool.all import *
import json
def isDifferentList(list1, list2):
return list1 != list2
def isDifferentGraph(graph1, graph2):
for k in graph1.keys():
if isDifferentList(graph1[k], graph2[k]):
# if all lists of connection exists, return true and stop all iterations,
# this mean that the graph exists, so need to generate a new one
return True
#the graph does not exist, we can continue with the current one
return False
def graphExists(graph, all):
for generated in all:
if not isDifferentGraph(graph, generated):
return True
return False
def generate_output_data(gr):
gGraph = {}
for vertex in gr.get_edges():
vertex0 = int(vertex[0]) + 1
vertex1 = int(vertex[1]) + 1
if int(vertex0) not in gGraph:
gGraph[vertex0] = []
if vertex1 not in gGraph:
gGraph[vertex1] = []
gGraph[vertex0].append(vertex1)
gGraph[vertex1].append(vertex0)
return gGraph
def getRandomGraph():
return generate_output_data(random_graph(VERTICES, lambda: DEGREE, directed=False))
def defineDegreeAndvertexs(vertexs, in_degree):
global VERTICES, DEGREE
DEGREE = in_degree
VERTICES = vertexs
def generateNgraph(in_vertices, in_degree, n_graphs):
#first store inputs in globals variable
defineDegreeAndvertexs(in_vertices, in_degree)
#generate empty list of generated uniques graphs
all_graphs = []
for i in range(0, n_graphs):
#generate a new random graph and get it as a desired output data struct
g = getRandomGraph()
# check if this graph already exists and generate a new one as long as it already been generated
while graphExists(g, all_graphs):
g = getRandomGraph()
#Write the new graph in a text file
with open("graphs.txt", 'a+') as f:
f.write(json.dumps(g))
f.write("\n")
#append the new graph in the all graph list - this list will be the input for the graphExists function
all_graphs.append(g)
if __name__ == '__main__':
generateNgraph(8, 3, 1500)
按照這里的主要 function 及其評論了解整個流程。
Output:
{"1": [2, 7, 5], "2": [1, 7, 5], "7": [1, 2, 6], "5": [1, 3, 2], "4": [6, 3, 8], "6": [4, 7, 8], "3": [4, 5, 8], "8": [6, 3, 4]}
{"1": [8, 7, 2], "8": [1, 2, 6], "7": [1, 4, 5], "2": [1, 6, 8], "6": [2, 3, 8], "4": [7, 3, 5], "3": [4, 5, 6], "5": [4, 3, 7]}
{"1": [8, 7, 5], "8": [1, 3, 2], "7": [1, 5, 6], "5": [1, 4, 7], "2": [3, 4, 8], "3": [2, 6, 8], "4": [6, 2, 5], "6": [4, 3, 7]}
其中每個字典的鍵是一個命名頂點,值是一個表示連接頂點的列表。
這不是一個真正的優化代碼,因為它的復雜性隨着圖的生成而增長。 它將檢查為每次迭代生成的每個圖,因此復雜度為 O(n)。
現在為您提出第一個問題:在給定 n 條邊和給定度數的情況下,我們 output 可以有多少個圖,首先,如果您希望所有邊嚴格連接,則可能沒有解決方案。 其次,在我看來,這是一個非常復雜的數學問題。
這是我到目前為止所發現的,但是為了在代碼中實現它,我讓其他專家來回答這個問題(因為我不知道對不起)。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.