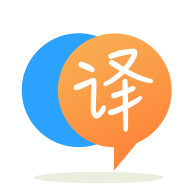
[英]how to get all records from one table and based on that each record fetch all records which associated to the first table records in mvc 5
[英]Datatable C# - How to get detail record from one table and its associated sub record from another table based on ID
如果我單擊任何單個記錄,它會執行兩個操作,我在數據表中有一個學生記錄。
獲取學生詳細信息,它工作正常,如使用 getJson 以綠色圈出的圖像中所述。
我還想獲得選定學生的所有發票及其付款。
注意:發票可以是一張或多張,付款也可以是一張或多張。
問題 - 用紅色圈出
一個。 我在數據表的第一行中沒有可用的數據。
灣。 僅從 Invoice 表中獲取數據,而不從 payment 表中獲取數據。
c。 無法從表中記錄 select。
解決方案/解決方法
作為用戶 select 列表中的任何學生,它應該顯示所有發票及其相應的付款,然后轉到下一張發票及其相關付款。
例子:
學生證:123
發票編號:12345、12347、123479
付款 ID:發票 ID 12345 為 12,12345 為 14,12347 為 17,依此類推。
JSON 代碼:
var oTable1;
oTable1 = $('#invoiceNo').dataTable();
$('#invoiceNo').on('click', 'tr', function ()
{
var stu_id = $(this).find('td').eq(0).text();
var aid = parseInt(stu_id);
//alert(aid);
$.getJSON("/Transaction/showStu_Inv_Pay",
{
id: aid
},
function (data) {
num_rows = data.length;
alert(num_rows);
var myTable = $('#inv_pay').DataTable();
myTable.clear().rows.add(myTable.data).draw();
$(data).each(function (index, item) {
$('#inv_pay tbody').append(
'<tr><td>' + this.InvoiceID +
'</td><td>' + this.InvoiceIDate +
'</td><td>' + this.Invoice_Type_Name +
'</td></tr>'
)
});
});
});
Linq查詢
public IList<Transaction> GetInvByStuID()
{
try
{
DBAPPSEntities _db = new DBAPPSEntities();
this.Invoice_Type_Name = "Invoice";
IList<Transaction> List = (from q in _db.DEL_STU_INV
where q.STU_ID == this.Stu_ID
select new Transaction
{
InvoiceID = q.INVOICE_ID,
InvoiceIDate = q.Inv_Issue_Date,
InvoiceDDate = q.Inv_Due_Date,
Invoice_Month1 = q.Inv_Month_1,
Invoice_Month2 = q.Inv_Month_2,
Invoice_Month3 = q.Inv_Month_3,
Invoice_Note = q.Inv_Note,
Invoice_Adjustment = q.Adjust_Type,
Invoice_Type_Name = this.Invoice_Type_Name,
}).ToList();
return List;
}
catch
{
return null;
}
}
注意:- linq 查詢僅來自發票表,不確定如何與付款表結合,可以更改。
第 101 節
Controller - 設置學生ID
public JsonResult showStu_Inv_Pay(int id)
{
var model = new Transaction { Stu_ID = id }; // student id set to model
var data = model.GetInvByStuID();
return Json(data, JsonRequestBehavior.AllowGet);
}
第 102 條
樣本數據
第 103 節 - 更新 - 1
第 104 節 - 更新 - 2
試試下面的代碼:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ConsoleApplication164
{
class Program
{
static void Main(string[] args)
{
DBAPPSEntities _db = new DBAPPSEntities();
_db.DEL_STU_INV = new List<DEL_STU_INV>() {
new DEL_STU_INV() { INVOICE_ID = 59456, Inv_Issue_Date = new DateTime(20,6,1), Inv_Due_Date = new DateTime(20,6,15), STU_ID = 197, STU_Name = "New_Student1", Amount = 1000},
new DEL_STU_INV() { INVOICE_ID = 59457, Inv_Issue_Date = new DateTime(20,6,1), Inv_Due_Date = new DateTime(20,6,10), STU_ID = 197, STU_Name = "New_Student2", Amount = 1000},
new DEL_STU_INV() { INVOICE_ID = 59458, Inv_Issue_Date = new DateTime(20,6,1), Inv_Due_Date = new DateTime(20,6,10), STU_ID = 103, STU_Name = "New_Student3", Amount = 1000},
new DEL_STU_INV() { INVOICE_ID = 59459, Inv_Issue_Date = new DateTime(20,6,1), Inv_Due_Date = new DateTime(20,6,10), STU_ID = 184, STU_Name = "New_Student4", Amount = 1000},
new DEL_STU_INV() { INVOICE_ID = 59460, Inv_Issue_Date = new DateTime(20,6,1), Inv_Due_Date = new DateTime(20,6,10), STU_ID = 197, STU_Name = "New_Student5", Amount = 1000}
};
_db.PAYMENT = new List<PAYMENT>() {
new PAYMENT() { PAYMENT_ID = 1, PDate = new DateTime(20,6,18), STU_ID = 197, INV_ID = 59456, Paid_Amount = 200, Balance = 800},
new PAYMENT() { PAYMENT_ID = 2, PDate = new DateTime(20,6,17), STU_ID = 934, INV_ID = 59458, Paid_Amount = 500, Balance = 500},
new PAYMENT() { PAYMENT_ID = 3, PDate = new DateTime(20,6,17), STU_ID = 197, INV_ID = 59456, Paid_Amount = 250, Balance = 550},
new PAYMENT() { PAYMENT_ID = 4, PDate = new DateTime(20,6,17), STU_ID = 197, INV_ID = 59457, Paid_Amount = 1000, Balance = 0},
new PAYMENT() { PAYMENT_ID = 5, PDate = new DateTime(20,6,17), STU_ID = 950, INV_ID = 59459, Paid_Amount = 1000, Balance = 0},
new PAYMENT() { PAYMENT_ID = 6, PDate = new DateTime(20,6,17), STU_ID = 197, INV_ID = 59456, Paid_Amount = 500, Balance = 50},
new PAYMENT() { PAYMENT_ID = 7, PDate = new DateTime(20,6,17), STU_ID = 196, INV_ID = 59458, Paid_Amount = 250, Balance = 250},
new PAYMENT() { PAYMENT_ID = 8, PDate = new DateTime(20,6,17), STU_ID = 1060, INV_ID = 59458, Paid_Amount = 250, Balance = 0}
};
int Stu_ID = 197;
var List = (from s in _db.DEL_STU_INV.Where(x => x.STU_ID == Stu_ID)
join p in _db.PAYMENT on s.INVOICE_ID equals p.INV_ID into ps
from z in ps.DefaultIfEmpty()
select new { s = s, p = (z == null)? null : z }
)
.GroupBy(x => x.s.INVOICE_ID)
.Select(x =>
new
{
invoice = new Result() { type = "Invoice", id = x.Key, date = x.First().s.Inv_Issue_Date, amount = x.First().s.Amount },
payments = x.Select(y => (y.p == null)? null : new Result() { type = "Payment", id = y.p.PAYMENT_ID, date = y.p.PDate, amount = y.p.Paid_Amount })
}).Select(y => (y.payments.Count() == 1) ? new List<Result>() { y.invoice } : (new List<Result>() { y.invoice }).Concat(y.payments).ToList())
.ToList();
}
}
public class DBAPPSEntities
{
public List<DEL_STU_INV> DEL_STU_INV { get;set;}
public List<PAYMENT> PAYMENT { get; set; }
}
public class DEL_STU_INV
{
public int STU_ID { get; set; }
public string STU_Name{ get;set;}
public int INVOICE_ID { get; set; }
public int Amount { get; set;}
public DateTime Inv_Issue_Date { get; set; }
public DateTime Inv_Due_Date { get; set; }
public string Inv_Month_1 { get; set; }
public string Inv_Month_2 {get; set; }
public string Inv_Month_3 { get; set; }
public string Inv_Note { get; set; }
public string Adjust_Type { get; set; }
}
public class PAYMENT
{
public int PAYMENT_ID { get; set; }
public DateTime PDate { get; set; }
public int STU_ID { get; set; }
public int INV_ID { get; set; }
public int Paid_Amount { get; set; }
public int Balance { get; set; }
}
public class Transaction
{
public int InvoiceID { get;set;}
public DateTime InvoiceIDate { get;set;}
public DateTime InvoiceDDate { get;set;}
public string Invoice_Month1 { get;set;}
public string Invoice_Month2 { get;set;}
public string Invoice_Month3 { get;set;}
public string Invoice_Note { get;set;}
public string Invoice_Adjustment { get; set; }
public string Invoice_Type_Name { get; set; }
}
public class Result
{
public string type { get; set; }
public int id { get; set; }
public DateTime date { get; set; }
public int amount { get; set; }
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.