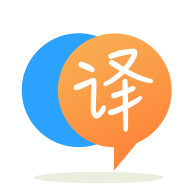
[英]System.FormatException: Input string was not in a correct format. c#
[英]C# Excel empty rows in combobox (causing error “System.FormatException:Input string was not in a correct format.”)
首先,對不起我的英語不好,但我是 C# 的初學者,他正在嘗試制作一個簡單的 Excel 應用程序(學校工作),您可以在其中編輯 ZC1D81AF5835844B4E9D936910DED8FDC 的玩家列表中的新玩家列表和添加新玩家列表。 編輯工作完美,但我無法在列表中添加新玩家。 當我查看應用程序的 combobox 時,有很多空單元格,可能正因為如此,添加新播放器不起作用。
嘗試添加新播放器時,出現以下錯誤:
System.FormatException:Input string was not in a correct format.
當我嘗試調試問題時,它表明問題出在這一行:
int nextID = Convert.ToInt32(lastID) + 1; // Lasketaan seuraavan ID:n arvo
已經謝謝了,如果您需要更多信息來幫助我,請不要猶豫!
這是實際程序的代碼。
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Office.Interop.Excel;
namespace Luokat.Persons
{
public class Persons : ExcelInstance
{
//variables internal use
private string id;
private string lastname;
private string firstname;
private string email;
private string postalcode;
private string city;
private string phone;
private ArrayList arInfo = new ArrayList();
private string ok = "0";
private string found = "0";
private string error = "0";
//properties external use
public string Id
{
get
{
return id;
}
set
{
id = value;
}
}
public string Lastname
{
get
{
return lastname;
}
set
{
lastname = value;
}
}
public string Firstname
{
get
{
return firstname;
}
set
{
firstname = value;
}
}
public string Email
{
get
{
return email;
}
set
{
email = value;
}
}
public string Postalcode
{
get
{
return postalcode;
}
set
{
postalcode = value;
}
}
public string City
{
get
{
return city;
}
set
{
city = value;
}
}
public string Phone
{
get
{
return phone;
}
set
{
phone = value;
}
}
public ArrayList ArInfo
{
get
{
return arInfo;
}
set
{
arInfo = value;
}
}
public string Ok
{
get
{
return ok;
}
set
{
ok = value;
}
}
public string Found
{
get
{
return found;
}
set
{
found = value;
}
}
public string Error
{
get
{
return error;
}
set
{
error = value;
}
}
//Methods
public void searchPersons()
{
try
{
int totalRows = xlRange.Rows.Count;
int totalColumns = xlRange.Columns.Count;
for (int rowCount = 2; rowCount <= totalRows; rowCount++)
{
this.id = xlRange.Cells[rowCount, 1].Text;
this.lastname = xlRange.Cells[rowCount, 2].Text;
this.firstname = xlRange.Cells[rowCount, 3].Text;
ArInfo.Add(this.Id + " " + this.Lastname + " " + this.Firstname);
}
//xlWorkBook.Close(true);
//xlApp.Quit();
}
catch (Exception e)
{
this.error = e.ToString();
}
}
public void searchPerson(string sID)
{
/*Microsoft.Office.Interop.Excel.Application xlApp = new Microsoft.Office.Interop.Excel.Application();
Microsoft.Office.Interop.Excel.Workbook xlWorkBook = xlApp.Workbooks.Open(FilePath);
Microsoft.Office.Interop.Excel.Worksheet xlWorkSheet = (Microsoft.Office.Interop.Excel.Worksheet)xlWorkBook.Worksheets.get_Item(1);
Microsoft.Office.Interop.Excel.Range xlRange = xlWorkSheet.UsedRange;*/
int totalRows = xlRange.Rows.Count;
int totalColumns = xlRange.Columns.Count;
for (int rowCount = 2; rowCount <= totalRows; rowCount++)
{
this.id = xlRange.Cells[rowCount, 1].Text;
if (this.id == sID)
{
this.Email = xlRange.Cells[rowCount, 4].Text;
this.Postalcode = xlRange.Cells[rowCount, 5].Text;
this.City = xlRange.Cells[rowCount, 6].Text;
this.Phone = xlRange.Cells[rowCount, 7].Text;
}
}
}
public void editPerson(string sID, string sLastname, string sFirstname, string sEmail, string sPostalcode, string sCity, string sPhone)
{
int totalRows = xlRange.Rows.Count;
int totalColumns = xlRange.Columns.Count;
for (int rowCount = 2; rowCount <= totalRows; rowCount++)
{
this.id = xlRange.Cells[rowCount, 1].Text;
if (this.id == sID)
{
xlRange.Cells[rowCount, 2] = sLastname;
xlRange.Cells[rowCount, 3] = sFirstname;
xlRange.Cells[rowCount, 4] = sEmail;
xlRange.Cells[rowCount, 5] = sPostalcode;
xlRange.Cells[rowCount, 6] = sCity;
xlRange.Cells[rowCount, 7] = sPhone;
this.ok = "1";
break;
}
}
}
public void newPerson(string sLastname, string sFirstname, string sEmail, string sPostalcode, string sCity, string sPhone)
{
int totalRows = xlRange.Rows.Count;
int totalColumns = xlRange.Columns.Count;
int nextRow = totalRows + 1; // Lisätään rivien arvoa yhdellä
string lastID = xlRange.Cells[totalRows, 1].Text; // Haetaan viimeinen ID
int nextID = Convert.ToInt32(lastID) + 1; // Lasketaan seuraavan ID:n arvo
// Tarkistetaan sähköposti
for (int rowCount = 2; rowCount <= totalRows; rowCount++)
{
this.email = xlRange.Cells[rowCount, 4].Text;
if (this.email == sEmail)
{
this.found = "1"; // Jos löytyi, annetaan muuttujalle arvo 1
break;
}
}
if (found == "1") // Jos löytyi suljetaan instanssit ja poistutaan
{
xlWorkBook.Close(true);
xlApp.Quit();
return;
}
// Jos sähköposia ei löydy, lisätään uusi henkilö tietoineen
xlRange.Cells[nextRow, 1] = nextID;
xlRange.Cells[nextRow, 2] = sLastname;
xlRange.Cells[nextRow, 3] = sFirstname;
xlRange.Cells[nextRow, 4] = sEmail;
xlRange.Cells[nextRow, 5] = sPostalcode;
xlRange.Cells[nextRow, 6] = sCity;
xlRange.Cells[nextRow, 7] = sPhone;
this.ok = "1";
}
public void removePerson(string sID)
{
int totalRows = xlRange.Rows.Count;
for (int rowCount = 2; rowCount <= totalRows; rowCount++)
{
this.id = xlRange.Cells[rowCount, 1].Text;
if (this.id == sID)
{
xlWorkSheet.Rows[rowCount].EntireRow.Delete();
this.ok = "1";
break;
}
}
}
public void closeExcel()
{
xlWorkBook.Close(true); //true = autosave
xlApp.Quit();
}
}
}
lastID 可能是也可能不是 int。
改用 Int32.TryParse(value, out number)。 這將解決你的問題。
int lastIDValue;
Int32.TryParse(lastID, out lastIDValue)
嘗試使用int.TryParse
if(int.TryParse(yourStr, out yourInt) == true)
{
//if success
}
else
{
//if fail
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.