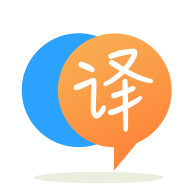
[英]How do i update an application after pusblishing it in visual studio
[英]How can I get the application to pause and update a bar chart in Visual Studio?
我試圖在它的排序過程中畫出一個快速排序算法。 這是快速排序的代碼。
public void swap(int[] arr, int i, int j)
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
/* This function takes last element as a pivot, places
the pivot element at its correct position in sorted
array, and places all smaller (smaller than pivot)
to left of pivot and all greater elements to right
of pivot */
}
public int partition(int[] arr, int low, int high)
{
// pivot
int pivot = arr[high];
// Index of smaller element and
// indicates the right position
// of pivot found so far
int i = (low - 1);
for (int j = low; j <= high - 1; j++)
{
// If current element is smaller
// than the pivot
if (arr[j] < pivot)
{
// Increment index of
// smaller element
i++;
swap(arr, i, j);
}
}
swap(arr, i + 1, high);
return (i + 1);
}
public void quickSort(int[] arr, int low, int high)
{
if (low < high)
{
// pi is partitioning index, arr[p]
// is now at right place
int pi = partition(arr, low, high);
// Separately sort elements before
// partition and after partition
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
updateBarChart(arr);
}
}
updateBarChart(arr)
獲取一個數組並將其繪制在條形圖控件上。 代碼如下:
public void updateBarChart(int[] selectedArray)
{
chart1.Series["Data"].Points.Clear();
int placement = 0;
foreach (int point in selectedArray)
{
this.chart1.Series["Data"].Points.AddXY(placement, point);
placement += 1;
}
}
我能夠進行插入排序以逐步繪制條形圖的方法是使用Task.Delay(some value)
,其中some value
是基於軌跡欄的 integer 。 它會暫停一段指定的時間,然后繪制條形圖,讓用戶可以在他們面前查看排序過程。 此方法適用於插入排序,我將在本段下方列出代碼,但是當我嘗試使用我的快速排序函數時,它不起作用。 數組將被完全排序,然后在已經排序后繪制。 用戶無法看到排序的發生,這違背了排序可視化器的目的。 我假設問題源於快速排序排序過程涉及遞歸這一事實,這會使Task.Delay
調用偏離軌道。 這是我的假設,但我真的不知道。
下面是我的插入排序 function。
public async void InsertionSort(int[] intArray)
{
int i = 1;
int j = 1;
int placeHolder = 1;
while (i < intArray.Length && stopStatus == false)
{
j = i;
while (j > 0 && intArray[j - 1] > intArray[j])
{
placeHolder = intArray[j];
intArray[j] = intArray[j - 1];
intArray[j - 1] = placeHolder;
j--;
updateBarChart(intArray);
await Task.Delay(sortSpeedBar.Value);
}
i++;
}
我試過使用計時器控件,我讓計時器基本上運行一秒鍾,然后嘗試繪制條形圖,但最終,它做了與 task.Delay 相同的事情:完全排序,然后繪制最終排序值。 另外,我知道為了快速排序以逐步繪制,我已將updateBarChart
方法放在適當的位置以使更新准確。 我的代碼沒有在正確的位置。 我只是想在繼續之前嘗試使用Task.Delay
解決線程/遞歸問題。 有沒有人有任何想法。
最后,僅作為示例,運行快速排序的驅動程序代碼。 這是在 .netframework 4.7.2 上運行的
Class Program
{
static void Main()
{
int[] arr = {7, 4, 9, 1, 12, 5, 0, 6, 4};
int arrayLength = arr.Length - 1;
quickSort(arr, 0, arrayLength);
}
}
如果你不介意阻塞你的主線程,你可以試試 Thread.Sleep(milliseconds):
public void quickSort(int[] arr, int low, int high)
{
....
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
updateBarChart(arr);
Thread.Sleep(sortSpeedBar.Value);
}
}
如果您需要它是異步的,您還可以在新的分離線程上調用 function。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.