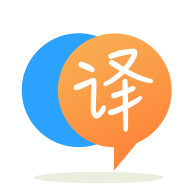
[英]Unity: Game works in editor but doesn't when built. Does editor code differ from build?
[英]Unity code works in the editor but does not work on Build C#
首先,我知道還有其他人有同樣的問題,不,他們的解決方案對我的情況沒有幫助。
我正在為大學做最后一個項目,他們強迫我們使用 Perforce Helix Client(順便說一下,這是純粹的地獄)進行版本控制。
我和我的團隊日夜工作,在編輯器中解決問題。 然后我們終於到了可以測試我們的構建的部分。 構建在我們的兩端運行良好。 (是的,我們在 perforce 中刪除了舊的構建文件並獲得了當前的構建文件)
然后,災難發生了,當我們的教授測試我們的游戲時,我們的動作腳本不起作用,他又給了我們一次機會,但說因此我們的成績不能高於 70。
無論如何,我不是制作這個腳本的人,但我想知道這里是否有任何東西會導致腳本在構建中不起作用。
對於那些不關心背景故事的人-
問題:玩家不會進入我們的構建和控制台垃圾郵件 nullreference。
預期結果:角色四處走動。
我們嘗試過的:刪除過去的構建並重新下載當前構建並檢查檢查器中的所有內容
移動腳本:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
private Vector3 moveInput;
private Vector3 moveVelocity;
private Vector3 mousePosition;
private Camera mainCamera;
// Start is called before the first frame update
void Start()
{
rb = GetComponent<Rigidbody>();
mainCamera = FindObjectOfType<Camera>();
}
// Update is called once per frame
void Update()
{
float distance = Vector3.Distance(transform.position, mousePosition);
if(distance > 2)
{
moveInput = new Vector3(Input.GetAxisRaw("Horizontal") * 40 * Time.smoothDeltaTime, 0f, Input.GetAxisRaw("Vertical") * 40 * Time.smoothDeltaTime);
moveVelocity = moveInput * moveSpeed * Time.smoothDeltaTime;
}
mousePosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit, 2000))
{
transform.LookAt(new Vector3(hit.point.x, transform.position.y, hit.point.z));
}
}
void FixedUpdate()
{
rb.velocity = moveVelocity;
}
}
積分守護者:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PointsKeeper : MonoBehaviour
{
public int points = 0;
public Text pointText;
public GameObject toExpensiveText;
public float expensiveTimer = 1.0f;
public GameObject healthPage;
public GameObject weaponPage;
public GameObject modPage;
public GameObject lifeBlocker;
public GameObject lifeSector;
public GameObject lifeDropChanceSector;
public GameObject shieldBlocker;
public GameObject shieldRegensector;
public GameObject healthBlocker;
public GameObject healthcapacitysector;
private PlayerHealth playerHealth;
public Sprite fullCell;
public Sprite emptyCell;
//Health Page
private int healprice = 100;
public Text healpriceText;
public GameObject healButton;
public int maxHealthLVL = 0;
private int maxHealthMAXLVL = 5;
private int maxHealthprice = 100;
public Text maxhealthpricetext;
public GameObject maxhealthButton;
public Image[] maxhealthCells;
public int healDropChanceLVL = 0;
private int healdropchanceprice = 100;
public int shieldcapacityLVL = 0;
private int sheildcapacityPrice = 100;
public int shieldRegenRateLVL = 0;
public int lifeDropChanceLVL = 0;
public int lifeCapacityLVL = 0;
private int lifePrice;
//Weapon Page
//Mod Page
// Start is called before the first frame update
void Start()
{
points = 0;
playerHealth = FindObjectOfType<PlayerHealth>();
toExpensiveText.SetActive(false);
}
// Update is called once per frame
void Update()
{
pointText.text = points.ToString();
healpriceText.text = healprice.ToString();
maxhealthpricetext.text = maxHealthprice.ToString();
maxHealthCellControl();
if (lifeCapacityLVL >= 1)
{
lifeBlocker.SetActive(false);
lifeSector.SetActive(true);
lifeDropChanceSector.SetActive(true);
}
if (shieldcapacityLVL >= 1)
{
shieldBlocker.SetActive(false);
shieldRegensector.SetActive(true);
}
if (playerHealth.playerHP == playerHealth.maxheart)
{
healthBlocker.SetActive(false);
healthcapacitysector.SetActive(true);
}
else
{
healthBlocker.SetActive(true);
healthcapacitysector.SetActive(false);
}
if(playerHealth.playerHP == playerHealth.maxheart)
{
healButton.SetActive(false);
}
else
{
healButton.SetActive(true);
}
if (Input.GetKeyDown(KeyCode.P))
{
points = points + 100000;
}
}
public void pointsGained(int pointsup)
{
points = points + pointsup;
}
public void healthPageOn()
{
healthPage.SetActive(true);
modPage.SetActive(false);
weaponPage.SetActive(false);
}
public void weaponPageOn()
{
healthPage.SetActive(false);
modPage.SetActive(false);
weaponPage.SetActive(true);
}
public void modPageOn()
{
healthPage.SetActive(false);
modPage.SetActive(true);
weaponPage.SetActive(false);
}
public void toExpensive()
{
}
public void buyHeal()
{
if(points >= healprice)
{
points = points - healprice;
healprice = healprice + 100;
playerHealth.playerHP = playerHealth.playerHP + 1;
}
else
{
toExpensive();
}
}
public void buyMaxHealth()
{
if (points >= maxHealthprice && maxHealthLVL < 5)
{
maxHealthLVL = maxHealthLVL + 1;
points = points - maxHealthprice;
playerHealth.maxheart = playerHealth.maxheart + 1;
}
else
{
toExpensive();
}
if (maxHealthLVL == 1)
{
maxHealthprice = 1000;
}
else if (maxHealthLVL == 2)
{
maxHealthprice = 2000;
}
else if (maxHealthLVL == 3)
{
maxHealthprice = 4000;
}
else if (maxHealthLVL == 4)
{
maxHealthprice = 10000;
}
else if (maxHealthLVL == 5)
{
maxhealthButton.SetActive(false);
maxhealthpricetext.gameObject.SetActive(false);
}
}
public void maxHealthCellControl()
{
for (int i = 0; i < maxhealthCells.Length; i++)
{
if (i < maxHealthLVL)
{
maxhealthCells[i].sprite = fullCell;
}
else
{
maxhealthCells[i].sprite = emptyCell;
}
if (i < maxHealthMAXLVL)
{
maxhealthCells[i].enabled = true;
}
else
{
maxhealthCells[i].enabled = false;
}
}
}
}
另外,關於如何修復空引用的任何想法都會很好。
抱歉,如果問題不夠具體或者我沒有提供足夠的信息。 我仍然很震驚,不經常使用這個網站。
試試這個,擺脫異常:
public void maxHealthCellControl()
{
if (maxhealthCells == null) return;
for (int i = 0; i < maxhealthCells.Length; i++)
{
if (i < maxHealthLVL)
{
maxhealthCells[i].sprite = fullCell;
}
else
{
您根據默認為0
moveSpeed
計算速度。
如果您在編輯器(檢查器)中設置此公共序列化字段,您的移動將起作用,但序列化值可能不會存儲在構建中(取決於您對播放器的初始化)。
我們最終重做了整個運動系統。 感謝您的幫助!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.