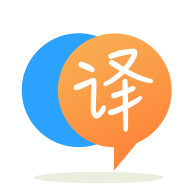
[英]How to overlay contour plot on 3-D surface plot with matplotlib or plotly?
[英]Plotly: Change a contour plot into a 3-d surface
我想將下面用於 2-D 高斯分布的等高線圖更改為 3-D 曲面圖。 我在下面有我的代碼和情節。 不知道如何將其更改為 3-D 版本。 有沒有人有任何提示? 注意:我實際上有四個等高線圖,我想將它們全部更改為 3-D 曲面圖。
import pandas as pd
import math
import cmath
import time
import random
import io
import itertools
import requests
import statistics
import numpy as np
from scipy.stats import multivariate_normal
import plotly.express as px
from plotly.subplots import make_subplots
import warnings
from IPython.display import HTML, display
import tabulate
import plotly.graph_objects as go
def norm_pdf_multivariate(x, mu, sigma):
size = len(x)
det = np.linalg.det(sigma)
norm_const = 1.0/ ( math.pow((2*math.pi),float(size)/2) * math.pow(det,1.0/2) )
x_mu = np.matrix(x - mu)
inv = np.linalg.inv(sigma)
result = math.pow(math.e, -0.5 * (x_mu * inv * x_mu.T))
return norm_const * result
x = np.linspace(-6,6,100)
y = np.linspace(-6,6,100)
X, Y = np.meshgrid(x, y)
X_ = X.tolist()
X_ = [y for x in X_ for y in x]
Y_ = Y.tolist()
Y_ = [y for x in Y_ for y in x]
Samples = [[X_[i],Y_[i]] for i in range(len(X_))]
Cov_1 = [[1,0],[0,1]]
Means_1 = [0,0]
Cov_2 = [[1,0],[0,5]]
Means_2 = [0,0]
Cov_3 = [[5,1],[1,1]]
Means_3 = [0,0]
Cov_4 = [[5,-1],[-1,1]]
Means_4 = [0,0]
Z_1 = []
Z_2 = []
Z_3 = []
Z_4 = []
for i in range(len(Samples)):
Z_1.append(norm_pdf_multivariate(x = np.array(Samples[i]),mu = Means_1,sigma = Cov_1))
Z_2.append(norm_pdf_multivariate(x = np.array(Samples[i]),mu = Means_2,sigma = Cov_2))
Z_3.append(norm_pdf_multivariate(x = np.array(Samples[i]),mu = Means_3,sigma = Cov_3))
Z_4.append(norm_pdf_multivariate(x = np.array(Samples[i]),mu = Means_4,sigma = Cov_4))
colorscale = [[0, 'gold'], [0.5, 'mediumturquoise'], [1, 'lightsalmon']]
fig = make_subplots(rows = 4,cols = 1)
fig.append_trace(go.Contour(z=Z_1,
x=X_,
y=Y_,
contours_coloring='lines',
colorscale = colorscale,
line_width=2,
showscale=False),row = 1,col = 1)
fig.append_trace(go.Contour(z=Z_2,
x=X_,
y=Y_,
contours_coloring='lines',
colorscale = colorscale,
line_width=2,
showscale=False),row = 2,col = 1)
fig.append_trace(go.Contour(z=Z_3,
x=X_,
y=Y_,
contours_coloring='lines',
colorscale = colorscale,
line_width=2,
showscale=False),row = 3,col = 1)
fig.append_trace(go.Contour(z=Z_4,
x=X_,
y=Y_,
contours_coloring='lines',
colorscale = colorscale,
line_width=2,
showscale=False),row = 4,col = 1)
fig.update_layout(
width = 350,
height = 1200
)
fig.update_layout(template = "plotly_white")
fig.show()
import math
import numpy as np
from plotly.subplots import make_subplots
import plotly.graph_objects as go
def norm_pdf_multivariate(x, mu, sigma):
size = len(x)
det = np.linalg.det(sigma)
norm_const = 1.0 / (math.pow((2 * math.pi),float(size)/2) * math.pow(det, 1.0/2))
x_mu = np.matrix(x - mu)
inv = np.linalg.inv(sigma)
result = math.pow(math.e, -0.5 * (x_mu * inv * x_mu.T))
return norm_const * result
x = np.linspace(-6, 6, 100)
y = np.linspace(-6, 6, 100)
Cov_1 = [[1,0],[0,1]]
Means_1 = [0,0]
Cov_2 = [[1,0],[0,5]]
Means_2 = [0,0]
Cov_3 = [[5,1],[1,1]]
Means_3 = [0,0]
Cov_4 = [[5,-1],[-1,1]]
Means_4 = [0,0]
Z1 = np.zeros((len(x), len(y)))
Z2 = np.zeros((len(x), len(y)))
Z3 = np.zeros((len(x), len(y)))
Z4 = np.zeros((len(x), len(y)))
for i in range(len(x)):
for j in range(len(y)):
Z1[i, j] = norm_pdf_multivariate(np.array([x[i], y[j]]), Means_1, Cov_1)
Z2[i, j] = norm_pdf_multivariate(np.array([x[i], y[j]]), Means_2, Cov_2)
Z3[i, j] = norm_pdf_multivariate(np.array([x[i], y[j]]), Means_3, Cov_3)
Z4[i, j] = norm_pdf_multivariate(np.array([x[i], y[j]]), Means_4, Cov_4)
colorscale = [[0, 'gold'], [0.5, 'mediumturquoise'], [1, 'lightsalmon']]
fig = make_subplots(rows=2, cols=2, vertical_spacing=0, horizontal_spacing=0,
specs=[[{'type': 'scene'}, {'type': 'scene'}], [{'type': 'scene'}, {'type': 'scene'}]])
fig.append_trace(go.Surface(z=Z1, x=x, y=y, colorscale=colorscale, showscale=False), row=1, col=1)
fig.append_trace(go.Surface(z=Z2, x=x, y=y, colorscale=colorscale, showscale=False), row=1, col=2)
fig.append_trace(go.Surface(z=Z3, x=x, y=y, colorscale=colorscale, showscale=False), row=2, col=1)
fig.append_trace(go.Surface(z=Z4, x=x, y=y, colorscale=colorscale, showscale=False), row=2, col=2)
fig.update_layout(template='plotly_white', margin=dict(t=0, b=0, l=0, r=0, pad=0), font=dict(size=10))
fig.show()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.